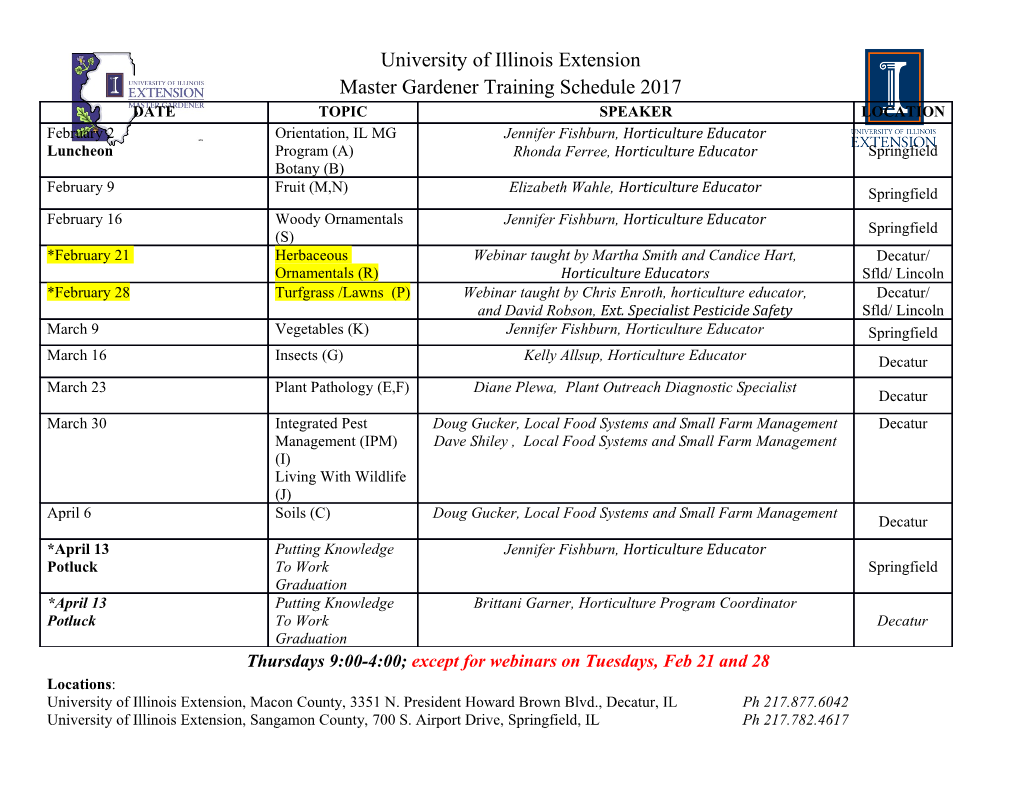
"Better than a thousand days of diligent study is one day with a Great Teacher" Java Question Bank (Version 10.0) www.techsparx.net www.techsparx.webs.com Saravanan.G Contents Most Simplest ............................................................................................................................................... 2 Decision Making Statements ........................................................................................................................ 4 Switch Statement .......................................................................................................................................... 7 Menu Driven Program................................................................................................................................... 7 Bill Programs ................................................................................................................................................. 8 Looping constructs (for, while, do while) ..................................................................................................... 9 Numbers – for loop ..................................................................................................................................... 10 Numbers – while loop ................................................................................................................................. 11 Series – nth term .......................................................................................................................................... 15 Series – n terms........................................................................................................................................... 16 Patterns ....................................................................................................................................................... 17 One Dimensional Array ............................................................................................................................... 19 Searching and Sorting ................................................................................................................................. 20 Strings – Part 1 ............................................................................................................................................ 21 Strings – Part 2 ............................................................................................................................................ 22 Strings – Part 3 ............................................................................................................................................ 23 Functions ..................................................................................................................................................... 27 Classes, Objects, Functions and Constructors ............................................................................................ 28 1 TechSparx Computer Training Center - 9880 205065 Most Simplest 1. Write a program (WAP) to print “Hello World”. 2. Write a program to print your name. 3. Write a program to add two numbers and display the given numbers and the sum. 4. Write a program to find the difference of two numbers and display the given numbers and the difference. 5. Write a program to find the product of two numbers and display the given numbers and the product. 6. Write a program to find the quotient of two numbers and display the given numbers and the quotient. 7. Write a program to find the remainder of two numbers and display the given numbers and the remainder. 8. Write a program to find the sum, difference, product, quotient and remainder of two numbers. 9. Write a program to find area of a square. 10. Write a program to find area of a rectangle. 11. Write a program to find the area of a circle. 12. Write a program to find the area of a triangle whose base and height are given. 13. Write a program to find area of a triangle whose 3 sides are given. 14. Write a program to find the volume and surface area of a sphere. 15. Write a program to convert Fahrenheit to Celsius 16. Write a program to convert Celsius to Fahrenheit. 17. Write a program to calculate the simple interest. 18. Write a program to calculate the compound interest. 19. Write a program to calculate the nth repunit. 20. Write a program to calculate the nth mersenne number. 21. Write a program swap two variables. 22. Write a program to find the roots of a quadratic equation. 