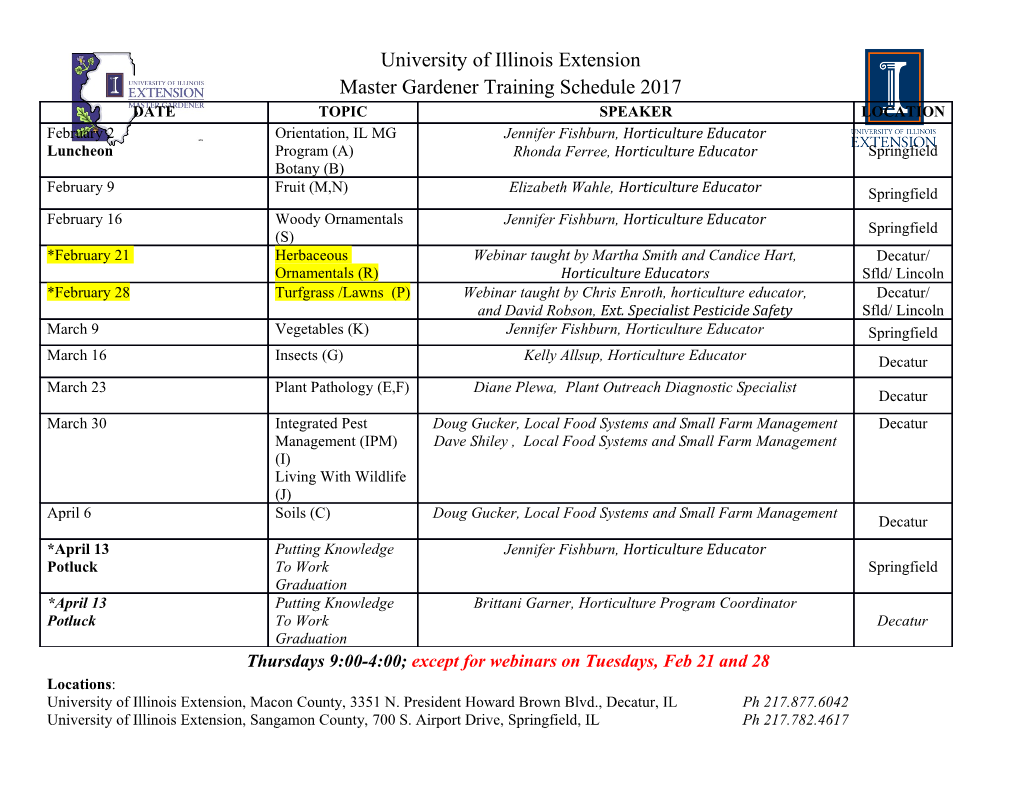
ATMCaseStudy,Part1: Object-Oriented Design with theUML 25 Actionspeakslouderthanwords butnot nearly as often. —Mark Twain Always design athing by consideringitinits next larger context. —Eliel Saarinen Oh,lifeisaglorious cycleof song. —Dorothy Parker TheWrightbrothers’ design … allowedthemtosurvive long enough to learnhow to fly. —MichaelPotts Objectives In this chapter you’ll learn: ■ Asimpleobject-oriented design methodology. ■ What arequirements document is. ■ To identify classes andclass attributes from a requirements document. ■ To identify objects’states, activities andoperationsfrom arequirements document. ■ To determine the collaborationsamong objects in asystem. ■ To work with theUML’s use case, class, state, activity, communication and sequence diagrams to graphically modelanobject- oriented system. © 2012 Pearson Education, Inc., Upper Saddle River, NJ. All Rights Reserved. 25-2 Chapter 25 ATMCaseStudy,Part1:Object-Oriented Design with theUML 25.1 Introduction 25.6 IdentifyingObjects’Statesand 25.2 IntroductiontoObject-Oriented Activities Analysis andDesign 25.7 IdentifyingClass Operations 25.3 Examiningthe ATMRequirements 25.8 Indicating CollaborationAmong Document Objects 25.4 Identifyingthe Classesinthe ATM 25.9 Wrap-Up RequirementsDocument 25.5 IdentifyingClass Attributes 25.1 Introduction Now we begin theoptionalportion of ourobject-orienteddesign andimplementation case study. In this chapterand Chapter26, you’ll design andimplementanobject-orientedau- tomatedteller machine (ATM) softwaresystem.The case studyprovides youwithacon- cise, carefully paced,completedesign andimplementation experience. You’ll perform the stepsofanobject-orienteddesign (OOD) process using theUML whilerelating themto theobject-oriented conceptsdiscussed in Chapters 2–13. In this chapter, you’ll work with sixpopular typesofUML diagrams to graphicallyrepresent thedesign. In Chapter26, you’ll tune the design with inheritanceand polymorphism, then fully implementthe ATMinan850-line C++application (Section 26.4). This is not an exercise; rather,it’sanend-to-end learning experiencethatconcludes with adetailedwalkthrough of the complete C++code that implements ourdesign. It will acquaint youwith the kindsofsubstantialproblems encountered in industry. Thesechapterscan be studiedasacontinuousunit after you’ve completed theintro- duction to object-oriented programming in Chapters 2–13.Or, youcan pace thesections afterChapters 3–7, 9and 13.Eachsection of thecasestudy beginswithanote telling you thechapterafter which it canbecovered. 25.2 IntroductiontoObject-OrientedAnalysisand Design Whatifyou were askedtocreateasoftwaresystemtocontrol thousandsofautomatedtell- er machinesfor amajor bank?Orsupposeyou were asked to work on ateamof1000soft- ware developers building thenextU.S.air trafficcontrol system. For projects so largeand complex, youcannotsimplysit down andstartwritingprograms. To createthe best solutions, youshould followaprocess for analyzing your project’s requirements (i.e.,determining what thesystemshould do) anddeveloping a design that satisfiesthem(i.e.,deciding how thesystemshould do it). Ideally,you’d go through this process andcarefully review