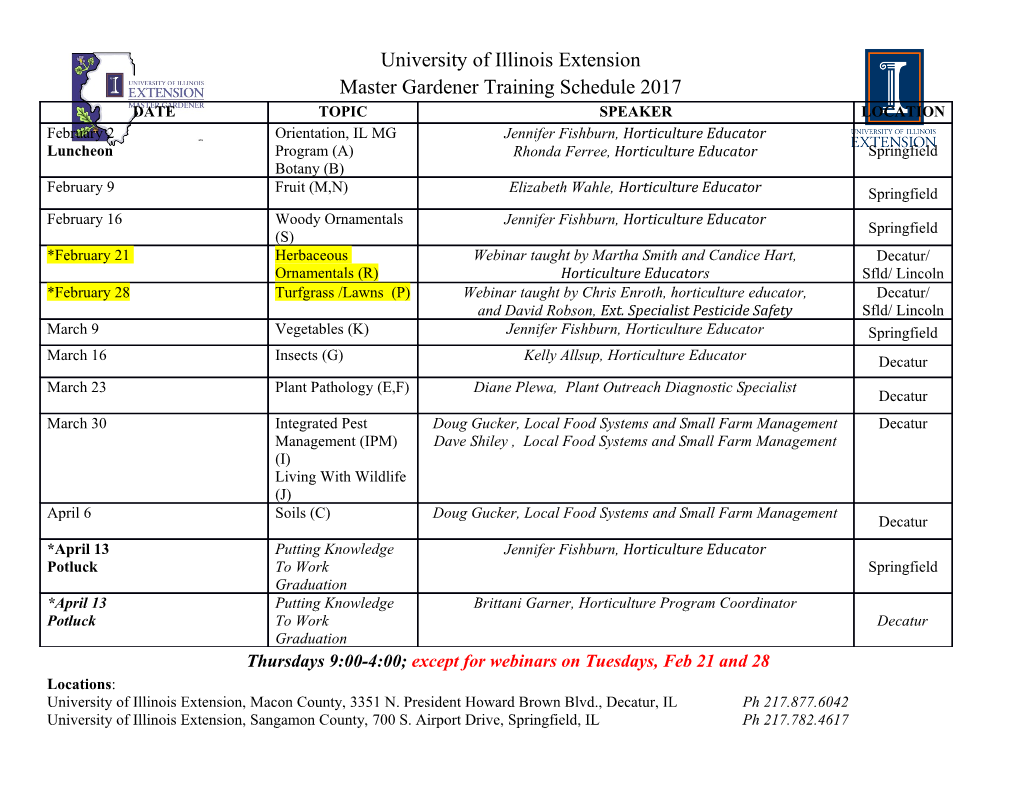
Hands On: C Programming and Unix Application Design: UNIX System Calls and Subroutines using C c A. D. Marshall 1998-2004 ii Contents 1 C/C++ Program Compilation 1 1.1 Creating, Compiling and Running Your Program . 1 1.1.1 Creating the program . 1 1.1.2 Compilation . 2 1.1.3 Running the program . 3 1.2 The C Compilation Model . 3 1.2.1 The Preprocessor . 3 1.2.2 C Compiler . 5 1.2.3 Assembler . 5 1.2.4 Link Editor . 5 1.2.5 Some Useful Compiler Options . 5 1.2.6 Using Libraries . 6 1.2.7 UNIX Library Functions . 7 1.2.8 Finding Information about Library Functions . 7 1.3 Lint | A C program verifier . 8 1.4 Exercises . 9 2 C Basics 11 2.1 History of C . 11 2.2 Characteristics of C . 12 2.3 C Program Structure . 14 2.4 Variables . 16 2.4.1 Defining Global Variables . 17 2.4.2 Printing Out and Inputting Variables . 19 2.5 Constants . 19 2.6 Arithmetic Operations . 20 2.7 Comparison Operators . 21 2.8 Logical Operators . 22 iii iv CONTENTS 2.9 Order of Precedence . 22 2.10 Exercises . 24 3 Conditionals 27 3.1 The if statement . 27 3.2 The ? operator . 28 3.3 The switch statement . 29 3.4 Exercises . 31 4 Looping and Iteration 33 4.1 The for statement . 33 4.2 The while statement . 34 4.3 The do-while statement . 36 4.4 break and continue ....................... 37 4.5 Exercises . 37 5 Arrays and Strings 43 5.1 Single and Multi-dimensional Arrays . 43 5.2 Strings . 44 5.3 Exercises . 45 6 Functions 47 6.1 void functions . 48 6.2 Functions and Arrays . 48 6.3 Function Prototyping . 49 6.4 Exercises . 51 7 Further Data Types 57 7.1 Structures . 57 7.1.1 Defining New Data Types . 58 7.2 Unions . 59 7.3 Coercion or Type-Casting . 61 7.4 Enumerated Types . 62 7.5 Static Variables . 63 7.6 Exercises . 64 8 Pointers 65 8.1 What is a Pointer? . 65 8.2 Pointer and Functions . 69 CONTENTS v 8.3 Pointers and Arrays . 71 8.4 Arrays of Pointers . 73 8.5 Multidimensional arrays and pointers . 74 8.6 Static Initialisation of Pointer Arrays . 77 8.7 Pointers and Structures . 77 8.8 Common Pointer Pitfalls . 78 8.8.1 Not assigning a pointer to memory address before using it 78 8.8.2 Illegal indirection . 79 8.9 Exercise . 80 9 Dynamic Memory Allocation and Dynamic Structures 81 9.1 Malloc, Sizeof, and Free . 81 9.2 Calloc and Realloc . 83 9.3 Linked Lists . 84 9.4 Full Program: queue.c ...................... 84 9.5 Exercises . 88 10 Advanced Pointer Topics 91 10.1 Pointers to Pointers . 91 10.2 Command line input . 93 10.3 Pointers to a Function . 