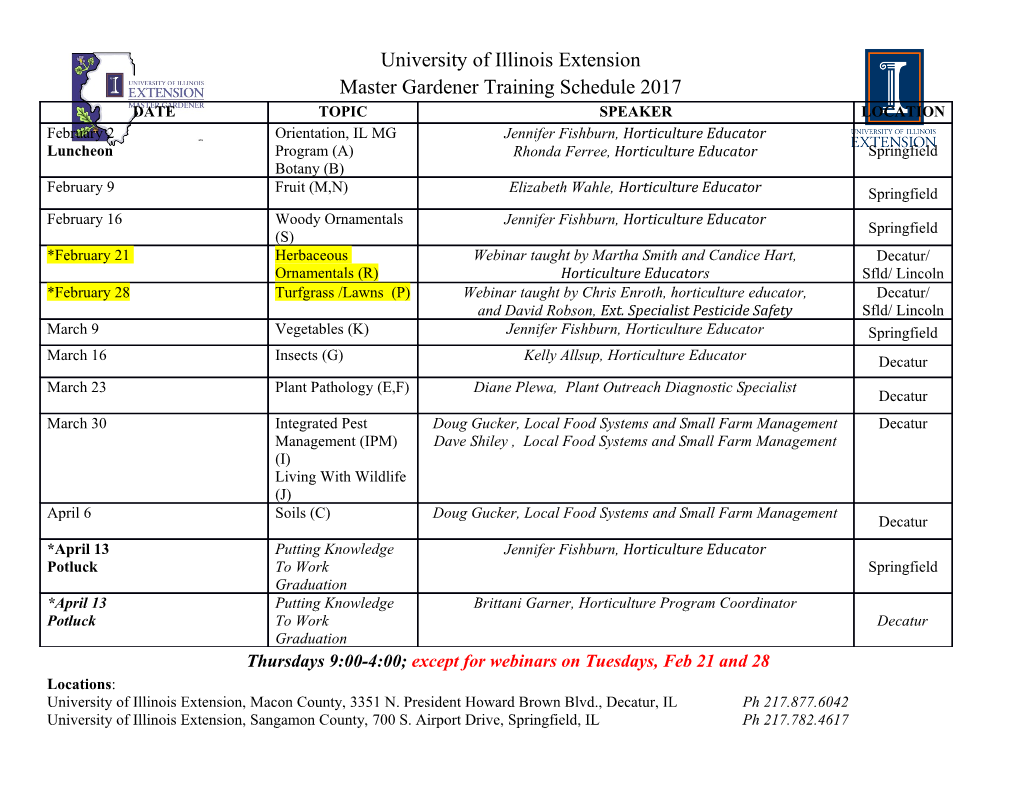
Object Oriented Perl Object Oriented Perl DAMIAN CONWAY MANNING Greenwich (74° w. long.) For electronic browsing and ordering of this and other Manning books, visit http://www.manning.com. The publisher offers discounts on this book when ordered in quantity. For more information, please contact: Special Sales Department Manning Publications Co. 32 Lafayette Place Fax: (203) 661-9018 Greenwich, CT 06830 email: [email protected] ©2000 by Manning Publications Co. All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or by means electronic, mechanical, photocopying, or otherwise, without prior written permission of the publisher. Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where those designations appear in the book, and Manning Publications was aware of a trademark claim, the designations have been printed in initial caps or all caps. Recognizing the importance of preserving what has been written, it is Manning’s policy to have the books we publish printed on acid-free paper, and we exert our best efforts to that end. Library of Congress Cataloging-in-Publication Data Conway, Damian, 1964- Object oriented Perl / Damian Conway. p. cm. includes bibliographical references. ISBN 1-884777-79-1 (alk. paper) 1. Object-oriented programming (Computer science) 2. Perl (Computer program language) I. Title. QA76.64.C639 1999 005.13'3--dc21 99-27793 CIP Manning Publications Co. Copyeditor: Adrianne Harun 32 Lafayette Place Typesetter: Tony Roberts Greenwich, CT 06830 Cover designer: Leslie Haimes Printed in the United States of America 1 2345678910– CM – 02 01 00 99 For Linda contents foreword xi preface xii acknowledgments xviii author online xx 1 What you need to know first (an object-orientation primer) 1 1.1 The essentials of object orientation 2 1.2 Other object-oriented concepts 13 1.3 Terminology: a few (too many) words 18 1.4 Where to find out more 18 1.5 Summary 20 2 What you need to know second (a Perl refresher) 21 2.1 Essential Perl 21 2.2 Non-essential (but very useful) Perl 51 2.3 The CPAN 65 2.4 Where to find out more 68 2.5 Summary 72 3 Getting started 73 3.1 Three little rules 73 3.2 A simple Perl class 80 3.3 Making life easier 89 3.4 The creation and destruction of objects 96 3.5 The CD::Music class, compleat 114 3.6 Summary 117 vii 4 Blessing arrays and scalars 118 4.1 What’s wrong with a hash? 118 4.2 Blessing an array 119 4.3 Blessing a pseudo-hash 126 4.4 Blessing a scalar 135 4.5 Summary 142 5 Blessing other things 143 5.1 Blessing a regular expression 143 5.2 Blessing a subroutine 151 5.3 Blessing a typeglob 158 5.4 Summary 166 6 Inheritance 168 6.1 How Perl handles inheritance 168 6.2 Tricks and traps 178 6.3 Example: Inheriting the CD class 193 6.4 Where to find out more 201 6.5 Summary 202 7 Polymorphism 203 7.1 Polymorphism in Perl 203 7.2 Example: Polymorphic methods for the Lexer class 205 7.3 The simple pretty-printer objectified 208 7.4 Using interface polymorphism instead 210 7.5 Where to find out more 212 7.6 Summary 212 8 Automating class creation 213 8.1 The Class::Struct module 213 8.2 The Class::MethodMaker module 222 8.3 Where to find out more 234 8.4 Summary 235 viii CONTENTS 9Ties236 9.1 A jacketing tie required 236 9.2 Tie-ing a scalar 238 9.3 Tie-ing a hash 243 9.4 Tie-ing an array 249 9.5 Tie-ing a filehandle 256 9.6 Inheriting from a tie’able package 262 9.7 Tied variables as objects 265 9.8 Where to find out more 274 9.9 Summary 275 10 Operator overloading 276 10.1 The problem 276 10.2 Perl’s operator overloading mechanism 278 10.3 Example: A Roman numerals class 284 10.4 Circumventing undesired reference semantics 291 10.5 The use and abuse of operators 292 10.6 Where to find out more 295 10.7 Summary 295 11 Encapsulation 296 11.1 The perils of trust 296 11.2 Encapsulation via closures 297 11.3 Encapsulation via scalars 302 11.4 Encapsulation via ties 309 11.5 Where to find out more 326 11.6 Summary 326 12 Genericity 327 12.1 Why Perl doesn’t need special generic mechanisms 327 12.2 Using specific mechanisms anyway 329 12.3 Implicit generics via polymorphism 336 12.4 Where to find out more 350 12.5 Summary 350 CONTENTS ix 13 Multiple dispatch 351 13.1 What is multiple dispatch? 