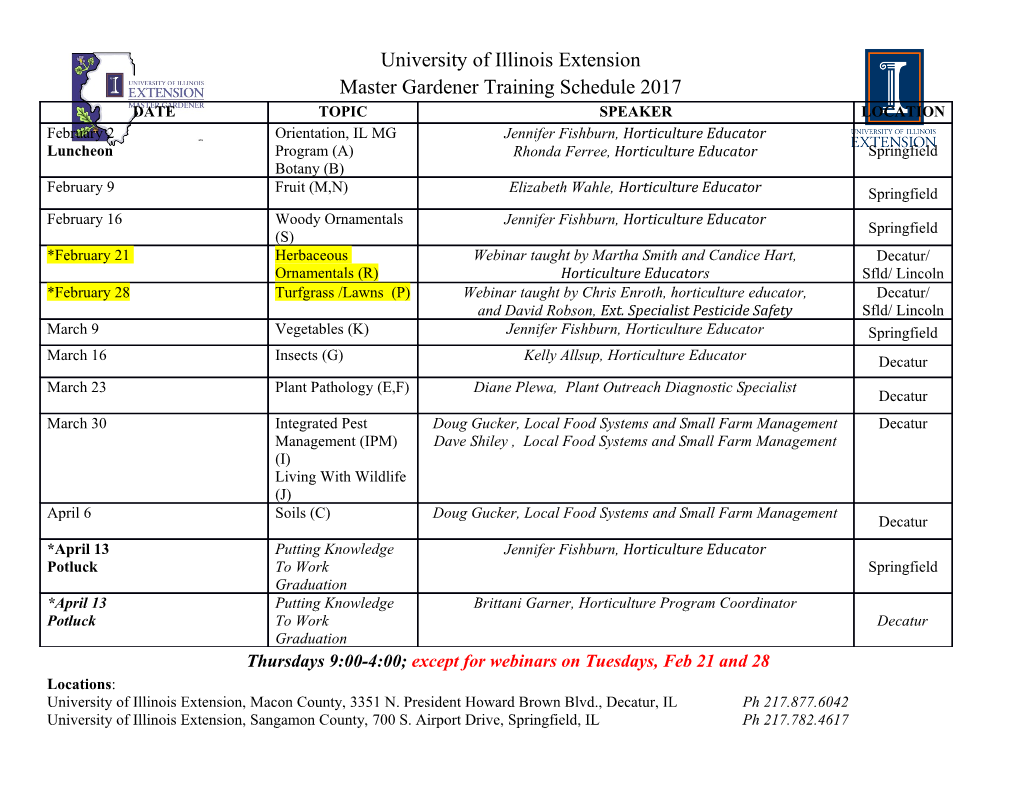
CS2361–Internet & Java 2.1 Introduction What is JavaScript? Discuss its features ¾ JavaScript is a client-side scripting language and is the defacto scripting language for most web-based application. ¾ It is used to enhance the functionality and appearance of Web pages by making it more dynamic, interactive and creating web pages on the fly. ¾ JavaScript is interpreted by the browser. ¾ Statements are terminated by a ; but is not required. ¾ JavaScript standard is defined by ECMA to which Netscape is fully compliant whereas IE complies with a modified version known as JScript. ¾ The <script> element introduces a client-side scripting language. ¾ It is generally placed within the <head> element, but can also be part of <body> o The type attribute specifies the MIME scripting language and its value is text/javascript for JavaScript. <html> <head> ... <script type="text/javascript"> <!-- Put your JavaScript code here // --> </script> </head> <body></body> </html> ¾ The script is executed either when page is loaded or when given event occurs. ¾ The script will not be executed if it is disabled in the browser environment. ¾ For browsers that do not understand javascript, the entire script code is enclosed within xhtml comment. ¾ Script can also be placed in a separate file and pointed by src attribute. ¾ JavaScript is an object-oriented language and has a set of pre-defined objects (document, window, form, etc) to access and manipulate XHTML elements. ¾ An object's attribute is used to set/read data, whereas a method provides service. ¾ Javascripthas utility classes such as date, math, string, etc which can be instantiated. List some common JavaScript objects and its usage? Object Description document Used to access XHTML body elements such as links, images, etc form It is used to access XHTML form elements in the current web page frame It corresponds to a frame in the window history Holds the history of sites previously visited location Holds information about the page URL navigator Refers to the browser itself window Refers to the current browser window http://cseannauniv.blogspot.in Vijai Anand CS2361–Internet & Java 2.2 List some methods of commonly used objects? ¾ document.writeʊwrites text to the current web page ¾ document.writelnʊwrites text to the current page and adds a newline character ¾ history.goʊnavigates the browser to a location in the browser history ¾ window.alertʊdisplays a message dialog ¾ window.promptʊdisplays an user input dialog ¾ window.openʊopens a new browser window ¾ window.closeʊCloses the current window List some properties of commonly used objects? ¾ document.linkcolorʊto set color of unvisited hyperlinks ¾ document.bgcolorʊto set background color of the current page ¾ document.lastmodifiedʊto know when the page was last modified ¾ document.titleʊtitle of the current page ¾ location.hostnameʊname of the ISP host ¾ navigator.appNameʊName of the browser. How are comments specified in JavaScript? ¾ A single line comment is placed after //. ¾ A multiline comment is placed within /* and */. List the rules for identifiers in JavaScript. ¾ Identifiers are declared using var statement and is loosely typed ¾ Identifier can contain alphabet, digits, underscore or dollar sign ¾ The first character must be an alphabet, underscore(_) or dollar sign ($) ¾ Identifiers are case sensitive and white spaces are not allowed ¾ Some valid identifiers are Sum, sum, total_marks, _valid, $value List the operators supported by JavaScript. ¾ Arithmetic: + - * / % ¾ Increment / Decrement operator: ++-- ¾ Combination assignment operators such as += *= /= etc ¾ Relational: <<= >>= == != ¾ ҏLogical: && || ! ¾ Bitwise operator: & | >><< ^etc ¾ Operator precedence are of order () ++ -- * / % + - <<= >>= == != ?: = += -= *= /= %= Give the syntax of Javascript's writeln method and explain with an example. document.writeln("string"); ¾ The document object represents the XHTML document currently displayed. ¾ The writeln method of document object instructs the browser to display the string and places cursor on the next line, whereas the write method displays further output on the same line. ¾ The + operator is used to concatenate strings. If string contains XHTML element or escape sequence, then it is interpreted by the browser accordingly. http://cseannauniv.blogspot.in Vijai Anand CS2361–Internet & Java 2.3 <html> <head> <title>writeln method</title> <script type="text/javascript"> <!