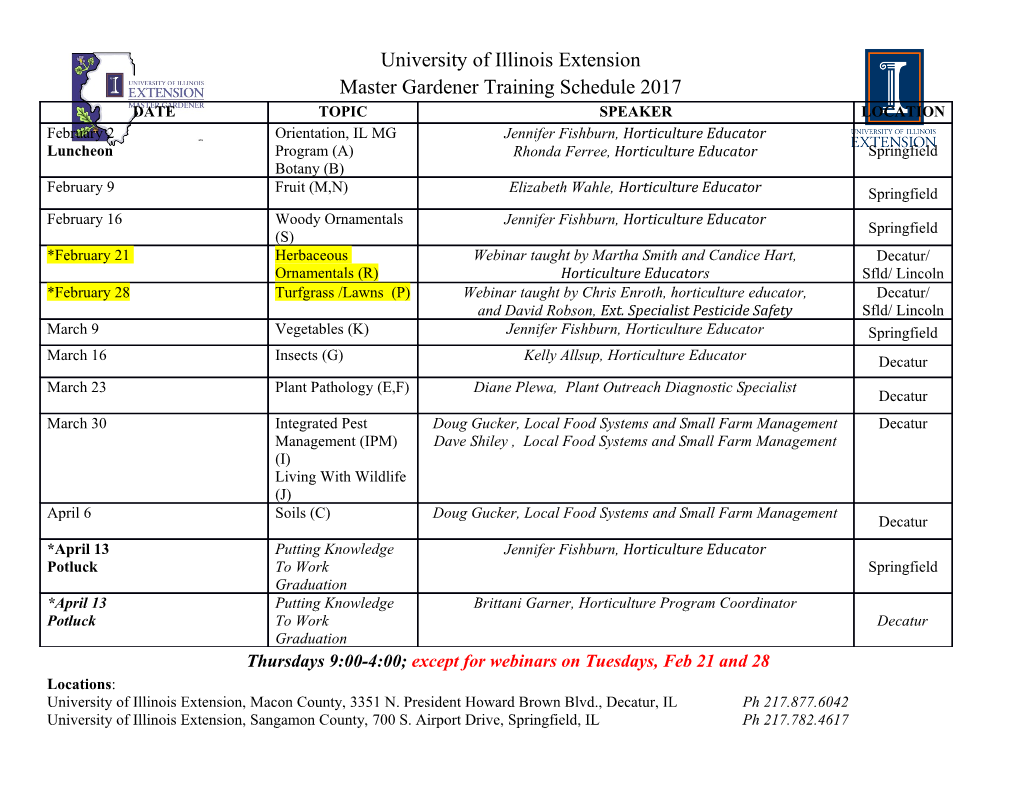
CSC 407/507 Programming Assignment #1: Common Lisp Due Date: Friday, February 2 Remember you can work in groups of up to three students. Download a version of Common Lisp. Two free (but trial limited) versions are available at Franz Common Lisp (http://www.franz.com/downloads/) and LispWorks (http://www.lispworks.com/). Another version is called Steel Bank Common Lisp which does not come with an IDE, just a command line interface (https://common-lisp.net/downloads/). A GNU’s Common Lisp is available for both Unix/Linux (https://www.gnu.org/software/gcl/) and Windows (https://www.cs.utexas.edu/users/novak/gclwin.html). LispWorks is recommended as the easiest and least fuss. In this program, you will write multiple Lisp functions which support (or implement wholly) sorting algorithms. Some functions may serve multiple sort algorithms if you can work it out although this is not necessary. You should aim to build helper functions so that you are not implementing any single sort in a single function. Some algorithms will require helper functions (e.g., mergesort which has at least the recursive mergesort function and the merge function). You are to implement five of the following seven sort algorithms: bubblesort, heapsort, insertionsort, mergesort, quicksort, radixsort and selectionsort. You must do at least one of mergesort or quicksort among the five you implement. You are to use recursion for the mergesort/quicksort functions and are encouraged to use recursion as much as possible in the remainder of your functions whether they are helper functions or the sort functions. Use lists to store the values to be sorted (they will all be integer numbers) rather than arrays. Your functions must then manipulate the lists of values in order to sort them. Implement the sorts to sort in ascending order. For radixsort, instead of having an array of 10 queues, you will use a list of lists, for instance after the first pass where the rightmost digit has been sorted, you might have ((400 240 180 420) (431 201 661 451) (232 802 522) () (654 434) () () (107) (408 508) ()). The () are currently empty lists because there are no numbers that end in 3, 5, 6 or 9. On the course website, there are three documents to look over. One has the seven sorting algorithms in pseudocode (keep in mind that your implementation will require the user of lists, not arrays). The second is a description of Common Lisp functions that you might want to use. Some of these descriptions include examples although not all of them do. You do not have to use all of these functions and in some cases may either pick between possibilities (e.g., cons versus append) or find other ways to implement your functions. In my solutions, I used most of them. The third file contains numerous Common Lisp functions to help clarify how to use the various functions and perhaps to inspire you in how to implement the sorting algorithms. There are additional examples of Common Lisp functions available at http://www.nku.edu/~foxr/CSC375/code.html if you want to see more about Common Lisp (but keep in mind that this site’s files was provided to support a full course on Common Lisp and so may not be particularly helpful for this assignment). Run each of your sort functions on at least four different lists of numbers as follows: a list of 1 number an already sorted list an already sorted list in descending order a list of at least 10 unsorted numbers The output when running the function should return the sorted list so your output might look like this (1 2 3 4 6 8 9). Submit the following: 1. All of your functions (and don’t forget to comment them!) 2. Example output of each of your five sorting algorithms in use. Run each on 1-2 samples. You can copy and paste the results with the program code. A sample run might look like this. CL-USER 183 > (bubblesort ’(6 3 18 4 2 9)) (2 3 4 6 9 18) 3. A report that explains the following. a. Who implemented what in your group b. How challenging was it to encode each of your sorts in Common Lisp? Were some of Common Lisp’s functions particularly useful? c. Would this assignment have been easier or harder had you been able to use arrays? Explain. d. Did you find the language easy, hard or about average to learn and use? What aspects of the language were hardest to learn and use? What aspects of the language did you enjoy the most? e. Would you ever choose to use this language again and if so, under what circumstances? f. Add anything else you want to say. .
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages2 Page
-
File Size-