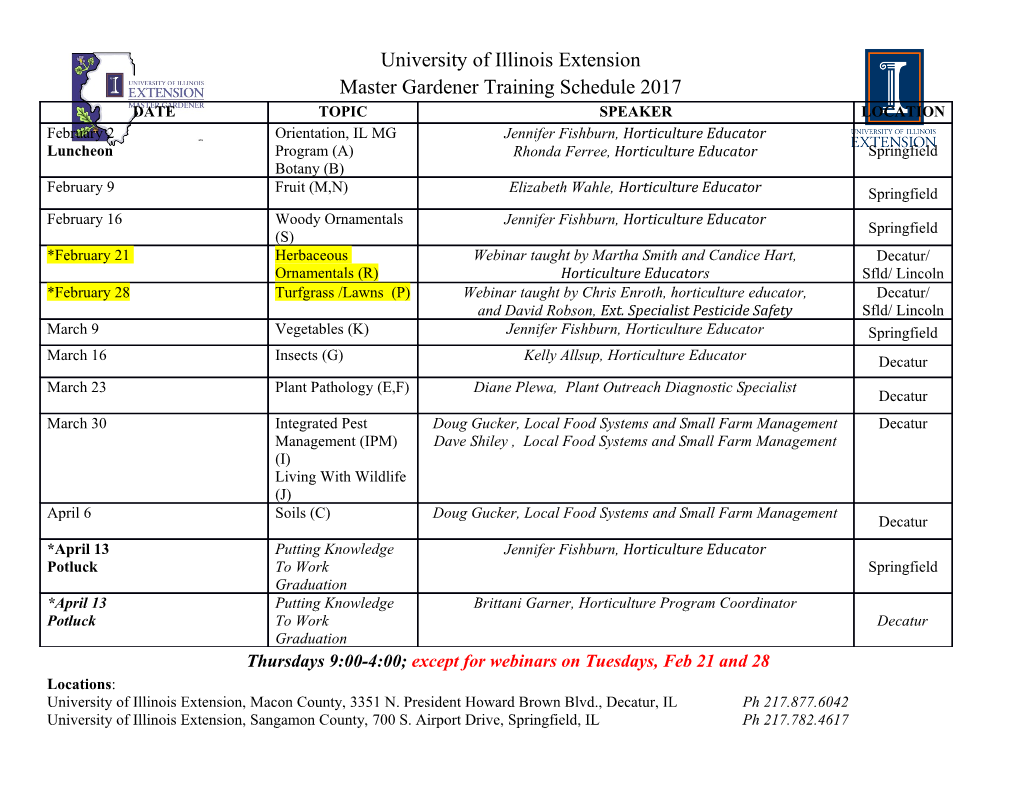
Verifying Linked Data Structure Implementations Karen Zee Viktor Kuncak Martin Rinard MIT CSAIL EPFL I&C MIT CSAIL Cambridge, MA Lausanne, Switzerland Cambridge, MA [email protected] viktor.kuncak@epfl.ch [email protected] Abstract equivalent conjunction of properties. By processing each conjunct separately, Jahob can use different provers to es- The Jahob program verification system leverages state of tablish different parts of the proof obligations. This is the art automated theorem provers, shape analysis, and de- possible thanks to formula approximation techniques[10] cision procedures to check that programs conform to their that create equivalent or semantically stronger formulas ac- specifications. By combining a rich specification language cepted by the specialized decision procedures. with a diverse collection of verification technologies, Jahob This paper presents our experience using the Jahob sys- makes it possible to verify complex properties of programs tem to obtain full correctness proofs for a collection of that manipulate linked data structures. We present our re- linked data structure implementations. Unlike systems that sults using Jahob to achieve full functional verification of a are designed to verify partial correctness properties, Jahob collection of linked data structures. verifies the full functional correctness of the data structure implementation. Not only do our results show that such full functional verification is feasible, the verified data struc- 1 Introduction tures and algorithms provide concrete examples that can help developers better understand how to achieve similar results in future efforts. Linked data structures such as lists, trees, graphs, and hash tables are pervasive in modern software systems. But because of phenomena such as aliasing and indirection, it 2 Example has been a challenge to develop automated reasoning sys- tems that are capable of proving important correctness prop- In this section we use a verified association list to demon- erties of such data structures. strate how developers specify data structure implementa- Jahob is a program verification system that leverages a tions in Jahob. Figure 1 presents selected portions of the rich specification language and a diversity of automated the- AssocList class. This class maintains a list of key, value oremprovers and decision procedures to verify complex pairs. Our system works with Java programs augmented properties of programs that manipulate linked data struc- with specifications. The specifications appear as special tures. Our specifications use abstract sets and relations to comments of the form /*: ... */ or //:, enabling the use of stan- characterize the abstract state of the data structure. A ver- dard Java compilers and virtual machines. The first com- ified abstraction function defines abstract sets and relations ment in Figure 1 identifies the abstract state content of the in terms of concrete objects and references that the imple- association list as a relation in the form of a set of pairs of mentation manipulates at run time. Specifications use ab- objects (we present the abstraction function that defines this stract sets and relations to state externally visible properties abstract state below).1 of the data structure state and to specify method precondi- The put(k0,v0) method inserts the pair (k0,v0) into the tions and postconditions. These specifications capture all of association list, returning the previous association for k0 (if the semantic information that the developer needs to use the such an association exists). The requires clause indicates data structure. Jahob establishes desired program properties using a 1Our examples use mathematical notation for concepts such as set number of external decision procedures, Nelson-Oppen union and universal quantification. While our source code files encode provers, and first-order provers. It automatically generates this notation in text, Isabelle’s version of the ProofGeneral Emacs mode renders the specifications within Java code in mathematical notation. The verification condition formulas from the program and its figures correspond to what the user sees on the screen in such mode. See specification, then splits each verification condition into an the screen shots at http://javaverification.org. 978-1-4244-1694-3/08/$25.00 ©2008 IEEE 1 Authorized licensed use limited to: MIT Libraries. Downloaded on September 7, 2009 at 09:45 from IEEE Xplore. Restrictions apply. class AssocList f vardefs ”content == first.. cnt”; // : public specvar content :: ”(obj ∗ obj) set” invariant CntDef: public Object put(Object k0, Object v0) ”8 x.x 2 Node ^ x 2 alloc ^ x 6= null ! /∗ : requires ”k0 6= null ^ v0 6= null” x.. cnt= f(x.. key,x.. value) g[ x..next..cnt ^ modifies content (8 v.