thedesign (or haveyourdesign reviewed by othersoftware professionals) before writing anycode.Ifthis process involves analyzing anddesigning your systemfromanobject-oriented pointofview, it’s called an object-oriented analysis anddesign(OOAD)process.Analysisand design cansavemanyhours by helping youto avoid an ill-plannedsystem-developmentapproach that hastobeabandoned partofthe waythroughits implementation,possiblywasting considerabletime, moneyand effort. Smallproblems do notrequire an exhaustiveOOAD process.Itmay be sufficient to write pseudocode before youbegin writing C++code. © 2012 Pearson Education, Inc., Upper Saddle River, NJ. All Rights Reserved. 25.3 Examiningthe ATMRequirements Document 25-3 As problemsand thegroups of people solving them increaseinsize,the methods of OOAD becomemore appropriate than pseudocode. Ideally,members of agroupshould agreeonastrictly definedprocessfor solving theirproblem andauniformway of commu- nicating theresults of that process to oneanother.Although many different OOAD pro- cesses exist, asinglegraphicallanguagefor communicating theresults of any OOAD process hascomeintowide use. This language, knownasthe UnifiedModeling Language (UML), wasdeveloped in themid-1990sunderthe initialdirection of threesoftware methodologists—Grady Booch, JamesRumbaugh andIvarJacobson. 25.3Examining the ATM Requirements Document [Note: This section canbestudied afterChapter3.] We begin ourdesign process by presenting a requirements document that specifiesthe ATMsystem’soverall purposeand what it must do. Throughoutthe case study, we refer to therequirements document to determinewhatfunctionality thesystemmustinclude. Requirements Document Alocal bank intendstoinstallanewautomated teller machine(ATM) to allowusers (i.e., bank customers) to performbasic financialtransactions(Fig. 25.1). Each usercan have only oneaccount at thebank. ATMusers should be able to view their account balance, withdraw cash (i.e., take moneyout of an account)and depositfunds (i.e.,place moneyintoanaccount). Welcome! Please enter youraccountnumber:12345 Screen Enter yourPIN:54321 Take cash here Cash Dispenser Keypad InserInsertdtdeposit envelopelope here here Deposit Slot Fig.25.1 | Automatedtellermachineuser interface. Theuserinterfaceofthe automatedteller machinecontains thefollowing hardware components: •ascreen that displays messages to theuser © 2012 Pearson Education, Inc., Upper Saddle River, NJ. All Rights Reserved. 25-4 Chapter 25 ATMCaseStudy,Part1:Object-Oriented Design with theUML •akeypad that receives numeric inputfrom theuser •acash dispenser that dispenses cash to theuserand •adepositslotthatreceives depositenvelopes from theuser. Thecashdispenserbeginseachday loaded with 500$20 bills. [Note: Owingtothe limited scopeofthis case study, certainelements of theATM described here do notaccurately mimicthose of arealATM.For example, arealATM typicallycontains adevice that reads auser’s account number from an ATMcard, whereasthis ATMasksthe user to typean accountnumberusing thekeypad.Areal ATMalsousuallyprints areceipt at theend of asession, but alloutputfrom this ATMappears on thescreen.] Thebankwants youtodevelop softwaretoperform thefinancialtransactions initi- ated by bank