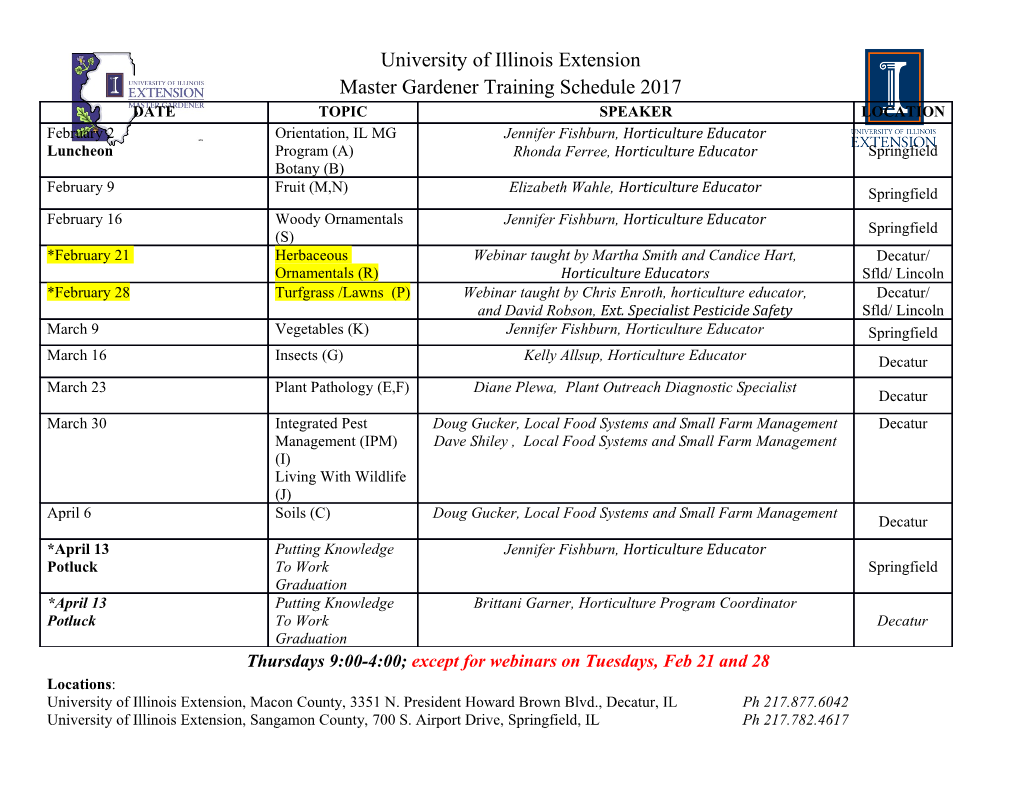
Readings • Chapter 6 Priority Queues & Binary Heaps › Section 6.1-6.4 CSE 373 Data Structures Winter 2007 Binary Heaps 2 FindMin Problem Priority Queue ADT • Quickly find the smallest (or highest priority) • Priority Queue can efficiently do: item in a set ›FindMin() • Applications: • Returns minimum value but does not delete it › Operating system needs to schedule jobs according to priority instead of FIFO › DeleteMin( ) › Event simulation (bank customers arriving and • Returns minimum value and deletes it departing, ordered according to when the event › Insert (k) happened) • In GT Insert (k,x) where k is the key and x the value. In › Find student with highest grade, employee with all algorithms the important part is the key, a highest salary etc. “comparable” item. We’ll skip the value. › Find “most important” customer waiting in line › size() and isEmpty() Binary Heaps 3 Binary Heaps 4 List implementation of a Priority BST implementation of a Priority Queue Queue • What if we use unsorted lists: • Worst case (degenerate tree) › FindMin and DeleteMin are O(n) › FindMin, DeleteMin and Insert (k) are all O(n) • In fact you have to go through the whole list • Best case (completely balanced BST) › Insert(k) is O(1) › FindMin, DeleteMin and Insert (k) are all O(logn) • What if we used sorted lists • Balanced BSTs › FindMin and DeleteMin are O(1) › FindMin, DeleteMin and Insert (k) are all O(logn) • Be careful if we want both Min and Max (circular array or doubly linked list) › Insert(k) is O(n) Binary Heaps 5 Binary Heaps 6 1 Better than a speeding BST Binary Heaps • A binary heap is a binary tree (NOT a BST) that • Can we do better than Balanced Binary is: Search Trees? › Complete: the tree is completely filled except • Very limited requirements: Insert, possibly the bottom level, which is filled from left to FindMin, DeleteMin. The goals are: right › FindMin is O(1) › Satisfies the heap order property › Insert is O(log N) • every node is less than or equal to its children • or every node is greater than or equal to its children › DeleteMin is O(log N) • The root node is always the smallest node › or the largest, depending on the heap order Binary Heaps 7 Binary Heaps 8 Binary Heap vs Binary Search Heap order property Tree • A heap provides limited ordering information Binary Heap Binary Search Tree • Each path is sorted, but the subtrees are not min value sorted relative to each other 5 94 › A binary heap is NOT a binary search tree 10 94 10 97 -1 min 2 1 value 0 1 97 24 5 24 4 6 2 6 0 Parent is less than both Parent is greater than left 7 5 8 4 7 These are all valid binary heaps (minimum) left and right children child, less than right child Binary Heaps 9 Binary Heaps 10 Structure property Examples 6 • A binary heap is a complete tree 2 4 2 › All nodes are in use except for possibly the 4 6 complete tree, 5 right end of the bottom row heap order is "max" 2 2 not complete 4 6 5 6 7 5 7 4 complete tree, complete tree, but min heap order is "min" heap order is broken Binary Heaps 11 Binary Heaps 12 2 Array Implementation of FindMin and DeleteMin Heaps • Root node = A[1] • FindMin: Easy! • Children of A[i] = A[2i], A[2i + 1] › Return root value A[1] 2 • Keep track of current size N (number › Run time = ? 