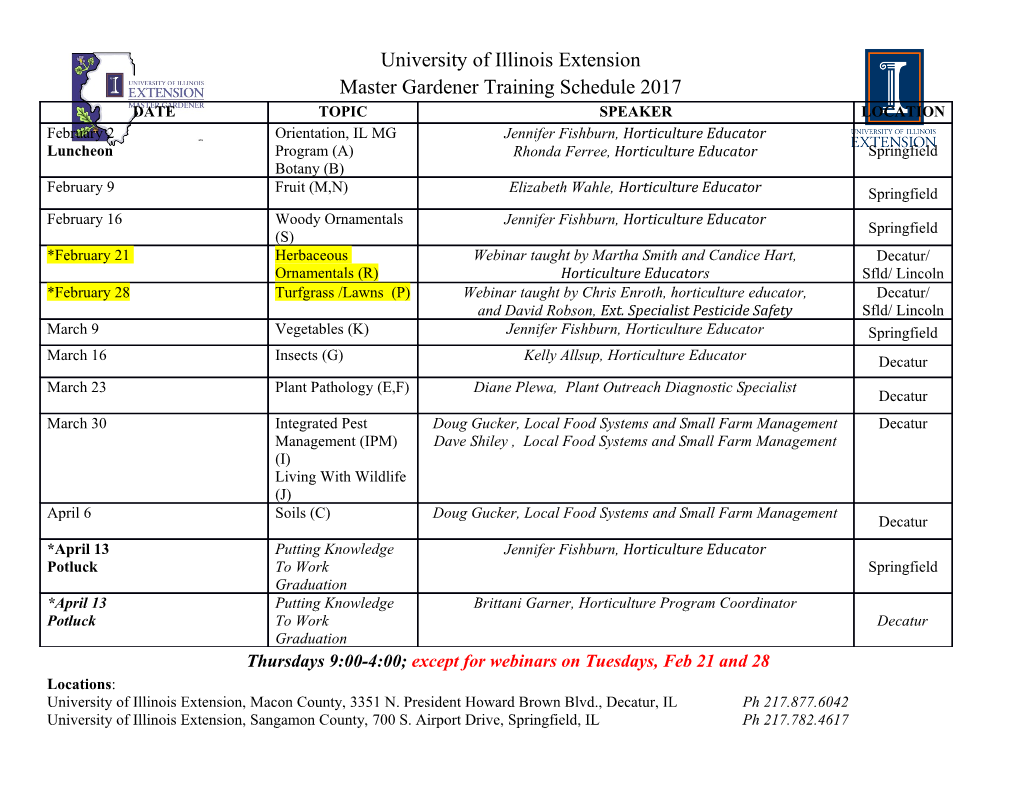
Gary Michael Wassermann September 2008 Computer Science Techniques and Tools for Engineering Secure Web Applications Abstract With the rise of the Internet, web applications, such as online banking and web-based email, have become integral to many people’s daily lives. Web applications have brought with them new classes of computer security vulnerabilities, such as SQL injection and cross-site scripting (XSS), that in recent years have exceeded previously prominent vulner- ability classes, such as buffer overflows, in both reports of new vulnerabilities and reports of exploits. SQL injection and XSS are both instances of the broader class of input validation- based vulnerabilities. At their core, both involve one system receiving, transforming, and constructing string values, some of which come from untrusted sources, and presenting those values to another system that interprets them as programs or program fragments. These input validation-based vulnerabilities therefore require fundamentally new techniques to characterize and mitigate them. This dissertation addresses input validation-based vulnerabilities that arise in the con- text of web applications, or more generally, in the context of metaprogramming. This dissertation provides the first principled characteriztion, based on concepts from program- ming languages and compilers, for such vulnerabilities, with formal definitions for SQL injection and XSS in particular. Building on this characterization, the dissertation also contributes practical algorithms for runtime protection, static analysis, and testing-based analysis of web applications to identify vulnerabilities in application code and prevent at- tackers from exploiting them. This dissertation additionally reports on implementations of these algorithms, showing them to be effective for their respective settings. They have low runtime overhead, validate the definitions, scale to large code bases, have low false-positive rates, handle real-world application code, and find previously unreported vulnerabilities. Techniques and Tools for Engineering Secure Web Applications By Gary Michael Wassermann B.S. (University of California, Davis) 2002 DISSERTATION Submitted in partial satisfaction of the requirements for the degree of DOCTOR OF PHILOSOPHY in COMPUTER SCIENCE in the OFFICE OF GRADUATE STUDIES of the UNIVERSITY OF CALIFORNIA DAVIS Approved: Professor Zhendong Su (Chair) Professor Premkumar T. Devanbu Professor Ronald Olsson Committee in Charge 2008 i c Gary Wassermann, 2008. All rights reserved. To my Lord and Savior, Jesus Christ and to my wife, Jaci, who has been a help and a joy to me. ii iii Contents 1 Introduction 1 1.1 WebApplications................................. 1 1.2 Input Validation-Based Vulnerabilities . ......... 2 1.3 CommonDefenses ................................ 6 1.4 DissertationStructure . ... 7 2 SQL Injection: Characterization and Runtime Checking 9 2.1 Introduction.................................... 9 2.2 OverviewofApproach .............................. 11 2.3 FormalDescriptions .............................. 14 2.3.1 Problem Formalization . 14 2.3.2 Algorithm for Runtime Enforcement . 18 2.3.3 CorrectnessandComplexity. 