2 TechSparx Computer Training Center - 9880 205065 Important formulas: Name Mathematical Formula Java Expression 1. Area of a Square double area = side * side; 2. Area of a Rectangle double area = length * breadth; 3. Area of a circle double area = Math.PI * radius * radius; 4. Area of a triangle double area = (1.0/2.0) * breadth * height; √ ( )( )( ) double s = (a * b * c) / 2.0; 5. Area of a triangle where double area = Math.sqrt( s * (s-a) * (s-b) * (s-c) ); double volume = (4.0 / 3.0) * 3.14 * r * r * r; or 6. Volume of a sphere double volume = (4.0 / 3.0) * Math.PI * r * r * r; or double volume = (4.0 / 3.0) * Math.PI * Math.pow(r, 3); Surface area of a 7. double surfaceArea = 4.0 * Math.PI * r * r; sphere double x = (-b + Math.sqrt( (b * b) – (4 * a * c) ) / ( 2 * a) ; Roots of quadratic √ 8. equation double x = (-b - Math.sqrt( (b * b) – (4 * a * c) ) / ( 2 * a) ; 9. Simple Interest double si = (p * t * r) / 100.0; 10. Compound Interest {( ) } ci = p * ( Math.pow( (1 + (r/100.0)), t ) - 1 ) 11. Repunit int repunit = ((int)Math.pow(10, n) – 1) / 9 12. Mersenne Number int mersenne = (int)Math.pow(2, n) – 1 Celsius to 13. ( ) double f = ( c * (9.0/5.0) ) + 32.0; Fahrenheit Fahrenheit to 14. ( ) ( ) double c = ( f – 32.0 ) * (5.0/9.0); Celsius 3 TechSparx Computer Training Center - 9880 205065 Decision Making Statements Using if 1. Write a program to find the larger of two given numbers. 2. Write a program to find the smaller of two given numbers. 3. Write a program to find if the given number is an even or odd number. 4. Write a program to check if the given number is Divisible by 7 (seven). 5. Write a program to check if the given number is positive or negative. Using if … else 6. Write a program to find the larger of two given numbers. 7. Write a program to find the smaller of two given numbers. 8. Write a program to find if the given number is an even or odd number. 9. Write a program to check if the given number is Divisible by 7 (seven). 10. Write a program to check if the given number is positive or negative. Using if … else if 11. Write a program to find the largest of three given numbers. 12. Write a program to find the smallest of three given numbers. 13. Write a program to find the real roots of a quadratic equation. (ax2 + bx + c = 0) 14. Write a program to print the grade based on the marks obtained according to the following table Marks Grade >=80 A 60 to 80 B 50 to 60 C 40 to 50 D <40 E 4 TechSparx Computer Training Center - 9880 205065 15. Write a program to print the day of week. (using if … else if ladder) Example: day = 1 dayOfWeek = “Monday” day = 2 dayOfWeek = “Tuesday” 16. Write a program to print the month of the year. (using if … else if ladder) Example: month = 1 --> monthOfYear = "January" month = 2 --> monthOfYear = "February" 17. Write a program to check if the given character is a digit or upper case letter or a lower case letter or a special character. 18. Write a program to check if the given character is a digit or upper case letter or a lower case letter or a special character. (using Character class functions) 19. Write a program to check if the given character is a vowel. 20. Write a program to check if the given number is a buzz number. Buzz number: A number which is either divisible by 7 or ends with 7. For Example: 67, 49, 37, 21 etc 21. Write a program to check if the given number is a Perfect square. For Example: 25, 36, 49 etc 5 TechSparx Computer Training Center - 9880 205065 6 TechSparx Computer Training Center - 9880 205065 Switch Statement 1. Write a program to input number of week’s day (1-7) and translate to its equivalent name of the day of the week. Example: 1 to Monday, 2 to Tuesday… 7 to Sunday 2. Write a program to input number of month of the year (1 - 12) and translate to its equivalent name of the month. Example: 1 to January, 2 to February … 12 to December 3. Write a program to check if the given character is a vowel. Menu Driven Program 1. Using a switch statement, write a MENU driven program to find the sum, difference, product quotient and remainder. OR Write a program to simulate a SIMPLE CALCULATOR. 2. Using a switch statement, write a MENU driven program to convert a given temperature from Fahrenheit to Celsius and vice versa. For an incorrect choice, an appropriate error message should be displayed. 3. Using a switch statement, write a MENU driven program to calculate the AREA of square, rectangle, triangle and circle. 7 TechSparx Computer Training Center - 9880 205065 Bill Programs 1. Design a class to enter the monthly salary of a person, calculate and print the annual tax payable by that person. Annual Salary (Rs.) Tax Payable Below 50,000 5% of annual salary 50,001 to 1,25,000 10% of annual salary Above 1,25,001 20% of annual salary 2. Define a class Electricity a. Accept Name, Consumer Number and Units consumed. b. Compute the electricity charges accordingly upto 100 units : 80 paise per unit for next 100 units : Rs. 1 per unit for more than 200 units : Rs. 1.25 per unit c. Display the name, consumer
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages32 Page
-
File Size-