-- document.writeln("<h1>Welcome to JavaScript</h1>" ); // --> </script> </head> <body></body> </html> List the escape sequences supported by JavaScript. ¾ The escape sequences are characters preceded by a \ (backslash) as in C. ¾ Used in output statement to provide special function. Commonly used ones are\n (newline) \t(tab) \" (quotes) \' (apostrophe), etc. How are variables declared in JavaScript? ¾ Variables in Javascript are declared using var keyword. ¾ Variables in Javascript are loosely typed, i.e., type of the variable is not specified in the declaration statement. Briefly explain prompt & alert dialog window with an example. window.alert("plaintext"); variable = window.prompt("plaintext", "default"); ¾ The prompt method of window object is used to display a user prompt dialog that displays a message and textbox for user input. ¾ An optional default value can be included. ¾ When user enters the value and presses OK, the value entered is stored in the variable as a string. ¾ If the user clicks Cancel or enters an incorrect type of data, error results. ¾ The alert method of window object is an information dialog used to display information to the user. It is closed by clicking the OK button. <html> <head> <title>An Addition Program</title> <script type="text/javascript"> <!-- varn1,n2,sum; n1 = parseInt(window.prompt("Enter first integer", "0")); n2 = parseInt(window.prompt("Enter second integer", "0")); sum = n1 + n2; window.alert( "The sum is " + sum); // --> </script> </head> <body></body> </html> http://cseannauniv.blogspot.in Vijai Anand CS2361–Internet & Java 2.4 Give the usage of parseInt function in JavaScript? ¾ The parseInt function is used to convert the string number to integer for processing. ¾ If the string is not a pure number, then NaN (Not a Number) error results. Control Structures – Selection Define algorithm. ¾ An algorithm is a step-by-step solution for a given problem. ¾ It is generally written in lay-man's language. Give the characteristics of a Pseudocode. ¾ Pseudocode is an artificial and informal language that helps programmers to develop algorithms. ¾ It is written in plain English, convenient and user friendly. ¾ It describes executable statements but not declarations. ¾ It is not a computer programming language. List some javascript keywords. Break case continue default while delete do else void for function if in var new return switch this typeof with Explain if statement(or) single selection statement in detail with an example. Syntax Flowchart if(condition) { statements } ¾ The if structure is a single-entry/single-exit structure. ¾ The if statement evaluates a condition (relational or logical expression), whose outcome is either true or false. ¾ If the condition is true, then next statement or statements enclosed within {} is executed otherwise not. <html> <head> <title>if statement</title> <script type="text/javascript"> <!-- var age; age = window.prompt("Enter your age"); if (parseInt(age) >= 18) document.writeln(age + " years is eligible to vote."); // --> http://cseannauniv.blogspot.in Vijai Anand CS2361–Internet & Java 2.5 </script> </head> <body></body> </html> Write if statement for the following: i. Display leap year if month is February and it has 29 days. ii. Display "Counter Closed" if it is non-banking hours if (day==29 && month=="February") document.writeln("Leap year"); if (hour < 8 || hour >17) document.writeln("Counter Closed"); Explain if-else statement(or) double selection in Javascript with an example Syntax FlowChart if(condition) { statements_1 } else { statements_2 } ¾ The if statement is extended to include an optional else part. ¾ The if-else statement is a two-way decision making. ¾ If the condition is true, then set of statements following if is executed, otherwise set of statements following else is executed. <html> <head> <title>if-else</title> <script type="text/javascript"> <!-- varnum; num = parseInt(window.prompt("Enter a number")); if (num%2 == 0) document.writeln("Given number " + num + " is Even"); else document.writeln("Given number " + num + " is Odd"); // --> </script> </head> <body></body> </html> What is ternary operator? Give an example. http://cseannauniv.blogspot.in Vijai Anand CS2361–Internet & Java 2.6 ¾ JavaScript provides a conditional operator known as ternary operator ?: ¾ Ternary operator provides a concise way of representing an if-else statement. Condition?statement1:statement2; ¾ If condition holds true, then statement1 is executed, else statement2 is executed. document.writeln(a>b ? "A is big" : "B is big" ); ¾ Ternary operators can be nested. Explain nested else-if (or) if-else structure with an example. ¾ Nested if-else structure checks multiple condition by placing an if-else as part of another if-else. ¾ The nested structure can contain an optional else part. ¾ Each boolean expression is evaluated in order. If true, then the corresponding sets of statements are executed and control is transferred out of the structure. ¾ When all conditions fail, then the last optional else part if present is executed. <html> <head> <title>else-ifladder</title> <script
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages26 Page
-
File Size-