22 2.4 Applications.................................... 23 2.4.1 CrossSiteScripting ........................... 23 2.4.2 XPathInjection ............................. 24 2.4.3 ShellInjection .............................. 25 2.5 Implementation.................................. 25 2.6 Evaluation..................................... 26 2.6.1 EvaluationSetup............................. 27 2.6.2 Results .................................. 29 2.6.3 Discussions ................................ 30 2.7 RelatedWork................................... 31 2.7.1 InputFilteringTechniques. 31 2.7.2 Syntactic Structure Enforcement . 31 2.7.3 RuntimeEnforcement . .. .. .. .. .. .. .. 32 2.7.4 Meta-Programming............................ 34 3 Static Analysis for SQL Injection 36 3.1 Introduction.................................... 36 3.2 Overview ..................................... 38 3.2.1 ExampleVulnerability . 38 3.2.2 AnalysisOverview ............................ 40 3.3 AnalysisAlgorithm............................... 42 3.3.1 String-TaintAnalysis . 42 3.3.2 Policy-Conformance Analysis . 48 3.3.3 Soundness................................. 51 3.4 Implementation.................................. 51 3.5 Evaluation..................................... 52 3.5.1 TestSubjects ............................... 53 3.5.2 AccuracyandBugReports . 54 3.5.3 Scalability and Performance . 55 3.6 RelatedWork................................... 57 3.6.1 StaticStringAnalysis . 57 3.6.2 StaticTaintChecking .......................... 58 4 Static Analysis for Detecting XSS Vulnerabilities 60 4.1 CausesofXSSVulnerabilities . 60 4.2 Overview ..................................... 62 4.2.1 CurrentPractice ............................. 62 4.2.2 OurApproach .............................. 62 4.2.3 RunningExample ............................ 64 4.3 AnalysisAlgorithm............................... 64 4.3.1 String-taintAnalysis . 65 4.3.2 Preventing Untrusted Script . 67 4.4 EmpiricalEvaluation. 71 4.4.1 Implementation.............................. 71 4.4.2 TestSubjects ............................... 71 4.4.3 EvaluationResults ............................ 73 4.4.4 CurrentLimitations ........................... 78 4.5 RelatedWork................................... 79 4.5.1 Server-Side Validation . 79 4.5.2 Client-Side Mitigation . 80 5 Concolic Testing of Web Applications 83 5.1 Introduction.................................... 83 5.2 Overview ..................................... 86 5.2.1 ExampleCode .............................. 87 5.2.2 ConstraintGeneration . 87 5.2.3 ConstraintResolution . 89 5.2.4 TestOracles................................ 90 5.2.5 Selective Constraint Generation . 92 5.3 Algorithm..................................... 93 5.4 Evaluation..................................... 101 5.4.1 Implementation.............................. 101 5.4.2 TestSubjects ............................... 102 5.4.3 Evaluation ................................ 104 5.5 Limitations .................................... 106 5.6 RelatedWork................................... 107 iv 5.6.1 TestInputGeneration . 107 5.6.2 Web Application Testing . 108 5.6.3 Static Analysis of PHP Web Applications . 108 6 Conclusion 110 6.1 Summary ..................................... 