4 3 of nodes) 7 5 8 10 1 2 11 9 6 14 2 3 • DeleteMin: value - 2 4 6 7 5 4 6 › Delete (and return) value index 01234567 7 5 at root node 4 5 N = 5 Binary Heaps 13 Binary Heaps 14 Maintain the Structure DeleteMin Property • We now have a “Hole” at the root 4 3 7 5 8 10 • Delete (and return) 4 3 › Need to fill the hole with another value 11 9 6 14 value at root node 7 5 8 10 • When we get done, the 11 9 6 14 tree will have one less 4 3 node and must still be 7 5 8 10 complete 11 9 6 14 Binary Heaps 15 Binary Heaps 16 Maintain the Heap Property DeleteMin: Percolate Down 14 • The last value has lost its ? 14 3 ? 3 node 14 › we need to find a new 4 3 4 4 8 place for it 7 5 8 10 7 5 8 10 7 5 14 10 • We can do a simple 4 3 11 9 6 11 9 6 11 9 6 insertion sort - like operation to find the 7 5 8 10 • Keep comparing with children A[2i] and A[2i + 1] • Copy smaller child up and go down one level correct place for it in the 11 9 6 • Done if both children are ≥ item or reached a leaf node tree • What is the run time? Binary Heaps 17 Binary Heaps 18 3 Percolate Down DeleteMin: Run Time Analysis PercDown(i:integer, x :integer): { // N is the number of entries in heap// • Run time is O(depth of heap) j : integer; • A heap is a complete binary tree Case{ 2i > N : A[i] := x; //at bottom// • Depth of a complete binary tree of N 2i = N : if A[2i] < x then A[i] := A[2i]; A[2i] := x; nodes? else A[i] := x; 2i < N : if A[2i] < A[2i+1] then j := 2i; › depth = ⎣log2(N)⎦ else j := 2i+1; if A[j] < x then • Run time of DeleteMin is O(log N) A[i] := A[j]; PercDown(j,x); else A[i] := x; }} Binary Heaps 19 Binary Heaps 20 Maintain the Structure Insert Property • Add a value to the tree 2 • The only valid place for a new node in a • Structure and heap 3 2 order properties must complete tree is at the 3 still be correct when we 4 8 end of the array are done 7 5 14 10 • We need to decide on 4 8 11 9 6 the correct value for the 7 5 14 10 new node, and adjust 11 9 6 the heap accordingly Binary Heaps 21 Binary Heaps 22 Maintain the Heap Property Insert: Percolate Up 2 3 3 ? 3 • The new value goes where? 4 8 4 8 8 • We can do a simple insertion 7 5 14 10 7 14 10 7 4 14 10 3 sort operation on the path from ? ? 11 9 6 11 9 6 5 11 9 6 5 the new place to the root to find 4 8 2 2 the correct place for it in the tree 7 5 14 10 • Start at last node and keep comparing with parent A[i/2] 11 9 6 • If parent larger, copy parent down and go up one level • Done if parent ≤ item or reached top node A[1] 2 •Run time? Binary Heaps 23 Binary Heaps 24 4 Insert: Done PercUp 2 PercUp(i : integer, x : integer): { if i = 1 then A[1] := x 3 8 else if A[i/2] < x then 7 4 14 10 A[i] := x; else 11 9 6 5 A[i] := A[i/2]; Percup(i/2,x); } •Run time? Binary Heaps 25 Binary Heaps 26 Sentinel Values Binary Heap Analysis • Every iteration of Insert needs to test: -∞ • Space needed for heap of N nodes: O(MaxN) › if it has reached the top node A[1] 2 › An array of size MaxN, plus a variable to store the › if parent ≤ item size N, plus an array slot to hold the sentinel • Can avoid first test if A[0] contains a very 3 8 large negative value •Time › sentinel -∞ < item, for all items 7 4 10 9 ›FindMin: O(1) • Second test alone always stops at top 11 9 6 5 › DeleteMin and Insert: O(log N) › BuildHeap from N inputs : O(N) (forthcoming) value -∞ 2 3 8 7 4 10 9 11 9 6 5 index 012345678 9 10 11 12 13 Binary Heaps 27 Binary Heaps 28 5.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages5 Page
-
File Size-