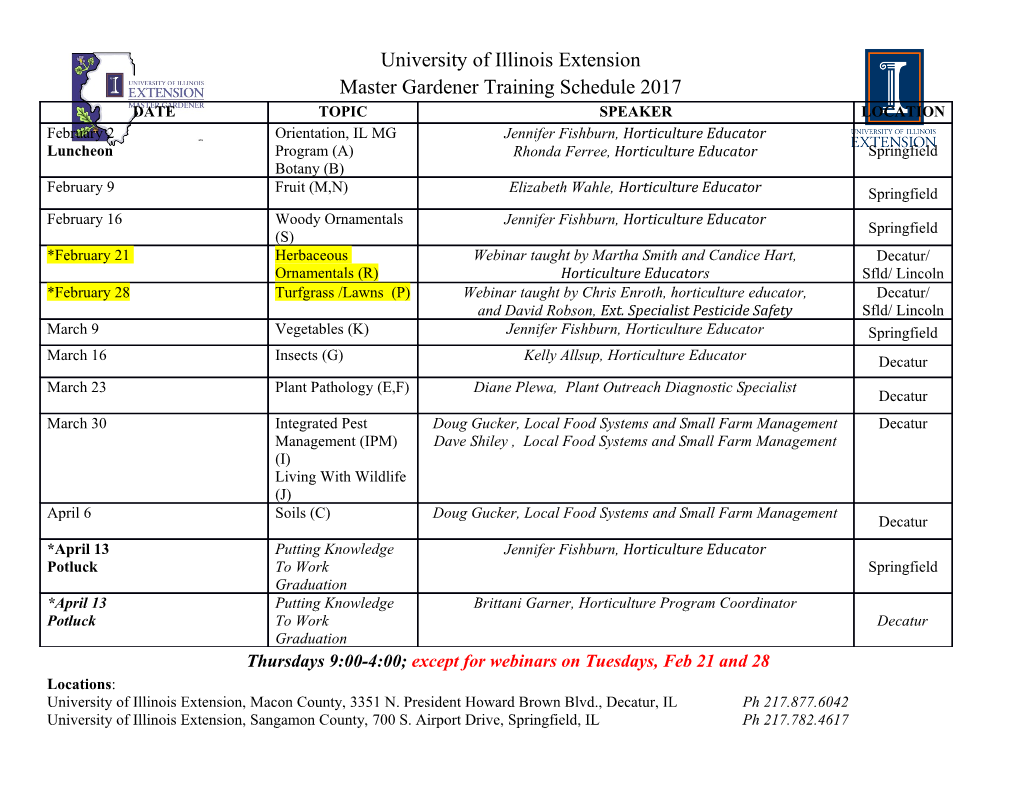
Eotv¨ os¨ Lor and´ University Faculty of Informatics Department of Programming Languages and Compilers Efficient computation of multidimensional data structures on CPU platform Supervisors: Departments of Computer Algebra and Prog. Lang. & Compilers Author: Attila Kov´acs Zal´an V´egv´ari Associate professor Software Information Technology BSc. Melinda T´oth Assistant lecturer ELTE-IK, PNYF Budapest, 2018. T´emabejelent˝o 1 Contents 1 Introduction 1 1.1 Fractals .................................. 1 1.1.1 Whatisafractal?......................... 1 1.1.2 Calculatingfractals. 2 1.1.3 TheEscape-TimeAlgorithm. 3 1.2 Generalization............................... 3 1.2.1 ExtendingTheEscape-TimeAlgorithm. 3 1.2.2 Extendingthespace ....................... 4 1.3 Auniversaltool.............................. 5 1.4 Scripting.................................. 5 1.5 Userinterface ............................... 6 2 User documentation 8 2.1 Thesolution................................ 8 2.2 NViewer.................................. 8 2.3 Graphicalengine ............................. 9 2.3.1 Thetransformationmatrix. 9 2.3.2 Thefunctions ........................... 10 2.3.3 Thelayers............................. 11 2.4 Goals.................................... 13 2.5 Thelanguage ............................... 13 2.5.1 Modules .............................. 14 2.5.2 Modes ............................... 14 2.5.3 Types ............................... 15 2.5.4 Parametersandstyles . 16 1 2.5.5 Queries .............................. 16 2.5.6 Definitions............................. 17 2.5.7 Nesteddefinitions. 17 2.5.8 Patterns .............................. 18 2.5.9 Branching ............................. 19 2.6 Standardlibrary ............................. 19 2.7 Toolusage................................. 23 2.7.1 Configuration ........................... 24 2.7.2 Sessions .............................. 24 2.7.3 Scripts............................... 25 2.7.4 Camera .............................. 25 2.7.5 Stylesandparameters . 26 2.7.6 Scenes ............................... 26 2.7.7 Exportingdata .......................... 27 3 Technical documentation 28 3.1 Problemdescription. 28 3.2 Enginestructure ............................. 29 3.3 Scriptstructure .............................. 30 3.3.1 Typesystem............................ 30 3.3.2 Functionstructure . 31 3.3.3 Informationflow . 31 3.4 Importingtheengine . 32 3.5 Importingthescriptparser. 32 3.6 Extendingthescriptlanguage . 33 3.7 Optimizations............................... 33 3.7.1 Functionstructure . 34 3.7.2 Constants ............................. 34 3.7.3 Memorymanagement. 34 3.8 Comparism ................................ 35 3.8.1 C++................................ 35 3.8.2 Haskell............................... 35 3.9 Examples ................................. 36 2 4 Summary 41 4.1 English................................... 41 4.2 Magyar .................................. 42 5 Results & testing 43 5.1 Testingplan................................ 43 5.2 Scripts................................... 44 Bibliography 48 6 Appendix 49 3 Abstract NViewer is a tool for visualizing N-dimensional shapes, more specifically fractals. This is not a tool to speed up something that was already known, it aims to uncover novel options for us. This tool allows us to be able to look into higher dimensions with an easy to handle camera technique. Further it allows us to discover structures unimaginable for us. NViewer provides us an easy way to transfer mathematical objects from paper to screen, in form of a scripting language. Additionally we are also providing a new type of graphical engine to render the results. Chapter 1 Introduction First, I intend to explain why this project was created and the problems it solves. The main objective is to create a tool that is easy to use not only for specific problems, but in more general cases. 1.1 Fractals Before we proceed any further, I need to clarify a number of concepts. 1.1.1 What is a fractal? A fractal is a geometrical or physical structure having an irregular or fragmented shape at all scales of measurement between a greatest and smallest scale such that certain mathematical or physical properties of the structure, as the perimeter of a curve or the flow rate in a porous medium, behave as if the dimensions of the structure (fractal dimensions) are greater than the spatial dimensions. [1] When somebody asks me what fractals are and I am to answer in a simple way, I would answer that these object represent a form of art created by mathematics and logic. 