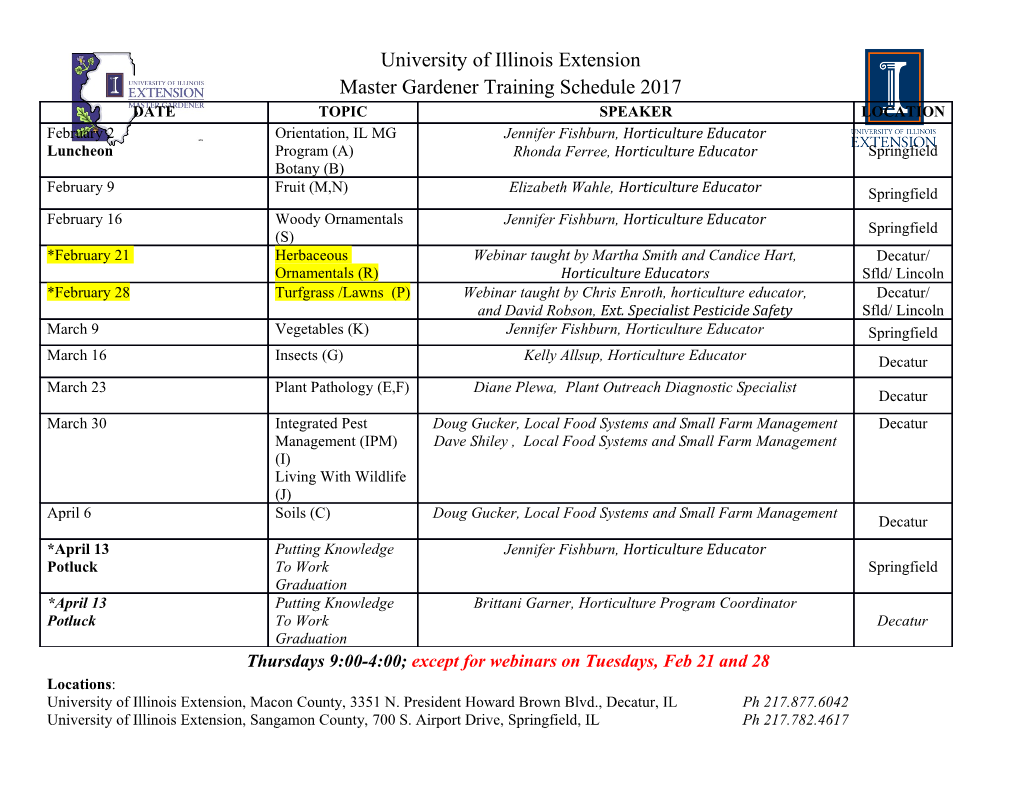
Kernel Programming Guide 2006-10-03 registered in the United States and other Apple Computer, Inc. countries. © 2002, 2006 Apple Computer, Inc. DEC is a trademark of Digital Equipment All rights reserved. Corporation. No part of this publication may be Java and all Java-based trademarks are reproduced, stored in a retrieval system, or trademarks or registered trademarks of Sun transmitted, in any form or by any means, Microsystems, Inc. in the U.S. and other mechanical, electronic, photocopying, countries. recording, or otherwise, without prior OpenGL is a registered trademark of Silicon written permission of Apple Computer, Inc., Graphics, Inc. with the following exceptions: Any person PowerPC and and the PowerPC logo are is hereby authorized to store documentation trademarks of International Business on a single computer for personal use only Machines Corporation, used under license and to print copies of documentation for therefrom. personal use provided that the documentation contains Apple’s copyright SPEC is a registered trademark of the notice. Standard Performance Evaluation Corporation (SPEC). The Apple logo is a trademark of Apple Computer, Inc. Simultaneously published in the United States and Canada. Use of the “keyboard” Apple logo (Option-Shift-K) for commercial purposes Even though Apple has reviewed this document, APPLE MAKES NO WARRANTY OR without the prior written consent of Apple REPRESENTATION, EITHER EXPRESS OR may constitute trademark infringement and IMPLIED, WITH RESPECT TO THIS DOCUMENT, ITS QUALITY, ACCURACY, unfair competition in violation of federal MERCHANTABILITY, OR FITNESS FOR A and state laws. PARTICULAR PURPOSE. AS A RESULT, THIS DOCUMENT IS PROVIDED “AS IS,” AND No licenses, express or implied, are granted YOU, THE READER, ARE ASSUMING THE ENTIRE RISK AS TO ITS QUALITY AND with respect to any of the technology ACCURACY. described in this document. Apple retains IN NO EVENT WILL APPLE BE LIABLE FOR all intellectual property rights associated DIRECT, INDIRECT, SPECIAL, INCIDENTAL, with the technology described in this OR CONSEQUENTIAL DAMAGES RESULTING FROM ANY DEFECT OR document. This document is intended to INACCURACY IN THIS DOCUMENT, even if assist application developers to develop advised of the possibility of such damages. applications only for Apple-labeled or THE WARRANTY AND REMEDIES SET Apple-licensed computers. FORTH ABOVE ARE EXCLUSIVE AND IN LIEU OF ALL OTHERS, ORAL OR WRITTEN, Every effort has been made to ensure that EXPRESS OR IMPLIED. No Apple dealer, agent, or employee is authorized to make any the information in this document is modification, extension, or addition to this accurate. Apple is not responsible for warranty. typographical errors. Some states do not allow the exclusion or limitation of implied warranties or liability for Apple Computer, Inc. incidental or consequential damages, so the above limitation or exclusion may not apply to 1 Infinite Loop you. This warranty gives you specific legal Cupertino, CA 95014 rights, and you may also have other rights which 408-996-1010 vary from state to state. Apple, the Apple logo, AppleTalk, Carbon, Cocoa, FireWire, Keychain, Logic, Mac, Mac OS, Macintosh, Pages, Panther, Power Mac, Power Macintosh, Quartz, QuickTime, and Xcode are trademarks of Apple Computer, Inc., registered