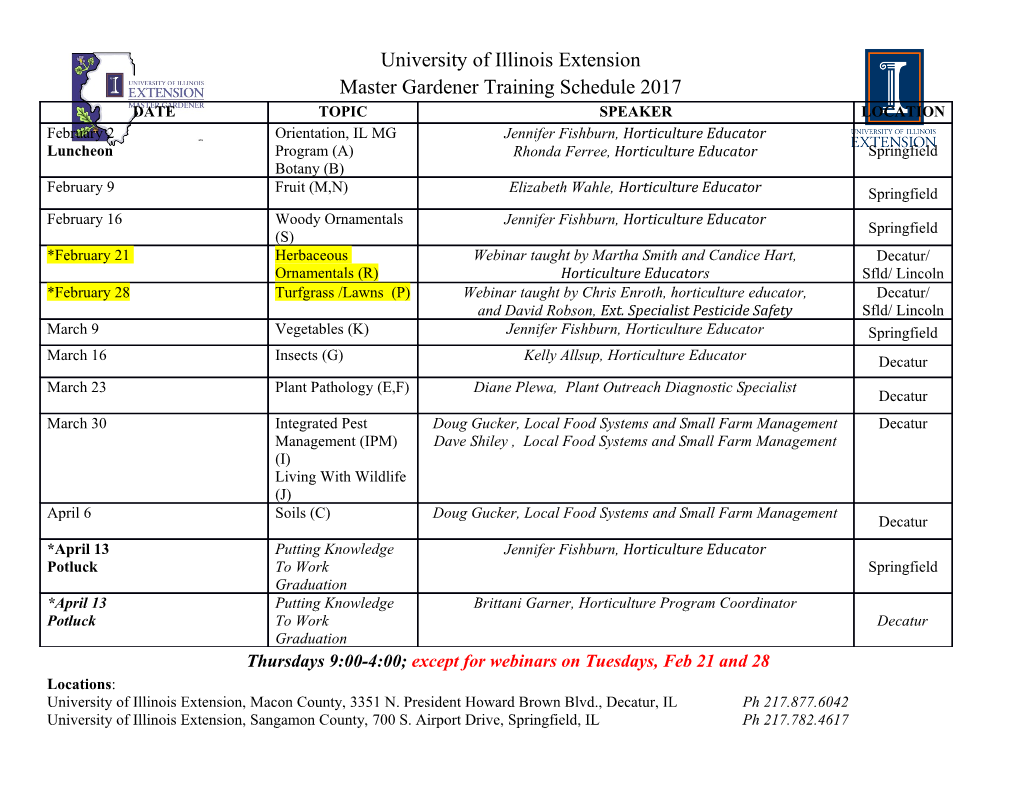
Designing Complex Systems Using SCL Data Objects Andrew A. Norton, Trilogy Consulting Corporation, Kalamazoo, Michigan INTRODUCTION Complexity: If you can change code easily. you can be"gin with a simple program and elaborate upon it in stages. This incremental development strategy is discussed further below. "Object-oriented ~ has become the buzzword of the moment. The recent marketing blitz has bleached it of all meaning, but Reuse: Reusing code in a different, unanticipated context the underlying concepts go back 25 years. Although these is a form of change. concepts developed incrementally from earlier software design principles, the end result is revolutionary -- once you have Transition from Analysis to Design: A well-established learned the new way of thinking, you can't go back. principle in software development is to separate analysis (determining what needs to be done) from design A complete object-oriented language is developing within the (determining how to do it). Object-oriented programming SAS* System. Many of the pieces are in place in releases 6.08 supports this principle by allowing design decisions to be and 6.10 of Screen Control Language (SCL). It is already postponed and revised. feasible to develop object-oriented systems in SAS just as yOll might in C++ or Smalltalk. This paper focuses on object~oriented strategies for managing change. Discussions of object-oriented programming in SAS often focus on small "toy" problems. Although this teChnique helps the Incremental Development novice to understand every aspect of the example, it gives a limited and artificial view of these powerful new features. This Traditional "waterfall" methodologies assume a predominantly paper is different. It focuses on how objects are used in one-way flow from analysis to design to implementation to constructing large-scale complex systems. I will not be testing. The analysis phase is completed before coding begins. spending much time discussing the nuts and bolts of how This requires committing to important decisions early, when you individual objects can be implemented in SAS. instead, I will have limited knowledge of their implications. be discussing what objects can do, and how they can be used in combination to tame complex and changing systems. Incremental development (Cockburn, 1995) strategies begin by developing a simplified prototype version. This prototype is Object-oriented programming is a natural progression from then incrementally enhanced based upon what has been learned traditional programming, so I will start there. Terms of special so far. This process can be repeated or "iterated" many times: significance are italicized the first time they appear. analyze-design-code-test, analyze-design-code-test. Object-oriented programming is especially suited to incremental WHAT'S SO GREAT ABOUT OO? development (and vice-versa) because it is easy to make changes. Because the penalty for design mistakes is relatively small, you :<f,an tackle complicated projects without having to Object-oriented programming (00) has become especiaUy understand everything at the beginning. popular for the development of Graphical User Interface (GUJ) applications. These applications can be very complex, because Avoiding "Ripple Effects" the user is given a great deal of freedom. Of course, batch processes can also be very complicated, and object-oriented SCL A small stone dropped into the water will affect the entire pond. can be helpful here as well. 1 In the same way, a small change in the specifications of a poorly designed system can require major efforts to implement. In general. object-oriented languages excel at complex tasks, The problem is ·"ripple effects"; local changes which require and are less effective for simple and repetitive tasks. changes in their neighbors (which then require changes in their neighbors). Advantages of 00 Laurence J. Peter (1986, page 155) says "The more complex Change is constant in the world of computer programming. the system, the more open it is to total breakdown." My because of improved understanding of the problem. changed defmition of total breakdown is a system which cannot be fixed requirements, debugging. and enhancements. Object-oriented because any ehange would break something else. progranuning helps you manage change, contributing to other advantages of object-oriented programming including control of To avoid "total breakdown", systems can be designed to be complexity, reuse of code, and smooth transition from analysis receptive to change. One way to do this is to construct systems to design: out of modular sections which can be changed without adversely affecting other modules. Such systems are also easier to 187 understand (Simon, 1981, page 219). This strategy for handling locate, revise, and