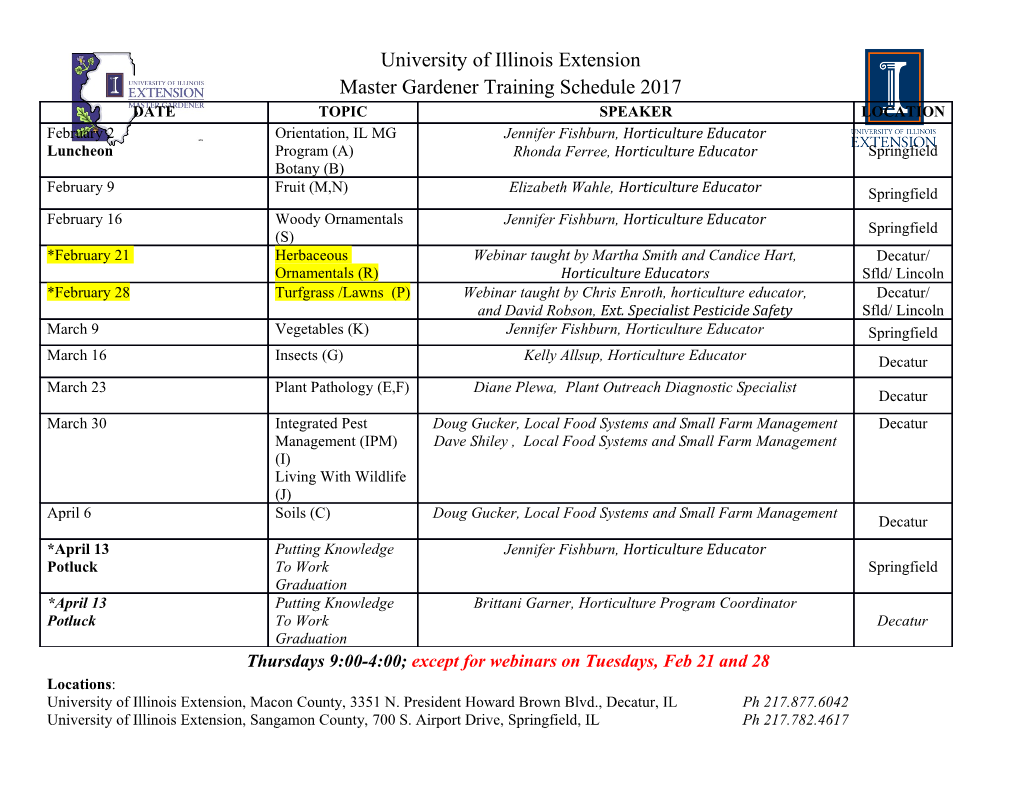
Chapter 1 Basic Signals and Systems January 17, 2007 Overview Matlab is an ideal software tool for studying Digital Signal Processing. Its language has many functions that are commonly needed to create and process signals. The plotting capa- bility of Matlab makes it possible to view the results of processing and gain understanding into complicated operations. In this first chapter, we present some of the basics of DSP in the context of Matlab. At this point, some of the exercises are extremely simple so that familiarity with the Matlab environment can be acquired. Generating and plotting signals is treated first, followed by the operation of difference equations as the basic linear time-invariant system. An important part of this chapter is understanding the role of the numerical computation of the Fourier transform (DTFT). Since Matlab is a numerical environment we must manipulate samples of the Fourier transform, rather than formulas. We also examine the signal property called group delay. Finally, the sampling process is studied to show the effects of aliasing, and different reconstruction schemes. There many excellent textbooks that provide background reading for the projects in this chapter. We mention as examples the books by Jackson (1986), McGillem and Cooper (1974), Oppenheim and Schafer (1989), Oppenheim and Willsky (1983), and Proakis and Manolakis (1988). In each of these sections we have indicated specific background reading from Oppenheim and Schafer (1989) but similar background reading can be found in most other texts on Digital Signal Processing. 1 Computer-Based Exercises for Signal Processing Signals This material was prepared by and is the property of CASPER, an informal working group of faculty from a number of universities. The working group consists of Professors C. Sidney Burrus, James H. McClellan, Alan V. Oppenheim, Thomas W. Parks, Ronald W. Schafer, and Hans W. Sch¨ußler. This material should not be reproduced or distributed without written permission. Signals continued 3 Signals Overview The basic signals used often in digital signal processing are the unit impulse signal δ[n], ex- ponentials of the form anu[n], sine waves, and their generalization to complex exponentials. The following projects are directed at the generation and representation of these signals in Matlab. Since the only data type in Matlab is the M N matrix, signals must be × represented as vectors: either M 1 matrices if column vectors, or 1 N matrices if row vec- × × tors. In Matlab all signals must be finite in length. This contrasts sharply with analytical problem solving where a mathematical formula can be used to represent an infinite-length signal, e.g., a decaying exponential, anu[n]. A second issue is the indexing domain associated with a signal vector. Matlab assumes by default that a vector is indexed from 1 to N, the vector length. In contrast, a signal vector is often the result of sampling a signal over some domain where the indexing runs from 0 to N 1; or, perhaps, the sampling starts at some arbitrary index that is negative, − say from N. The information about the sampling domain cannot be attached to the − signal vector containing the signal values. Instead the user is forced to keep track of this information separately. Usually this is no big problem until it comes time to plot the signal, in which case the horizontal axis must be labelled properly. A final point is the useage of Matlab’s vector notation to generate signals. A signficant power of the Matlab environment is its high-level notation for vector manipulation; for loops are almost always unnecessary. When creating signals such as a sine wave, it is best to apply the sin function to a vector argument, consisting of all the time samples. In the following projects, we will treat the common signals encountered in digital signal processing: impulses, impulse trains, exponentials, and sinusoids. Background Reading Oppenheim and Schafer (1989), Chapter 2, sections 2.0 and 2.1. Project 1: Basic Signals Project Description This project concentrates on the issues involved with generation of some basic discrete-time signals in Matlab. Much of the work centers on using internal Matlab vector routines for signal generation. In addition, a sample Matlab function will be implemented. Hints Plotting discrete-time signals is done with the comb function in Matlab. The following Matlab code will create 31 points of a discrete-time sinusoid. nn = 0:30; %-- vector of time indices sinus = sin(nn/2+1); Notice that the n = 0 index must