in the United States and other countries. eMac and Finder are trademarks of Apple Computer, Inc. NeXT, Objective-C, and OPENSTEP are trademarks of NeXT Software, Inc., Contents Chapter 1 About This Document 11 Who Should Read This Document 11 Road Map 11 Other Apple Publications 13 Mach API Reference 13 Information on the Web 13 Chapter 2 Keep Out 15 Why You Should Avoid Programming in the Kernel 15 Chapter 3 Kernel Architecture Overview 17 Darwin 18 Architecture 19 Mach 20 BSD 20 I/O Kit 22 Kernel Extensions 22 Chapter 4 Security Considerations 23 Security Implications of Paging 24 Buffer Overflows and Invalid Input 25 User Credentials 25 Basic User Credentials 26 Access Control Lists 27 Remote Authentication 27 One-Time Pads 27 Time-based authentication 28 Temporary Files 29 /dev/mem and /dev/kmem 29 Key-based Authentication and Encryption 29 Public Key Weaknesses 30 Using Public Keys for Message Exchange 31 Using Public Keys for Identity Verification 32 Using Public Keys for Data Integrity Checking 32 3 2006-10-03 | © 2002, 2006 Apple Computer, Inc. All Rights Reserved. CONTENTS Encryption Summary 32 Console Debugging 33 Code Passing 33 Chapter 5 Performance Considerations 35 Interrupt Latency 35 Locking Bottlenecks 36 Working With Highly Contended Locks 36 Reducing Contention by Decreasing Granularity 37 Code Profiling 37 Using Counters for Code Profiling 38 Lock Profiling 38 Chapter 6 Kernel Programming Style 41 C++ Naming Conventions 41 Basic Conventions 41 Additional Guidelines 42 Standard C Naming Conventions 43 Commonly Used Functions 44 Performance and Stability Tips 45 Performance and Stability Tips 45 Stability Tips 47 Style Summary 47 Chapter 7 Mach Overview 49 Mach Kernel Abstractions 49 Tasks and Threads 50 Ports, Port Rights, Port Sets, and Port Namespaces 51 Memory Management 52 Interprocess Communication (IPC) 53 IPC Transactions and Event Dispatching 54 Message Queues 54 Semaphores 55 Notifications 55 Locks 55 Remote Procedure Call (RPC) Objects 55 Time Management 55 Chapter 8 Memory and Virtual Memory 57 Mac OS X VM Overview 57 Memory Maps Explained 59 Named Entries 60 4 2006-10-03 | © 2002, 2006 Apple Computer, Inc. All Rights Reserved. CONTENTS Universal Page Lists (UPLs) 60 Using Mach Memory Maps 62 Other VM and VM-Related Subsystems 63 Pagers 63 Working Set Detection Subsystem 64 VM Shared Memory Server Subsystem 64 Address Spaces 65 Background Info on PCI Address Translation 65 IOMemoryDescriptor Changes 66 VM System and pmap Changes: 66 Kernel Dependency Changes 67 Summary 67 Allocating Memory in the Kernel 67 Allocating Memory Using Mach Routines 67 Allocating Memory From the I/O Kit 68 Chapter 9 Mach Scheduling and Thread Interfaces 71 Overview of Scheduling 71 Why Did My Thread Priority Change? 72 Using Mach Scheduling From User Applications 73 Using the pthreads API to Influence Scheduling 73 Using the Mach Thread API to Influence Scheduling 74 Using the Mach Task API to Influence Scheduling 76 Kernel Thread APIs 77 Creating and Destroying Kernel Threads 77 SPL and Friends 78 Wait Queues and Wait Primitives 78 Chapter 10 Bootstrap Contexts 81 How Contexts Affect Users 82 How Contexts Affect Developers 83 Chapter 11 I/O Kit Overview 85 Redesigning the I/O Model 85 I/O Kit Architecture 87 Families 87 Drivers 87 Nubs 88 Connection Example 88 For More Information 91 5 