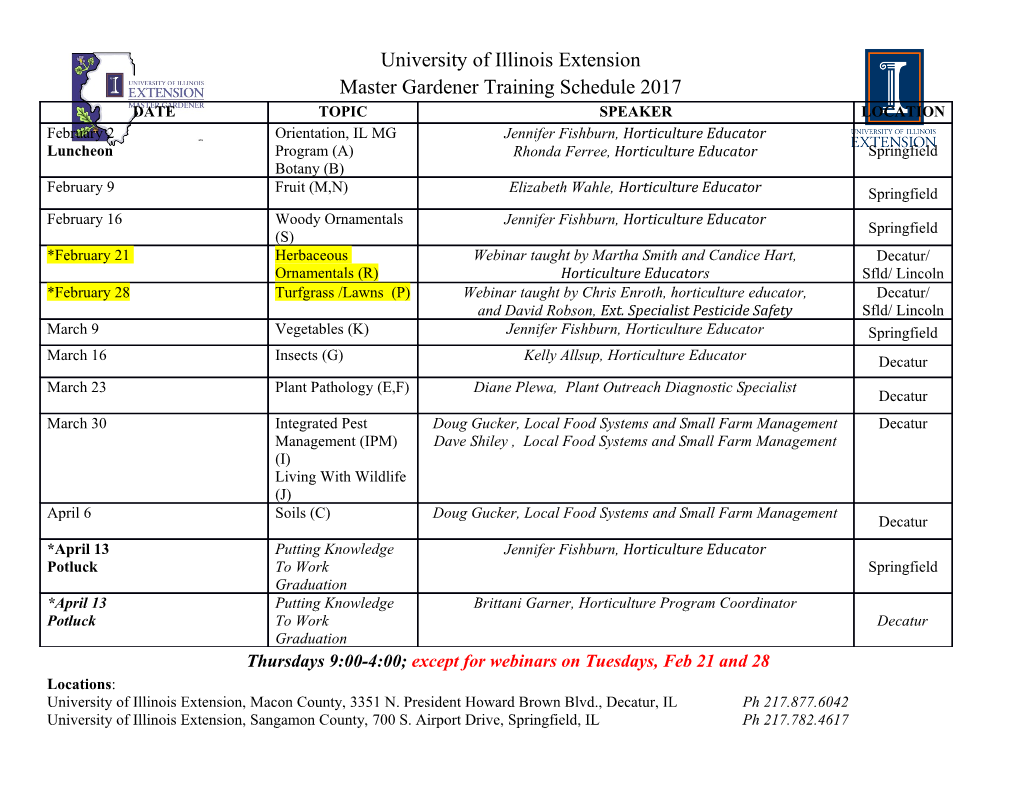
Understanding Programming Languages M. Ben-Ari Weizmann Institute of Science Originally published by John Wiley & Sons, Chichester, 1996. Copyright °c 2006 by M. Ben-Ari. You may download, display and print one copy for your personal use in non-commercial academic research and teaching. Instructors in non-commerical academic institutions may make one copy for each student in his/her class. All other rights reserved. In particular, posting this document on web sites is prohibited without the express permission of the author. Contents Preface xi I Introduction to Programming Languages 1 1 What Are Programming Languages? 2 1.1 The wrong question . 2 1.2 Imperative languages . 4 1.3 Data-oriented languages . 7 1.4 Object-oriented languages . 11 1.5 Non-imperative languages . 12 1.6 Standardization . 13 1.7 Computer architecture . 13 1.8 * Computability . 16 1.9 Exercises . 17 2 Elements of Programming Languages 18 2.1 Syntax . 18 2.2 * Semantics . 20 2.3 Data . 21 2.4 The assignment statement . 22 2.5 Type checking . 23 2.6 Control statements . 24 2.7 Subprograms . 24 2.8 Modules . 25 2.9 Exercises . 26 v Contents vi 3 Programming Environments 27 3.1 Editor . 28 3.2 Compiler . 28 3.3 Librarian . 30 3.4 Linker . 31 3.5 Loader . 32 3.6 Debugger . 32 3.7 Profiler . 33 3.8 Testing tools . 33 3.9 Configuration tools . 34 3.10 Interpreters . 34 3.11 The Java model . 35 3.12 Exercises . 37 II Essential Concepts 38 4 Elementary Data Types 39 4.1 Integer types . 39 4.2 Enumeration types . 43 4.3 Character type . 46 4.4 Boolean type . 47 4.5 * Subtypes . 48 4.6 * Derived types . 50 4.7 Expressions . 52 4.8 Assignment statements . 55 4.9 Exercises . 57 5 Composite Data Types 59 5.1 Records . 59 5.2 Arrays . 62 5.3 Reference semantics in Java . 64 5.4 Arrays and type checking . 66 5.5 * Array subtypes in Ada . 69 5.6 String type . 70 5.7 Multi-dimensional arrays . 72 Contents vii 5.8 Array implementation . 73 5.9 * Representation specification . 76 5.10 Exercises . 80 6 Control Structures 82 6.1 switch-/case-statements . 82 6.2 if-statements . 86 6.3 Loop statements . 91 6.4 for-statements . 94 6.5 Sentinels . 99 6.6 * Invariants . 100 6.7 goto-statements . 102 6.8 Exercises . 103 7 Subprograms 105 7.1 Subprograms: procedures and functions . 105 7.2 Parameters . 107 7.3 Passing parameters to a subprogram . 109 7.4 Block structure . 117 7.5 Recursion . 122 7.6 Stack architecture . 124 7.7 More on stack architecture . 130 7.8 * Implementation on the 8086 . 132 7.9 Exercises . 134 III Advanced Concepts 136 8 Pointers 137 8.1 Pointer types . 137 8.2 Data structures . 143 8.3 Dynamic data structures in Java . 149 8.4 Equality and assignment in Java . 149 8.5 Memory allocation . 151 8.6 Algorithms for heap allocation . 153 8.7 Exercises . 156 Contents viii 9 Real Numbers 157 9.1 Representations of real numbers . 157 9.2 Language support for real numbers . 160 9.3 The three deadly sins . 163 9.4 * Real types in Ada . 165 9.5 Exercises . 166 10 Polymorphism 168 10.1 Type conversion . 168 10.2 Overloading . 169 10.3 Generics . 171 10.4 Polymorphic data structures in Java . 174 10.5 Variant records . 175 10.6 Dynamic dispatching . 178 10.7 Exercises . 179 11 Exceptions 180 11.1 Exception handling requirements . 180 11.2 Exceptions in PL/I . 181 11.3 Exceptions in Ada . 182 11.4 Exceptions in C++ . 184 11.5 Error handling in Eiffel . 186 11.6 Exercises . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages322 Page
-
File Size-