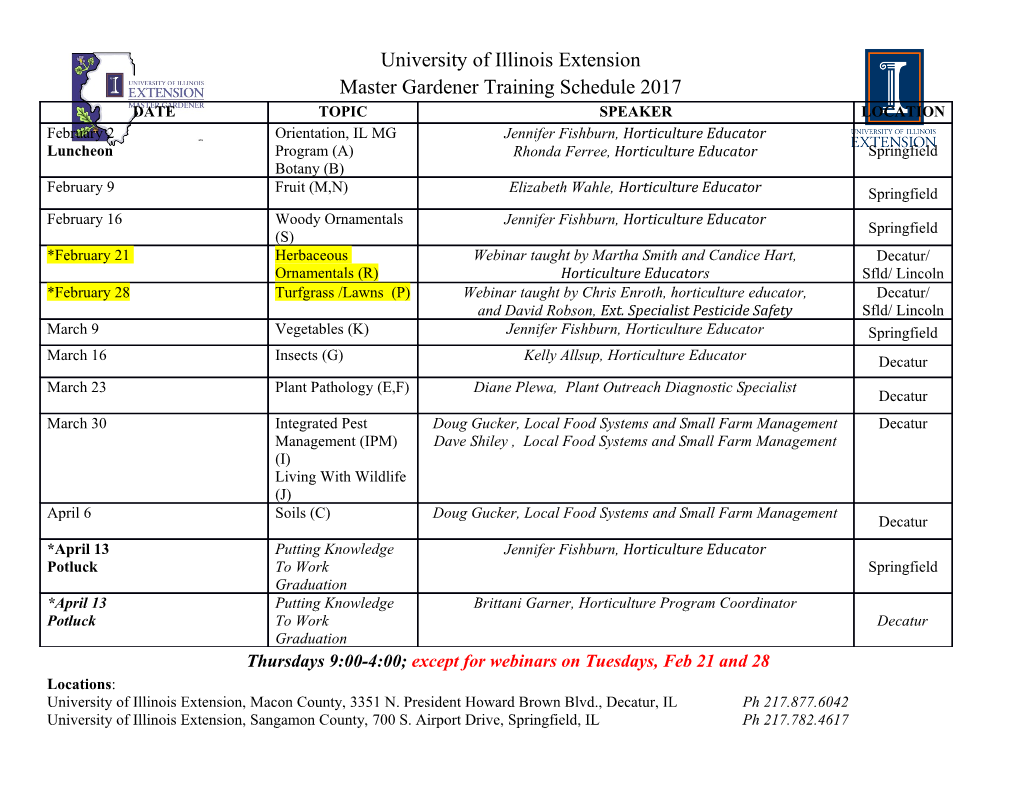
Compaq Extended Math Library Reference Guide January 2001 This document describes the Compaq® Extended Math Library (CXML). CXML is a set of high-performance mathematical routines designed for use in numerically intensive scientific and engineering applications. This document is a guide to using CXML and provides reference information about CXML routines. Revision/Update Information: This document has been revised for this release of CXML. Compaq Computer Corporation Houston, Texas © 2001 Compaq Computer Corporation Compaq, the COMPAQ logo, DEC, DIGITAL, VAX, and VMS are registered in the U.S. Patent and Trademark Office. Alpha, Tru64, DEC Fortran, OpenVMS, and VAX FORTRAN are trademarks of Compaq Information Technologies, L.P. in the United States and other countries. Adobe, Adobe Acrobat, and POSTSCRIPT are registered trademarks of Adobe Systems Incorporated. CRAY is a registered trademark of Cray Research, Incorporated. IBM is a registered trademark of International Business Machines Corporation. IEEE is a registered trademark of the Institute of Electrical and Electronics Engineers Inc. IMSL and Visual Numerics are registered trademarks of Visual Numerics, Inc. Intel and Pentium are trademarks of Intel Corporation. KAP is a registered trademark of Kuck and Associates, Inc. Linux is a registered trademark of Linus Torvalds. Microsoft, Windows, and Windows NT are either trademarks or registered trademarks of Microsoft Corporation in the United States and other countries. OpenMP and the OpenMP logo are trademarks of OpenMP Architecture Review Board. SUN, SUN Microsystems, and Java are registered trademarks of Sun Microsystems, Inc. UNIX, Motif, OSF, OSF/1, OSF/Motif, and The Open Group are trademarks of The Open Group. All other trademarks and registered trademarks are the property of their respective holders. All other product names mentioned herein may be the trademarks or registered trademarks of their respective companies. Confidential computer software. Valid license from Compaq required for possession, use or copying. Consistent with FAR 12.211 and 12.212, Commercial Computer Software, Computer Software Documentation, and Technical Data for Commercial Items are licensed to the U.S. Government under vendor’s standard commercial license. Compaq shall not be liable for technical or editorial errors or omissions contained herein. The information in this document is provided ‘‘as is’’ without warranty of any kind and is subject to change without notice. The warranties for Compaq products are set forth in the express limited warranty statements accompanying such products. Nothing herein should be construed as constituting an additional warranty. CXML documention is available on CD-ROM. This document prepared using DECdocument, Version 3.3-1b. Contents Preface ............................................................ xix Introduction to CXML 1 Parallel Library Support for Symmetric Multiprocessing .............. 2 2 Cray SciLib Support (SCIPORT) ................................ 2 3 Calling CXML from Programming Languages ...................... 2 4 How CXML Achieves High Performance . ....................... 3 5 CXML’s Accuracy ............................................ 