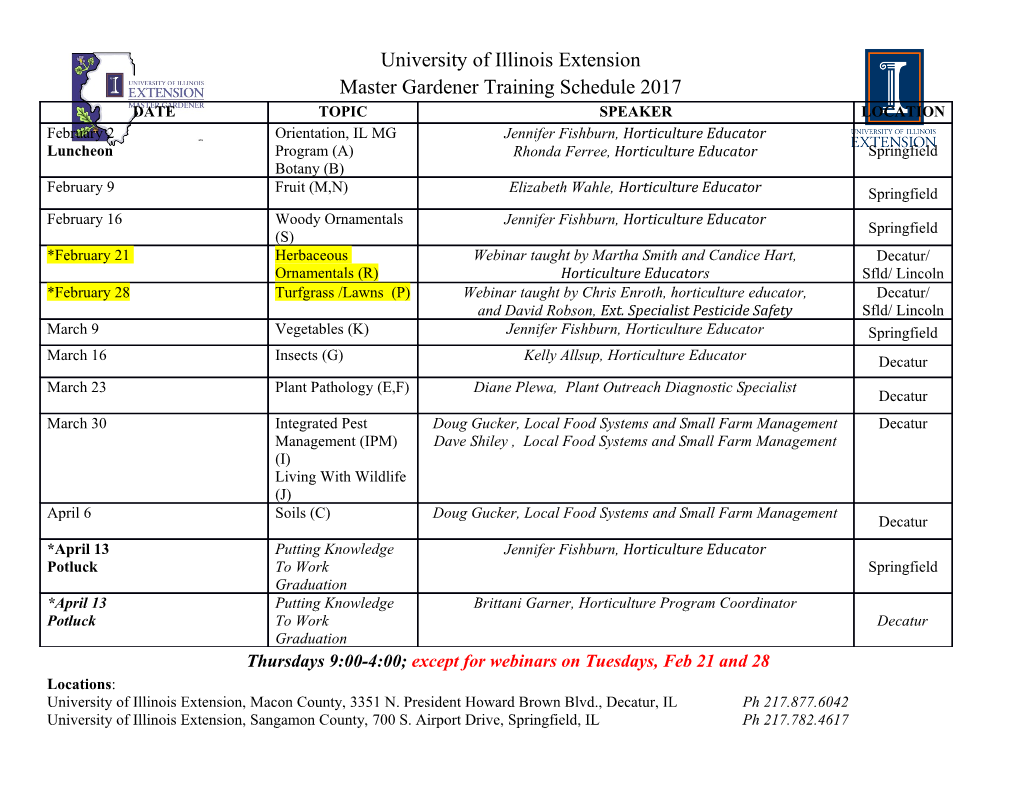
ptg16091132 www.it-ebooks.info The Go Programming Language ptg16091132 www.it-ebooks.info This page intentionally left blank ptg16091132 www.it-ebooks.info The Go Programming Language Alan A. A. Donovan Google Inc. Brian W. Kernighan ptg16091132 Princeton University New York • Boston • Indianapolis • San Francisco Toronto • Montreal • London • Munich • Paris • Madrid Capetown • Sydney • Tokyo • Singapore • Mexico City www.it-ebooks.info Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in this book, and the publisher was aware of a trade- mark claim, the designations have been printed with initial capital letters or in all capitals. T e authors and publisher have taken care in the preparation of this book, but make no expressed or implied warranty of any kind and assume no responsibility for errors or omissions. No liability is assumed for incidental or consequential damages in connection with or arising out of the use of the information or programs contained herein. For information about buying this title in bulk quantities, or for special sales opportunities (which may include electronic versions; custom cover designs; and content particular to your business, train- ing goals, marketing focus, or branding interests), please contact our corporate sales department at [email protected] or (800) 382-3419. For government sales inquiries, please contact [email protected]. For questions about sales outside the United States, please contact [email protected]. ptg16091132 Visit us on the Web: informit.com/aw Library of Congress Control Number: 2015950709 Copyright © 2016 Alan A. A. Donovan & Brian W. Kernighan All rights reserved. Printed in the United States of America. T is publication is protected by copyright, and permission must be obtained from the publisher prior to any prohibited reproduction, storage in a retrieval system, or transmission in any form or by any means, electronic, mechanical, photocopying, recording, or likewise. To obtain permission to use material from this work, please submit a written request to Pearson Education, Inc., Permissions Department, 200 Old Tappan Road, Old Tappan, New Jersey 07675, or you may fax your request to (201) 236-3290. Front cover: Millau Viaduct, Tarn valley, southern France. A paragon of simplicity in modern engi- neering design, the viaduct replaced a convoluted path from capital to coast with a direct route over the clouds. © Jean-Pierre Lescourret/Corbis. Back cover: the original Go gopher. © 2009 Renée French. Used under Creative Commons Attribu- tions 3.0 license. Typeset by the authors in Minion Pro, Lato, and Consolas, using Go, grof , ghostscript, and a host of other open-source Unix tools. Figures were created in Google Drawings. ISBN-13: 978-0-13-419044-0 ISBN-10: 0-13-419044-0 Text printed in the United States on recycled paper at RR Donnelley in Crawfordsville, Indiana. First printing, October 2015 www.it-ebooks.info The Go Programming Language © 2016 Alan A. A. Donovan & Brian W. Kernighan revision 3b600c, date 29 Sep 2015 Fo r Leil a an d Me g ptg16091132 