2006-10-03 | © 2002, 2006 Apple Computer, Inc. All Rights Reserved. CONTENTS Chapter 12 BSD Overview 93 BSD Facilities 94 Differences between Mac OS X and BSD 95 For Further Reading 96 Chapter 13 File Systems Overview 97 Working With the File System 97 VFS Transition 98 Chapter 14 Network Architecture 99 Chapter 15 Boundary Crossings 101 Security Considerations 102 Choosing a Boundary Crossing Method 102 Kernel Subsystems 103 Bandwidth and Latency 103 Mach Messaging and Mach Interprocess Communication (IPC) 104 Using Well-Defined Ports 104 Remote Procedure Calls (RPC) 105 Calling RPC From User Applications 107 BSD syscall API 107 BSD ioctl API 107 BSD sysctl API 108 General Information on Adding a sysctl 108 Adding a sysctl Procedure Call 109 Registering a New Top Level sysctl 111 Adding a Simple sysctl 111 Calling a sysctl From User Space 112 Memory Mapping and Block Copying 114 Summary 115 Chapter 16 Synchronization Primitives 117 Semaphores 117 Condition Variables 119 Locks 119 Spinlocks 119 Mutexes 121 Read-Write Locks 122 Spin/Sleep Locks 124 Using Lock Functions 125 6 2006-10-03 | © 2002, 2006 Apple Computer, Inc. All Rights Reserved. CONTENTS Chapter 17 Miscellaneous Kernel Services 127 Using Kernel Time Abstractions 127 Obtaining Time Information 127 Event and Timer Waits 128 Handling Version Dependencies 130 Boot Option Handling 131 Queues 132 Installing Shutdown Hooks 132 Chapter 18 Kernel Extension Overview 133 Implementation of a Kernel Extension (KEXT) 134 Kernel Extension Dependencies 134 Building and Testing Your Extension 135 Debugging Your KEXT 135 Installed KEXTs 136 Chapter 19 Building and Debugging Kernels 139 Adding New Files or Modules 139 Modifying the Configuration Files 139 Modifying the Source Code Files 141 Building Your First Kernel 141 Building an Alternate Kernel Configuration 143 When Things Go Wrong: Debugging the Kernel 143 Setting Debug Flags in Open Firmware 144 Avoiding Watchdog Timer Problems 145 Choosing a Debugger 146 Using gdb for Kernel Debugging 146 Using ddb for Kernel Debugging 149 Document Revision History 155 Bibliography Bibliography 157 Apple Mac OS X Publications 157 General UNIX and Open Source Resources 157 BSD and UNIX Internals 158 Mach 158 Networking 160 Operating Systems 160 POSIX 161 Programming 161 Websites and Online Resources 161 7 2006-10-03 | © 2002, 2006 Apple Computer, Inc. All Rights Reserved. CONTENTS Security and Cryptography 162 Glossary 163 Index 173 8 2006-10-03 | © 2002, 2006 Apple Computer, Inc. All Rights Reserved. Figures, Tables, and Listings Chapter 3 Kernel Architecture Overview 17 Figure 3-1 Mac OS X architecture 17 Figure 3-2 Darwin and Mac OS X 18 Figure 3-3 Mac OS X kernel architecture 19 Chapter 6 Kernel Programming Style 41 Table 6-1 Commonly used C functions 44 Chapter 9 Mach Scheduling and Thread Interfaces 71 Table 9-1 Thread priority bands 71 Table 9-2 Thread policies 74 Table 9-3 Task roles 76 Chapter 11 I/O Kit Overview 85 Figure 11-1 I/O Kit architecture 89 Chapter 16 Synchronization Primitives 117 Listing 16-1 Allocating lock attributes and groups (lifted liberally from kern_time.c) 125 Chapter 19 Building and Debugging Kernels 139 Table 19-1 Debugging flags 145 Table 19-2 Switch options in ddb 151 9 2006-10-03 | © 2002, 2006 Apple Computer, Inc.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages182 Page
-
File Size-