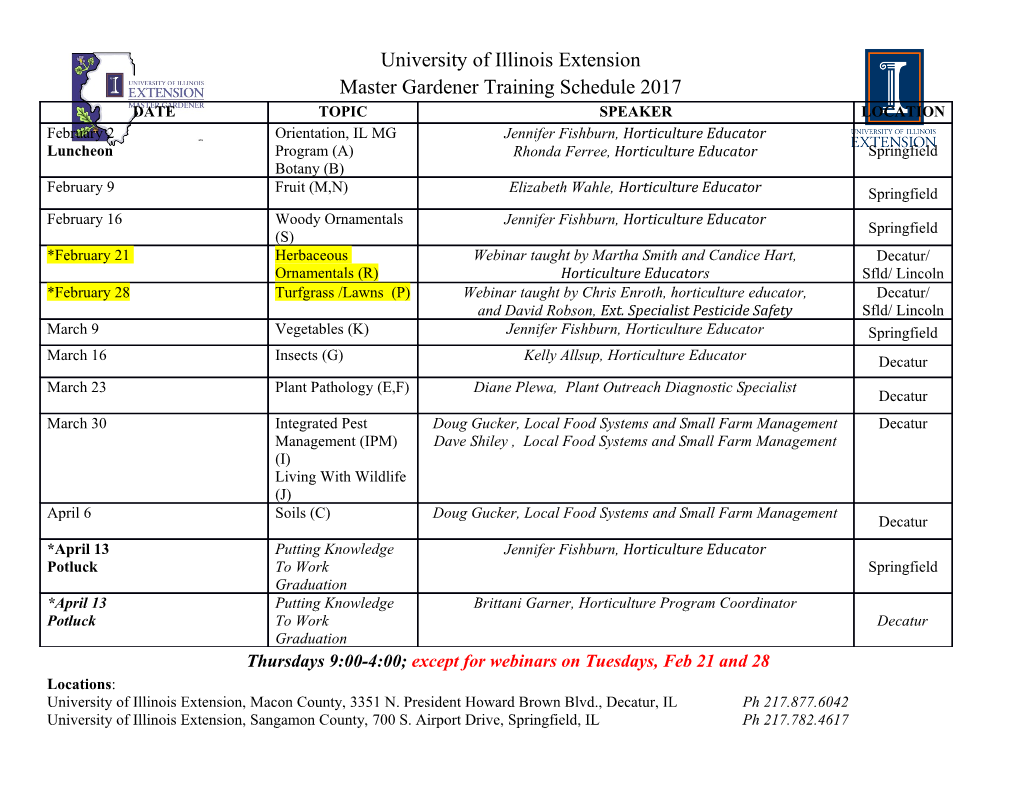
MATH 235.9 WRITTEN HW 4: APPLICATIONS ANDREW J. HAVENS This is a collection of short projects rather than a regular problem set. It will be due on the last day of class. Please choose at least three to work on. It is recommended you discuss these with your classmates, or work in groups as needed; however each person still must submit their individual solutions, written up in an organized way (keep problems from a given topic together!) Any additional work may be counted as bonus credit towards your written assignment average. The topics are: linear ciphers, matrices and groups, graph theory, finite vector spaces, linear recurrence relations, and quaternions. Linear Ciphers. These problems illustrate and give practice with some constructions of linear ciphers, which use linear algebra to obscure plaintext. We will first consider monoalphabetic substitution ciphers, where each letter of plaintext is mapped to a single letter of ciphertext. Affine maps in one dimension can accomplish this, and furnish simple but easy to break examples of classic ciphers. It is advised, should you choose to complete this project, that you write programs to perform the computations in this problem, as they are quite repetitive and easily amenable to coding. If you do work on this, you are welcome to come discuss your code with me. There are 26 basic letters in the english alphabet, however, since 26 is not prime, not every element of integers mod 26 can be inverted multiplicatively (consider: 2(13) ≡ 0 mod 26. Thus, we will work with the field F29 of integers mod 29. We can assign to each letter an integer, and use the remaining elements of F29 for punctuation and the space character. For example, we could use the following scheme: A B C D E F G H I J K L M N O 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 P Q R S T U V W X Y Z . ? x y 16 17 18 19 20 21 22 23 24 25 26 27 28 0 Figure 1. Our alphanumeric correspondence. Recall that the arithmetic in F29 works as follows: to take the sum of two elements, simply add the integers, then find the remainder modulo 29, e.g. 14 + 18 = 32 in Z, and 32 ≡ 3 mod 29, so in F29, 14 + 18\="3 (I will simply write ≡ for \=" henceforth in these problems, where it is clear and implicit that we are working over F29.) Similarly, for multiplication, one takes the product as usual in Z and then reduce modulo 29, e.g. 2 × 16 = 32 ≡ 3. Division ∗ by a 2 F29 − f0g =: F29 corresponds to solving the congruence ax ≡ 1 mod 29 for x 2 F29. It may be helpful to create a table of multiplicative inverses (you may look one up, or try to write a program to produce one for general Fp; the extended Euclidean algorithm is useful for this). 1 2 ANDREW J. HAVENS (1) An early cipher is known as the Caesar cipher or shift cipher, which simply shifts letters by a fixed value (this works even mod 26). For example, shifting every letter by 3 in the above scheme turns the plaintext message \is the kernel nontrivial?" to \lvcwkhcnhuqhocqrqwulyldob". Note that if we suspected it was a shift cipher, it would be easy to decode if we knew about the above alphanumeric assignment scheme: at worst we check 28 possible shifts to see if anything produced is not babble. If we are more clever, we use that \e" is the most common letter in written english, and that spaces are also common. Letters \c", \h", \l" and \q" all occur with the same high frequency (12%) in the ciphertext, so we might might guess that one of these letters is the encipherment of \e" and another of the space character. Observe that \c"∼ 3 and \h"∼ 8 differ by 5, as do \x y"∼ 0 and \e"∼ 5, so we might assume that a shift of 3 is most probable, and try to decrypt using a shift of −3 ≡ 26 mod 29. ∗ Another variation is a linear cipher, where we fix a 2 F29 and map the numeric code x of each letter of plaintext to ax. For example, using a = 13, one has the ciphertext \ao ?qg .gbhgk huh?bayamkp". This is also susceptible to letter frequency analysis. An affine monoalphabetic cipher combines these two into one: the key is a pair ∗ (a; b) 2 F29 × F29, and the ciphertext is produced by the rule c(x) ≡ ax + b mod 29. Assume that we fix the scheme of alphanumeric assignment above. (a) Use affine encryption with the key (8; 14) to encipher the following plaintext: \What is the fundamental theorem of linear algebra if not the rank nullity theo- rem?" (b) Assume you are given some cipher text, and suppose you know that \e" enciphers to \o". Knowing