3 Part 1—Programming Considerations 1 Preparing and Storing Program Data 1.1 Data and Data Types . ........................................ 1–1 1.2 Platforms and Number Formats ................................ 1–2 1.3 Storing Data ............................................... 1–3 1.3.1 Arrays . ................................................ 1–3 1.3.1.1 One-dimensional arrays . ................................ 1–3 1.3.1.2 Two-dimensional Arrays . ................................ 1–3 1.3.1.3 Storing Values in an Array .............................. 1–3 1.3.1.4 Array Storage Requirements ............................. 1–4 1.3.2 Fortran Arrays . ........................................ 1–4 1.3.2.1 One-Dimensional Fortran Array Storage .................... 1–4 1.3.2.2 Two-Dimensional Fortran Array Storage .................... 1–4 1.3.2.3 Array Elements ....................................... 1–5 1.3.3 Vectors . ................................................ 1–5 1.3.3.1 Transpose and Conjugate Transpose of a Vector ............... 1–6 1.3.3.2 Defining a Vector in an Array ............................ 1–6 1.3.3.2.1 Vector Length ...................................... 1–7 1.3.3.2.2 Vector Location .................................... 1–7 1.3.3.2.3 Stride of a Vector . ................................ 1–7 1.3.3.2.4 Selecting Vector Elements from an Array . ............... 1–8 1.3.3.3 Storing a Vector in an Array ............................. 1–9 1.3.4 Matrices ................................................ 1–10 1.3.4.1 Transpose and Conjugate Transpose of a Matrix .............. 1–11 1.3.4.2 Storing a Matrix in an Array ............................. 1–11 1.3.4.3 Defining a Matrix in an Array ............................ 1–12 1.3.4.3.1 Matrix Location .................................... 1–12 1.3.4.3.2 First Dimension of the Array . ....................... 1–12 1.3.4.3.3 Number of Rows and Columns of the Matrix .............. 1–13 1.3.4.3.4 Selecting Matrix Elements from an Array ............... 1–13 1.3.4.4 Symmetric and Hermitian Matrices . ....................... 1–13 1.3.4.5 Storage of Symmetric and Hermitian Matrices ............... 1–14 iii 1.3.4.5.1 Two-Dimensional Upper- or Lower-Triangular Storage . 1–14 1.3.4.5.2 One-Dimensional Packed Storage . ................... 1–15 1.3.4.6 Triangular Matrices .................................... 1–17 1.3.4.7 Storage of Triangular Matrices ........................... 1–17 1.3.4.8 General Band Matrices ................................. 1–17 1.3.4.9 Storage of General Band Matrices ......................... 1–18 1.3.4.10 Real Symmetric Band Matrices and Complex Hermitian Band Matrices . ............................................ 1–19 1.3.4.11 Storage of Real Symmetric Band Matrices or Complex Hermitian Band Matrices ........................................ 1–20 1.3.4.12 Upper- and Lower-Triangular Band Matrices ................ 1–21 1.3.4.13 Storage of Upper- and Lower-Triangular Band Matrices ........ 1–22 2 Coding an Application Program 2.1 Selecting the Appropriate Data Type . ............................ 2–1 2.2 Data Structure and Storage Methods . ............................ 2–1 2.3 Improving Performance . .................................... 