351 13.2 Multiple dispatch via single dispatch and cases 353 13.3 Multiple dispatch via a table 356 13.4 Comparing the two approaches 361 13.5 Dynamic dispatch tables 363 13.6 Some lingering difficulties 367 13.7 The Class::Multimethods module 367 13.8 Comparing the three approaches 385 13.9 Where to find out more 385 13.10 Summary 385 14 Persistent objects 387 14.1 The ingredients 387 14.2 Object-oriented persistence 398 14.3 Coarse-grained persistence 400 14.4 Fine-grained persistence 412 14.5 Where to find out more 427 14.6 Summary 428 A Quick reference guide 429 B What you might know instead 438 B.1 Perl and Smalltalk 438 B.2 Perl and C++ 443 B.3 Perl and Java 449 B.4 Perl and Eiffel 454 glossary 459 bibliography 466 index 468 x CONTENTS foreword I’ve waited years for the perfect object-oriented Perl book to use for our Stonehenge corporate and open trainings, and the wait is now over. Damian Conway has written a comprehensive guide, organized well for both the casual OO hacker as well as the experienced OO user, includ- ing large reusable chunks of code (and that’s what OO is all about). Damian’s humor makes the reading light and fast. The depth of coverage from “what’s the big fuss about Perl objects?” to “creating a self-tied inheritable overloaded filehandle with auto- loaded accessors” means that this is the first and last book I need to teach Perl objects to my students. For experienced users, the appendix comparing and contrasting Perl with other popular OO languages is by itself worth the entire price of the book. I’ve been recommending this book heartily upon seeing the first draft. Thank you, Damian. RANDAL L. SCHWARTZ xi preface What’s this book about? This book is about the Laziness—on a grand scale. It’s about how to create bigger, more robust applications that require less effort to build, less time to debug, fewer resources to maintain, and less trouble to extend. Specifically, it’s about how to do all that with the object-oriented features of Perl—how those features work and how to make use of the many labor-saving techniques and “tricks” that they make possible. Presenting these new language features requires only a few chapters (specifically, chapters 3 to 6), but the range of programming styles and idioms they make available would fill several books. This book concentrates on the most useful and powerful ways to use object-oriented Perl. This book is also about the tremendous flexibility of Perl’s approach to object orientation, and how—in Perl—there’s almost always more than one object-oriented way to solve a given problem. You’ll find that the text revisits a few key examples time and again, extending and re- implementing them in various ways. Sometimes those changes will add extra functionality to a previous example; sometimes they’ll merely illustrate an alternative solution with different strengths and limitations. This book is about helping you to develop new Perl programming skills that scale. Perl is a great language for “one-line-stands”: ad hoc solutions that are quick, cryptic, and unstructured. But Perl can also be a great language for developing large and complex applications. The only problem is that “quick, cryptic, and unstructured” is cute in a throw-away script, but not so amus- ing in 5,000 or 50,000 lines of application code. Object-oriented programming techniques are in- valuable for building large, maintainable, reusable, and comprehensible systems in Perl. Finally, this book is about how Perl makes object-oriented programming more enjoyable and how object-oriented programming makes Perl more enjoyable too. Life is too short to endure the cultured bondage-and-discipline of Eiffel programming or wrestle the alligators that lurk in the muddy semantics of C++. Object-oriented Perl gives you all the power of those languages (and more!) with few of their tribulations. And, best of all, like regular Perl, it’s fun! Who’s this book for? This book was written for the whole Perl community. In other words, for an eclectic range of people of wildly differing backgrounds, interests, and levels of experience. xii To that end, it starts slowly, assuming only a basic familiarity with the core features of Perl itself: scalars, arrays and hashes, pattern matching, basic I/O, and simple subroutines. If these things sound familiar, this book is definitely for you. If they don’t, chapter 2 provides a quick re- fresher course in everything you’ll need to know. The only other assumption that’s made is that you’re interested in object orientation. Maybe you’ve only heard about its many advantages.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages510 Page
-
File Size-