be referred to as nn(1), due to Matlab’s indexing scheme; likewise, sinus(1) is the first value in the sinusoid. When plotting the sine wave we would use the comb function which produces the discrete-time signal plot commonly seen 4 Signals continued SINE WAVE 1 o o o o o o o 0.8 o o o o 0.6 o 0.4 o o 0.2 o o o 0 o o -0.2 o o o -0.4 o o -0.6 o o -0.8 o o o -1 o o 0 5 10 15 20 25 30 TIME INDEX (n) Figure 1: Example of Plotting a discrete-time signal with comb. in DSP books, see Fig. 1: comb( nn, sinus ); The first vector argument must be given in order to get the correct n-axis. For comparison, try comb(sinus) to see the default labelling. Exercise 1.1: Basic Signals—Impulses The simplest signal is the (shifted) unit impulse signal: 1 n = n δ[n n ]= 0 (1.1) − 0 0 n = n ( 6 0 In order to create an impulse in Matlab, we must decide how much of the signal is of interest. If the impulse δ[n] is going to be used to drive a causal LTI system, we might want to see the L points from n = 0 to n = L 1. If we choose L = 31, the following Matlab − code will create an “impulse” L = 31; nn = 0:(L-1); imp = zeros(L,1); imp(1) = 1; Notice that the n = 0 index must be referred to as imp(1), due to Matlab’s indexing scheme. Signals continued 5 (a) Generate and plot the following sequences. In each case, the horizontal (n) axis should extend only over the range indicated and should be labelled accordingly. Each sequence should be displayed as a discrete-time signal using comb. x [n]= .9δ[n 5] 1 n 20 1 − ≤ ≤ x [n]= .8δ[n] 15 n 15 2 − ≤ ≤ x [n] = 1.5δ[n 333] 300 n 350 3 − ≤ ≤ x [n] = 4.5δ[n + 7] 10 n 0 4 − ≤ ≤ (b) The shifted impulses, δ[n n ], can be used to build a weighted impulse train, with − 0 period P and total length MP : M 1 − s[n]= A δ[n ℓP ] (1.2) ℓ − Xℓ=0 The weights are Aℓ; if they are all the same, the impulse train is periodic with period P . Generate and plot a periodic impulse train whose period is P = 5, and whose length is 50. Start the signal at n = 0. Try to use one or two vector operations rather than a for loop to set the impulse locations. How many impulses are contained within the finite-length signal? (c) The following Matlab code will produce a repetitive signal in the vector x: x = [0;1;1;0;0;0] * ones(1,7); x = x(:); size(x) %<--- return the signal length Plot x in order to visualize its form; then give a mathematical formula similar to (1.2) to describe this signal. Exercise 1.2: Basic Signals—Sinusoids Another very basic signal is the cosine wave. In general, it takes three parameters to completely describe a real sinusoidal signal: amplitude (A), frequency (ω0), and phase (φ). x[n]= A cos(ω0n + φ) (1.3) (a) Generate and plot each of the following sequences. Use Matlab’s vector capability to do this with one function call by taking the cosine (or sine) of a vector argument. In each case, the horizontal (n) axis should extend only over the range indicated and should be labelled accordingly. Each sequence should be displayed as a sequence using comb. x [n] = sin π n 0 n 25 1 17 ≤ ≤ x [n] = sin π n 15 n 25 2 17 − ≤ ≤ x [n] = sin(3πn + π ) 10 n 10 3 2 − ≤ ≤ x [n] = cos π n 0 n 50 4 √23 ≤ ≤ Give a simpler formula for x3[n] that does not use trignometric functions. Explain why x4[n] is not a periodic sequence. 6 Signals continued (b) Write a Matlab function that will generate a finite-length sinusoid. The function will need a total of 5 input arguments; 3 for the parameters, and 2 more to specify the first and last n index of the finite-length signal. The function should return a column vector that contains the values of the sinusoid. Test this function by plotting the results for various choices of the input parameters. In particular, show how to generate the signal 2 sin(πn/11) for 20 n 20. − ≤ ≤ (c) Modification: Write the function to return two arguments: a vector of indices over the range of n, as well as the values of the signal. Exercise 1.3: Sampled Sinusoids Often a discrete-time signal is produced by sampling a continuous-time signal such as a constant frequency sine wave. The interrelationship between the continuous-time frequency and the sampling frequency is the main point of the Nyquist-Shannon Sampling Theorem, which requires that the sampling frequency be at least twice the highest frequency in the signal for perfect reconstruction. In general, a continuous-time sinusoid is given by the following mathematical formula: s(t)= A cos(2πf0t + φ) (1.4) where A is the amplitude, f0 is the frequency in Hertz, and φ is the initial phase.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages404 Page
-
File Size-