test every use of the subroutine. This is a complex problems can be summed up by the phrase: disaster! Divide and Conquer. Suppose driver license policy changes: High school dropouts Subroutines are ineligible for a license until- age 18. The ELIGJBLE subroutine now requires another parameter HIGHSCHL The traditional way to "divide and conquer" computer programs (dropout status). Every program calling the ELIGIBLE has been through the use of subroutines (known in SAS as subroutine will need to be modified to read: "methods" and "macros".) Subroutines let applications share call method ('Driver' , 'eligible' , code. There is only one copy of the code to maintain, which age, drivtrn, highschl, ok); Some of the programs that call the ELIGffiLE program may ensures consistency, saves effort, improves quality, and themselves need to be modified to obtain the IDGHSCHL minimizes testing. infonnation. Subroutines also protect applications from changes in the Unfortunately, subroutine signatures often need to be modified subroutine code. When an application calls a subroutine, the to handle new siruations or make additional distinctions. If a two programs communicate through a limited and well-defined modified subroutine requires additional data, the signature will set of variables called parameters. need to be changed accordingly. Remember: Exposed data details lead to ripple effects. As long as the subroutine returns the correct output for the provided input data, then the details of implementation on either Luckily there is a solution, and this solution sets us on the path side (calling program or called subroutine) are irrelevant. What towards object-oriemed programming. the subroutine does (the external interface) has -been separated from how the subroutine works (the internal implementation). The Solution There is a natural division of responsibilities: the subroutine knows what to do with the input and the caller knows what to We need a way to pass additional information to a subroutine do with the results. without modifYing the signature. This can be done if a parameter contains a bundle of data rather than an individuat The use of subroutines simplifies testing because the behavior of variable. In some languages this can be done with a compound each subroutine is dependent upon its parameters alone and does data structure. In Screen Control Language, you can use an not change from one context to another. Similarly, maintenance SCL list (SAS Institute, 1991). If more data elements are becomes much easier: Once you have located and corrected the needed by the subroutine, just add them to the list. For appropriate subroutine, you are done. example, assuming an SCL list DRIVER! contains elements named SEX, AGE, and COUNTRY for a certain automobile In Screen Control Language, a typical subroutine call might be: driver, we could have: call method ('Oriver','eligible', age, drivtrn, ok); call method ('Driver','eligible', The first argument identifies the SCL entry containing the driver1, ok); method code: DRIVER.SCL. The second argument identifies No harm is done if the list includes additional information not the labeled block of code to be executed: ELIGIBLE .. The used by the subroutine. Because a variety of subroutines will remaining parameters (if any) are defmed by the programmer. need some data about drivers, we could maintain one list for In this case there are two input parameters, AGE and each driver containing all attributes of that driver. Such a list DRIVTRN (driver training status), and one output parameter could be used by any subroutine needing data about that driver: OK (eligibility for a driver's license). Because subroutines call method ('Driver' , Irenew', contain no persistent data, we know that the result OK is solely driver1, result); determined by the ELIGIBLE algorithm using the parameters AGE and DRIVTRN. The SeL lists for different drivers may contain different elements. The SCL list DRIVER1 might refer to a suspended This technology has been well-established for many years, and driver and contain different elements than SCL lists DRIVER2 you might not see any flaws with it until a bener way has been and DRIVER3 which refer to drivers with active licenses. described. The Problem Encapsulation If you write a really good subroutine, it might get used over and I have been emphasizing the importance of separating internal over again, in many different contexts. It might be used years implementations from external interfaces. Code is hidden within after you wrote it, or by people you have never met. This is a subroutine, and data parameters are hidden within a data list. success! Both are intimately related: Changes to data and code occur together. So instead of considering
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages8 Page
-
File Size-