1 Figure 1.1: The mandelbrot set 1.1.2 Calculating fractals While fractals can be created in many different ways, they appear even in Nature. Plant development often shows fractal-like structures. Typical examples include plants such as broccoli, pinecone and basically most leaves. [4] In this project I will discuss only those, generated using com- puters. Some basic methods can be implemented in comenius logo in a recursive graphical representation pattern. A more complex way is to choose a set of points and run them across an iterated function system, also referred to as IFS [6]. In this case the set of points will be transformed in each step Figure 1.2: Broccoli which, in some cases, will approximate a set, which can be, to some extent, a fractal. As shown below, the nviewer project is capable of visualizing these types of fractals in an inefficient way, however my primary objective was to work with The Escape-Time Algorithm and some other similar algorithms. 2 1.1.3 The Escape-Time Algorithm The Escape-Time Algorithm is a widely used algorithm to visualize fractals. The basic idea is to use an iteration func- tion and test all points in the observed space to test if the iteration is convergent or not. If so, the point belongs to the fractal. Although this task is not entirely possible using a computer, approximations can be conducted. The most fa- mous application of this technique is the Mandelbrot Set [2]. Figure 1.3: Mandel- The convergence is tested after each iteration, as follows: if brot using low itera- the length of the current vector is greater than a specific tion settings value, it is not convergent and the iteration is interrupted. If this does not happen in a specific number of iterations, the original point (where the iteration stared), is part of the fractal at the current approximately level. For the points that are not part of the fractal, the time when they ”escape” is used for colouring, which both shows how the iterations approximates the fractal and provides an aestethical image. 1.2 Generalization Ok. Now a tool is available which is able to render 3D fractals, which is itself already useful, further, t many other objects can be created using the same approach. 1.2.1 Extending The Escape-Time Algorithm For proper functioning, the Escape-Time Algorithm requires the availability of the following features: per-pixel data management, graphical engine to render the re- sults, mathematical core to perform the necessary calculations at an acceptable speed. Once these features are available, it becomes easy to extend some of the features. The Escape-Time Algorithm provides escape-time to the points of the space, so that these can be coloured, where the colouring is carried out as a result 3 of a built-in process. Therefore, the graphical engine will not use this algorithm, but it will use more general functions that assign colours to points. This way, the colouring methods for the Escape-Time Algorithm can ban modified manually or it allows the implementation of various algorithms. At this point, we are able to create a function that colours each point in the space for rendering. It allows us to render any shape or object that can be expressed in such a function. Though this is not enough, we cannot yet apply light or any other effect to the surface. This sounds unimportant for the first time, because it seems to be only a special effect. When working in 3D, lighting is one of the most important tasks, because it allows us to visualize the surface of the object. Figure 1.4 shows a part of the mandelbox with and without lighting. If no light- ing is present, fog effect had to be used with a special colouring to make a surface patterns visible. Without these effects, only the outline of the mandelbox is visible. The second image show what it looks like, when lights are applied. There is a slight green fog that makes the distances more visible. So far this can be done using the graphical engine, but it might not satisfy all our needs, because lighting is applied in the same way to all points of the surface. This issue is continued (section 2.3.3). (a) Without lighting (b) With lighting Figure 1.4: Mandelbox with and without lighting 1.2.2 Extending the space A lot of 2D fractals have been recognized. They are easy to generate, easy to examine and at the same time they are beautiful. Additionally, three and more dimensional 4 fractals are known. Of course, besides fractals, a 4D model of the black holes has also been described [3]. This creates a need for a graphical engine that is capable of rendering N-dimensional objects. Usually, tools that operate in N-dimension, simply conduct a 2D or 3D projection of the original object. Is that enough? Projections cover some details, because only the surface will be visible. 1.3 A universal tool Most tools are designed to serve one purpose only. That is not wrong at all, because specialized tools are usually more optimized, but they have a great disadvantage: they
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages59 Page
-
File Size-