www.it-ebooks.info This page intentionally left blank ptg16091132 www.it-ebooks.info The Go Programming Language © 2016 Alan A. A. Donovan & Brian W. Kernighan revision 3b600c, date 29 Sep 2015 Cont ents Pr eface xi TheOrigins ofGoxii TheGoPro jec t xiii Organizat ionofthe Bookxv ptg16091132 Wh ere toFindMoreInfor mat ionxvi Ac knowledgments xvii 1. Tutorial1 1.1. Hel lo, Wor ld1 1.2. Command-L ineArguments 4 1.3. FindingDup lic ateLines 8 1.4. Animated GIFs 13 1.5. FetchingaURL 15 1.6. FetchingURLs Con cur rently17 1.7. A We b Server 19 1.8. Loose End s 23 2. Pro gramStr ucture27 2.1. Names 27 2.2. Declarat ions 28 2.3. Var iables 30 2.4. Assig nments 36 2.5. Typ e Decl arat ions 39 2.6. Packages andFiles 41 2.7. Scope 45 vii www.it-ebooks.info The Go Programming Language © 2016 Alan A. A. Donovan & Brian W. Kernighan revision 3b600c, date 29 Sep 2015 viii CONTENTS 3. Basic Data Typ es51 3.1. Int egers 51 3.2. Float ing-Point Numbers 56 3.3. Complex Numbers 61 3.4. Boole ans63 3.5. Str ings64 3.6. Con stants75 4. Com positeTyp es81 4.1. Arrays 81 4.2. Slices 84 4.3. Maps 93 4.4. Str ucts99 4.5. JSON107 4.6. Text andHTMLTempl ates113 5. Func tions 119 5.1. FunctionDeclarat ions 119 5.2. Rec ursion121 5.3. MultipleRetur n Values 124 5.4. Erro rs127 5.5. FunctionValues 132 ptg16091132 5.6. Anony mou s Func tions 135 5.7. Var iadic Functions 142 5.8. Defer red FunctionCal ls143 5.9. Panic 148 5.10. Recov er151 6. Metho ds 155 6.1. Met hod Declarat ions 155 6.2. Met hodswit h aPoint erReceiver158 6.3. ComposingTyp es by Str uct Emb edding161 6.4. Met hod Values andExpressions 164 6.5. Example: Bit Vec tor Typ e 165 6.6. Encapsulat ion168 7. Interfaces171 7.1. Int erfaces as Contrac ts 171 7.2. Int erface Typ es 174 7.3. Int erface Satisfac tion175 7.4. ParsingFlags wit h flag.Value 179 7.5. Int erface Values 181 www.it-ebooks.info The Go Programming Language © 2016 Alan A. A. Donovan & Brian W. Kernighan revision 3b600c, date 29 Sep 2015 CONTENTSix 7.6. Sor tingwit h sort.Interface 186 7.7. The http.Handler Interface 191 7.8. The error Interface 196 7.9. Example: ExpressionEvaluator197 7.10. Typ e As ser tions 205 7.11. Discriminat ingError s with Typ e As ser tions 206 7.12. Quer yingBeh avior s with Int erface Typ e As ser tions 208 7.13. Typ e Sw itch es210 7.14. Example: Token-B ased XML Decoding213 7.15. A Fe w Wo rds ofAdv ice 216 8. Gor o utines and Channels 217 8.1. Goroutines217 8.2. Example: Con cur rentClo ckSer ver 219 8.3. Example: Con cur rentEch o Server 222 8.4. Channel s 225 8.5. Looping inParal lel234 8.6. Example: Con cur rentWeb Craw ler 239 8.7. Multiplexingwit h select 244 8.8. Example: Con cur rentDirec tor y Traversal247 8.9. Cancellat ion251 8.10. Example: ChatSer ver 253 ptg16091132 9. Concurrency withShared Vari ables257 9.1. Race Con dit ion s 257 9.2. Mut ual Exc lusion: sync.Mutex 262 9.3. Read/Write Mut exes: sync.RWMutex 266 9.4. Memor y Sy nchro nizat ion267 9.5. Lazy Initializat ion: sync.Once 268 9.6. TheRace Detec tor 271 9.7. Example: Con cur rentNon-Blo cking Cache 272 9.8. Goroutinesand Threads 280 10. Pack ages and