customersthrough theATM. Thebankwillintegrate thesoftwarewiththe ATM’shardwareatalatertime. Thesoftwareshouldencapsulatethe functionalityofthe hardwaredevices(e.g.,cashdispenser,deposit slot)within software components,but it need notconcern itself with how thesedevicesperform theirduties.The ATMhardware hasnot been developedyet,soinstead of writing your softwaretorun on theATM,you should developafirstversion of thesoftwaretorun on apersonal computer. This version should usethe computer’smonitor to simulatethe ATM’sscreen,and the computer’s key- board to simulate theATM’s keypad. An ATMsession consists of authenticating auser(i.e., provingthe user’s identity) basedonanaccount number andpersonal identification number (PIN), followed by cre- ating andexecuting financial transactions. To authenticate auserand perform transac- tions, theATM must interact with thebank’saccount information database.[Note: A database is an organized collectionofdata storedonacomputer.]For each bank account, thedatabasestoresanaccountnumber,aPINand abalance indicating theamountof moneyinthe account.[Note: For simplicity, we assume that thebank planstobuild only oneATM, so we do notneedtoworry about multiple ATMs accessing this database at thesame time.Furthermore,weassumethatthe bank doesnot make any changes to theinformationin thedatabase while auserisaccessing theATM. Also,any business systemlikeanATM faces reasonably complicatedsecurityissuesthatgowell beyond the scopeofafirst- or second- semester computerscience course. We makethe simplifyingassumption,however,that thebanktruststhe ATMtoaccess andmanipulate theinformation in thedatabase without significant securitymeasures.] Upon firstapproachingthe ATM, theusershould experiencethe followingsequence of events (shown in Fig. 25.1): 1. Thescreen displaysawelcomemessage andprompts theusertoenteranaccount number. 2. Theuserenters afive-digit account number,using thekeypad. 3. Thescreen promptsthe user to enterthe PIN (personalidentification number) associatedwiththe specifiedaccount number. 4. Theuserenters afive-digit PIN, using the keypad. 5. If theuserenters avalid account number andthe correctPIN forthataccount, thescreen displaysthe mainmenu(Fig.25.2).Ifthe userenters an invalid ac- count number or an incorrect PIN, thescreen displaysanappropriate message, then theATM returnstoStep 1 to restartthe authentication process. © 2012 Pearson Education, Inc., Upper Saddle River, NJ. All Rights Reserved. 25.3 Examiningthe ATMRequirements Document 25-5 Main menu: 1-View my balance 2-Withdraw cash 3-Deposit funds 4-Exit Enter achoice: Take cash here InserInserttddepositeposit envelopelope here here Fig.25.2 | ATMmainmenu. Afterthe ATMauthenticatesthe user,the mainmenu(Fig.25.2) displaysanum- beredoption foreachofthe threetypes of transactions:balance inquiry (option 1),with- drawal (option 2) anddeposit (option 3).The main menu also displaysanoption that allows theusertoexitthe system (option 4).The user then chooses either to perform a transaction (byentering1,2or 3) or to exit thesystem(by entering4). If theuserenters an invalid option,the screen displaysanerror message,thenredisplays to
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages42 Page
-
File Size-