(x.. key,v) 2= x..next..cnt)”; ensures invariant CntNull: ”( result= null ! content= old content [f (k0, v0)g) ^ ”8 x.x 2 Node ^ x 2 alloc ^ x= null ! x..cnt= fg”; ( result 6= null ! private static specvar edge ::”obj ) obj ) bool”; content= old content −f (k0, result) g[f (k0, v0)g)” ∗/ vardefs ”edge ==( λ xy.(x 2 Node ^ y=x..next) _ f...g (x 2 AssocList ^ y=x..first))”; g invariant InjInv: Figure 1. Association List put method ”8 x1 x2y.y 6= null ^ edge x1y ^ edge x2y ! x1=x2”; Figure 3. Abstraction Function and Selected public /∗: claimedby AssocList ∗/ class Node f Invariants public Object key; public Object value; public Node next; // : public ghost specvar cnt :: ”(obj ∗ obj) set” = ”fg” g Figure 2. Node Definition mantic domain in which the verification takes place. The domain is an infinite set of objects. Classes correspond to sets of objects within this domain. Fields correspond to to- that it is the client’s responsibility to ensure that neither k0 tal functions from objects to values— each object has all of nor v0 is null. The modifies clause indicates that the method the fields from all of the classes. If the object is not a mem- observably changes nothing except the abstract state con- ber of a given class, the values of all of the fields from that tent of the association list. The ensures clause states that class are simply null. For example, x 2 Node states that ob- the abstract state content after the method executes is the ject x has class Node. The expression x..next is a shorthand abstract state old content from before the method executed for the application of function next to object x, with the next augmented with the new association (k0,v0). Any previous function modeling the Java field next. association (k0,result) is removed from the association list, with result returned as the result of the put method. It re- turns null if no such previous association exists. public Object get(Object k0) Figure 2 presents the definition of the Node class, which /∗ : requires ”k0 6= null” ensures ”(result 6= null ! (k0, result) 2 content) ^ contains the key, value, and next fields that implement the ( result= null !: (9 v.(k0,v) 2 content))” ∗/ linked list of key, value pairs in the association list. The f assertion claimedby AssocList specifies that only the meth- Node current = first ; while /∗: inv ”8 v.((k0,v) 2 content)= ods in the AssocList class can access these fields. Jahob ((k0,v) 2 current..cnt)” ∗/ enforces this visibility condition by a syntactic check. (current != null ) f if (current.key == k0) f return current.value; g Each Node object has a specification variable cnt. This current = current.next; variable holds a set of pairs representing all of the associa- g tions in the part of the association list starting at the given return null ; g Node object. It is initialized to the empty set, is explicitly Figure 4. Implementation of the get method updated as the implementation manipulates the list of nodes, and is conceptually part of the state of the corresponding Node object. It is used only during the verification of the Figure 4 presents the implementation of the get(k0) association list and does not exist when the program runs. method. This method searches the list to find the Node con- The purpose of the cnt variable in the specification is to de- taining the key k0, then returns the corresponding value v fine the abstract state content of the association list. (or null if no such value exists). The loop invariant states Figure 3 presents the invariants that characterize the cnt that the pair (k0, value) is in the association list if and only specification variable and the corresponding abstract state if it is in the part of the list remaining to be searched—in content of the association list. The variable first holds a ref- effect, that the the search does not skip the Node with key erence to the first Node in the linked list that implements k0. Given the specification and the invariants, Jahob is ca- the association list; the abstract state content is the value of pable of verifying that this method 1) correctly implements the cnt specification variable of the first node in the list. The its specification, and 2) correctly preserves the invariants. CntDef invariant recursively defines cnt for non-null nodes In addition to this method, the association list contains as the key, value pair in the node union the value of cnt for other methods that check membership of keys in the asso- the next node in the list. The CntNull invariant defines cnt ciation list, add associations to the list, and remove associa- as empty for the null object. tions from the list. For each of these methods, Jahob is able The syntax of these invariants reflects the underlying se- to statically verify full functional specifications. 2 Authorized licensed use limited to: MIT Libraries. Downloaded on September 7, 2009 at 09:45 from IEEE Xplore. Restrictions apply. provides a facility to spawn provers in parallel and succeed MONA SPASS E CVC3 Z3 as soon as at least one of them succeeds.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-