110 6.2 ExtensionsandEnhancements . 112 6.3 Outlook ...................................... 113 v vi List of Figures 1.1 Web application system architecture. ....... 2 1.2 Recent vulnerability and attack numbers. ....... 3 1.3 Resultsofrecentattacks. ... 4 2.1 A JSP page for retrieving credit card numbers. ....... 11 2.2 System architecture of SqlCheck........................ 13 2.3 Parse trees for generated queries. ...... 16 2.4 Simplified grammar for the SELECT statement.................. 20 2.5 Exampleaugmentedgrammar. 21 2.6 Parse tree fragments for an augmented query. ....... 21 3.1 ExamplecodewithanSQLCIV. 39 3.2 SQLCIVanalysisworkflow. 40 3.3 Exampleintermediategrammar. 41 3.4 Grammarreflectsdataflow. 43 3.5 Examplefinitestatetransducer.. ..... 44 3.6 Taint propagation in CFG-FSA intersection. ........ 46 3.7 Semantics of explode............................... 47 3.8 Sourceofafalsepositive. 53 3.9 Source of an indirect errorreport......................... 54 4.1 VulnerablePHPcode. .............................. 63 4.2 CodeinSSAform. ................................ 65 4.3 ProductionsforextendedCFG. 65 4.4 Example intermediate grammar for XSS analysis. ........ 66 4.5 Clientarchitecture.. 68 4.6 Transducerwithuntrusteddata. 70 4.7 A vulnerability in Claroline 1.5.3. ....... 75 5.1 ExamplePHPcode. ............................... 86 5.2 A transducer for concatenation. ..... 89 5.3 An automaton and its image over a transducer. ...... 89 5.4 Expressionlanguage. .. .. .. .. .. .. .. .. 93 5.5 Algorithm to construct sets of single variable-occurrence expressions. 94 5.6 Constraintlanguage. .. .. .. .. .. .. .. .. 95 5.7 Type conversion from Boolean in PHP. 97 5.8 Algorithm for converting arbitrary constraints to Boolean constraints. 97 5.9 FSA image and construction and inversion. ...... 98 5.10 Algorithm to solve for variables. ...... 99 5.11 InputhandlingcodeinMantis. 102 5.12 InputhandlingcodeinMambo. 103 vii viii List of Tables 2.1 Subject programs used in our empirical evaluation. .......... 27 2.2 Precision and timing results for SqlCheck................... 29 3.1 Resourceusagefromevaluation.. ..... 52 3.2 Evaluationresults. .............................. 53 4.1 Statisticsonsubjects’files. ..... 71 4.2 File and memory results for test subjects. ....... 72 4.3 Timingresultsfortestsubjects.. ...... 72 4.4 Bugreports..................................... 74 4.5 Analysis results for input validation functions. ............ 75 4.6 Explanation of vulnerabilities. ....... 77 5.1 Tracelogfiledata. ................................ 104 5.2 Iterations to find an injection vulnerability. .......... 105 Abstract With the rise of the Internet, web applications, such as online banking and web-based email, have become integral to many people’s daily lives. Web applications have brought with them new classes of computer security vulnerabilities, such as SQL injection and cross-site scripting (XSS), that in recent years have exceeded previously prominent vulner- ability classes, such as buffer overflows, in both reports of new vulnerabilities and reports of exploits. SQL injection and XSS
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages141 Page
-
File Size-