that an affine cipher was used, can you recover the plaintext from the given ciphertext with this information alone? Justify. (c) Suppose that you know that not only does \e" enciphers to \o" but also \t" enciphers to \q". Determine the key values a and b, and decipher the following ciphertext: \q.oxy?nuoxzsxcxbmxq ovqhxrzioxq.?vxq.oxy?nuoxzsx?x .bg.xbmxszuip". (d) Use frequency analysis or other logical assumptions about the usual form of english text to decipher the following affine ciphertext, and also provide the key pair: \voxosoy kqeqlsqi?.k?qukqeq?pils?qkd.avcen.?vbaksok tdbk e.ikimdscsqnfk?l?ok{ {eqikobetlukzs.ock ?oci.klsqi?.kdsvbi.ok?qukp?osdkd.avc?q?laosofk.lxosf?". Note that this text is padded with a few random characters at the beginning and end. The dashes before and after the line break indicate that there is no space in the original ciphertext, and are inserted to ensure that the text fits on the page. It should be clear from the above that a message is not very secure when enciphered by these methods. Among the obvious flaws: choosing to include spaces and punc- tuation makes frequency analysis much easier. Generally, any monoalphabetic substi- tution cipher, even if it does not encrypt spaces or punctuation, is highly susceptible to frequency analysis provided a sufficient amount of plaintext is available. We thus will consider using linear algebra to encrypt things polyalphabetically, where a given plaintext character may be enciphered to different ciphertext values across the same message. MATH 235.9 WRITTEN HW 4: APPLICATIONS 3 (2) One of the most famous and basic ways to encrypt plaintext polyalphabetically is the family of ciphers known as Hill ciphers. (INSERT HISTORY) These are linear ciphers in more than one dimension. For the Hill cipher of size n over F29, one chooses an invertible square matrix A 2 Matn×n(F29), and wraps the numerically encoded plain text into an n × m matrix P, where m depends on the length of the message and any padding used, and one computes the cipher matrix C = AP. As a simple example, we could take our key to be the matrix A 2 Mat4×4(F29) given by 2 4 17 5 1 3 6 15 24 12 11 7 A = 6 7 : 4 28 2 7 13 5 3 6 21 0 Then if the plaintext message we wished to encode was \Wizards pluck joy from the quivering box"1, then we'd have plaintext matrix 2 23 18 16 11 25 15 8 21 18 0 3 6 9 4 12 0 0 13 5 9 9 2 7 P = 6 7 : 4 26 19 21 10 6 0 0 22 14 15 5 1 0 3 15 18 20 17 5 7 24 Note that the text is wrapped here column to column from left to right and top to bottom. Other schemes could be used to add a layer of difficulty in recovering the text (such techniques amount to composing the Hall cipher method with a transposition cipher, which permutes the arrangement of plaintext or ciphertext). The ciphertext would be produced by computing C = AP mod 29 and reading off the ten columns of C, converting numbers using the table in figure 1. (a) Use the above encryption matrix to encrypt the given plaintext above, and give the output as text using the table in figure 1. (b) Find the inverse of the above encryption matrix (you can use Gauss-Jordan, mod 29). This is the decryption key. (c) Using the above key, decipher the following text: \kxaglfqtbwviaqxmho.o v?jyfoamyhuwcvfkhus" Bonus : Hill ciphers are still susceptible to various forms of analysis, and are computationally expensive enough that they did not catch on during the era when they were less vulnera- ble to machine-assisted analysis. With modern computers, Hill's cipher, and variations thereof can be rapidly implemented, but also more readily analyzed and deciphered. Try to decrypt the following text, which was encrypted using a dynamic variation of a Hill cipher of size 2: \o?bawcxikerlmopk?srgakhiebtojydvvhcxzb.pyyvjcs tos gveunffktbwolyracgfslsjjahrob" 1This sentence is constructed so as to have letter frequencies which do not match the average; every consonant appears exactly once, and note also that there are only two \e"s, just as many \o"s and \u"s and even more \i"s. 4 ANDREW J. HAVENS Groups of Matrices. These problems explore the definitions of some groups of matrices. Groups are the natural mathematical objects to consider when studying symmetries. A group is a mathematical set G, together with a binary product, µ: G × G ! G called multiplication such that (i:) The multiplication is associative: µ(g; µ(h; k)) = µ(µ(g; h); k) for any g; h; k 2 G.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages15 Page
-
File Size-