94 10.4 Exercises . 96 11 Low Level Operators and Bit Fields 99 11.1 Bitwise Operators . 99 11.2 Bit Fields . 101 11.2.1 Bit Fields: Practical Example . 102 11.2.2 A note of caution: Portability . 104 11.3 Exercises . 104 12 The C Preprocessor 107 12.1 #define . 108 12.2 #undef . 109 12.3 #include . 109 12.4 #if | Conditional inclusion . 109 12.5 Preprocessor Compiler Control . 110 12.6 Other Preprocessor Commands . 111 12.7 Exercises . 112 vi CONTENTS 13 C, UNIX and Standard Libraries 113 13.1 Advantages of using UNIX with C . 113 13.2 Using UNIX System Calls and Library Functions . 114 14 Integer Functions, Random Number, String Conversion, Search- ing and Sorting: <stdlib.h> 117 14.1 Arithmetic Functions . 117 14.2 Random Numbers . 119 14.3 String Conversion . 121 14.4 Searching and Sorting . 122 14.5 Exercises . 123 15 Mathematics: <math.h> 125 15.1 Math Functions . 125 15.2 Math Constants . 126 16 Input and Output (I/O):stdio.h 129 16.1 Reporting Errors . 129 16.1.1 perror() .........................129 16.1.2 errno . 130 16.1.3 exit() . 130 16.2 Streams . 130 16.2.1 Predefined Streams . 131 16.3 Basic I/O . 132 16.4 Formatted I/O . 133 16.4.1 Printf . 133 16.5 scanf . 135 16.6 Files . 135 16.6.1 Reading and writing files . 136 16.7 sprintf and sscanf . 137 16.7.1 Stream Status Enquiries . 138 16.8 Low Level I/O . 138 16.9 Exercises . 140 17 String Handling: <string.h> 143 17.1 Basic String Handling Functions . 143 17.1.1 String Searching . 145 17.2 Character conversions and testing: ctype.h . 147 CONTENTS vii 17.3 Memory Operations: <memory.h> . 148 17.4 Exercises . 148 18 File Access and Directory System Calls 151 18.1 Directory handling functions: <unistd.h> . 151 18.1.1 Scanning and Sorting Directories:<sys/types.h>,<sys/dir.h>152 18.2 File Manipulation Routines: unistd.h, sys/types.h, sys/stat.h . 155 18.2.1 File Access . 155 18.2.2 File Status . 156 18.2.3 File Manipulation:stdio.h, unistd.h . 157 18.2.4 Creating Temporary FIles:<stdio.h> . 158 18.3 Exercises . 158 19 Time Functions 161 19.1 Basic time functions . 161 19.2 Example time applications . 162 19.2.1 Example 1: Time (in seconds) to perform some com- putation . 163 19.2.2 Example 2: Set a random number seed . 163 19.3 Exercises . 164 20 Process Control: <stdlib.h>,<unistd.h> 165 20.1 Running UNIX Commands from C . 165 20.2 execl() . 166 20.3 fork() . 167 20.4 wait() . 168 20.5 exit() . ..
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages613 Page
-
File Size-