the GoTool283 10.1. Int roduc tion283 10.2. Imp ort Pat hs284 10.3. ThePackageDeclarat ion285 10.4. Imp ort Declarat ions 285 10.5. Blank Imp orts286 10.6. Packages andNaming289 10.7. TheGoTool290 www.it-ebooks.info The Go Programming Language © 2016 Alan A. A. Donovan & Brian W. Kernighan revision 3b600c, date 29 Sep 2015 xCONTENTS 11. Testing 301 11.1. The go test To ol302 11.2. Test Func tions 302 11.3. Cov erage318 11.4. Benchmark Func tions 321 11.5. Profiling323 11.6. Example Func tions 326 12. Reflecti on329 12.1. Why Reflec tion?329 12.2. reflect.Type and reflect.Value 330 12.3. Display,aRec ursiveValue Print er333 12.4. Example: Enco dingS-E xpressions 338 12.5. Setting Var iables wit h reflect.Value 341 12.6. Example: DecodingS-E xpressions 344 12.7. AccessingStr uct Field Tags 348 12.8. Displaying the Met hodsofaTyp e 351 12.9. A Wo rdofCaution 352 13. Low-L evel Pro gramming353 13.1. unsafe.Sizeof, Alignof,and Offsetof 354 13.2. unsafe.Pointer 356 ptg16091132 13.3. Example: DeepEquivalence 358 13.4. Cal lingCCodewit h cgo 361 13.5. Another WordofCaution 366 Index367 www.it-ebooks.info The Go Programming Language © 2016 Alan A. A. Donovan & Brian W. Kernighan revision 3b600c, date 29 Sep 2015 Pref ace ‘‘Go is anopensourc e prog rammi n g lang uagethatmak esiteasytobui ldsimpl e,rel iab le, an d efficientsof tware.’’ (Fro m theGoweb sit e at golang.org) Go was conceive d in Septemb er2007 byRob ert Gries emer,Rob Pike, and Ken Thomps on, all ptg16091132 at Google, and was announced inNov emb er2009. Thegoals ofthe langu ageand its accom- pany ing tools weretobeexpressive, efficient in bot h comp ilation and exe cut ion,and effec tive in writing reliableand robustprograms. Go bears a sur face simi lar ity toC and,likeC,isatoolfor prof essionalprogrammers, achie v- ingmaximum effe ctwit h minimum means.But it ismuchmorethananupdated versionof C. Itbor rowsand adaptsgood ide as from manyother langu ages, whi leavoidingfeaturesthat have led tocomplexity and unreliablecode. Its facilities for con cur rency are new and efficient, andits approach to dat aabstrac tionand obj e ct-oriente d prog rammingisunu sually flexible. It hasaut omat ic memory managementor garb age col lec tion. Go isesp eci ally wel l suit edfor bui ldinginf rastr ucturelikenet wor ked ser vers, andtools and systems for prog rammers, but it istruly a general-pur pos e language and find s us e in domains as divers e as graphics, mobileapp lic ations,and machinelearning. Ithas becom e popu lar as a repl acementfor unt ypedscr ipt ing langu ages because itbal ances expressivenesswit h safety : Go programstypic ally run fasterthanprogramswritt enindynamic langu ages andsuf fer far fe wer crashesdue tounexp ected typ e er ror s. Go isanopen-s ource pro jec t,sosourcecodefor itscompi ler,librar ies,andtools is fre ely avai l- able toany one.Contr ibution s to the pro jec t come fro m an active worldw ide community.Go runs onUnix-li kesystems—Linux, Fre eBSD, OpenBSD, Mac OS X—andonPlan9and Micros oft Windows.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages400 Page
-
File Size-