2–1 2.4 Calling Sequences ........................................... 2–2 2.4.1 Passing of Arguments . .................................... 2–3 2.4.2 Implicit and Explicit Arguments . ............................ 2–3 2.4.3 Expanding Argument Lists ................................. 2–3 2.5 Calling Subroutines and Functions in Fortran . ................... 2–4 2.5.1 Fortran Program Example .................................. 2–5 2.6 Using CXML from Non-Fortran Programming Languages . ........... 2–5 2.6.1 Calling CXML from C Programs . ............................ 2–6 2.6.2 C Program Example . .................................... 2–6 2.7 Error Handling . ............................................ 2–7 2.7.1 Internal Exceptions . .................................... 2–8 3 Compiling and Linking an Application Program 3.1 Tru64 UNIX Platform ........................................ 3–1 3.1.1 CXML Libraries .......................................... 3–1 3.1.2 Compiling and Linking to the Serial Library . ................... 3–1 3.1.3 Compiling and Linking to the Parallel Library .................. 3–2 3.1.4 Compiling and Linking to the Archive Library .................. 3–2 3.2 Windows NT Platform ........................................ 3–2 3.2.1 CXML Libraries .......................................... 3–2 3.2.2 Using the Libraries from the Command Console ................. 3–2 3.2.3 Using the Libraries from Developer Studio . ................... 3–3 3.3 OpenVMS Alpha Platform . .................................... 3–3 3.3.1 Compiling . ............................................ 3–3 3.3.2 CXML Image Libraries .................................... 3–4 3.3.3 Linking to a CXML Library ................................. 3–4 3.3.4 Linking Errors ........................................... 3–5 iv Part 2—Using CXML Subprograms 4 Using the Level 1 BLAS Subprograms and Extensions 4.1 Level 1 BLAS Operations ...................................... 4–1 4.2 Vector Storage .............................................. 4–1 4.3 Naming Conventions . ........................................ 4–2 4.4 Summary of Level 1 BLAS Subprograms . ....................... 4–2 4.5 Calling Subprograms . ........................................ 4–8 4.6 Argument Conventions ........................................ 4–8 4.7 Error Handling ............................................. 4–8 4.8 Definition of Absolute Value .................................... 4–9 4.9 A Look at a Level 1 Extensions Subprogram ....................... 4–9 5 Using the Sparse Level 1 BLAS Subprograms 5.1 Sparse Level 1 BLAS Operations ................................ 5–1 5.2 Sparse Vector Storage ........................................ 5–2 5.2.1 Sparse Vectors . ........................................ 5–2 5.2.2 Storing a Sparse Vector .................................... 5–3 5.3 Naming Conventions . ........................................ 5–3 5.4 Summary of Sparse Level 1 BLAS Subprograms .................... 5–4 5.5 Calling Subprograms . ........................................ 5–6 5.6 Argument Conventions .......................................
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages584 Page
-
File Size-