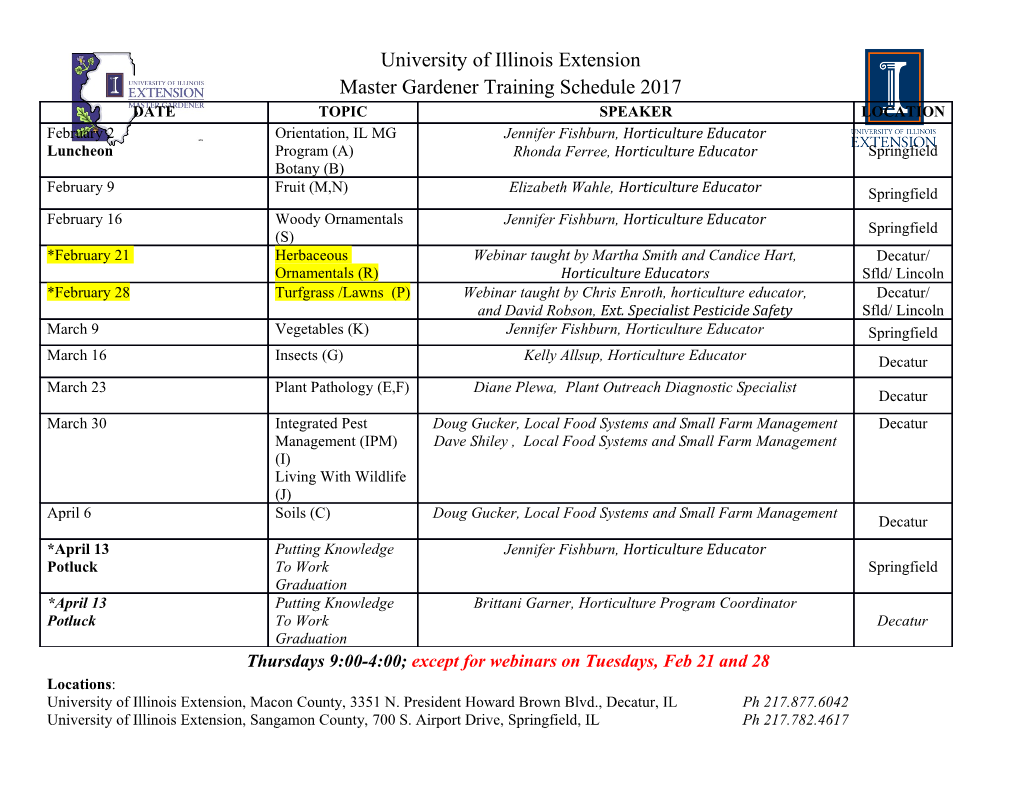
7/17/2018 C/C++ Programming for Engineers: Loops John T. Bell Department of Computer Science University of Illinois, Chicago Review What would be the best data type to use to record the concentration of a solution in a chemistry laboratory experiment? A. bool B. char C. double D. int E. long int 2 1 7/17/2018 The Power of Loops • Computers are dumb machines, that only do what they are told. • Their power lies in that they follow instructions very quickly, and don’t mind repeating the same instruction millions of times per second. Hence the power of the loop. • The programmers job is then to tell the computer how to loop, when to stop looping, and what to do each pass through the loop. 3 While Loops • The simplest loops to understand are while loops, which continue looping as long as some condition remains true. • The condition is evaluated BEFORE each iteration of the loop, and the loop is executed only if the condition is true. • ( If the condition is initially false, then the loop will execute zero times, i.e. not at all. ) 4 2 7/17/2018 While Loop Syntax while( condition ) { // code in body of loop condition False ? } True body • If braces are omitted, then a Following single statement comprises code the body of the loop. 5 While Example int guess = -1, answer = rand( ) % 10 + 1; Must be initialized to ensure loop entry while( guess != answer ) { cout << “Enter a guess from 1 to 10: “; cin >> guess; } cout << “That’s right! Congratulations!\n”; 6 3 7/17/2018 Looping With a Counter • Although a while loop could be used with a counter to loop a specified number of times, that is not good practice. • The best approach when a loop must execute a specified number of times is with a for loop, which initializes the counter, tests it on each iteration, and ( typically ) increments it on each iteration. 7 For Loop Syntax for( init; condition; incr ) { // code in body of loop init } condition False ? • If braces are omitted, then a True incr body single statement comprises the body of the loop. Following • Note “incr” always happens code after executing the body. 8 4 7/17/2018 The for incrementation component most commonly uses auto-increment: • “i++;”, as a stand-alone statement, is equivalent to “i += 1;” or “i = i + 1;” • “i--;”, as a stand-alone statement, is equivalent to “i -= 1;” or “i = i - 1;” • There is more to auto-increment ( and auto- decrement ), which we cover elsewhere. 9 For Loop Example cout << “ i i^2 i^3” << endl; int i; for( i = 1; i <= 10; i++ ) { cout << i << “ “ << i * i << “ “ << i * i * i; cout << endl; } cout << “After the loop, i = “ << i << endl; 10 5 7/17/2018 What will be printed after the loop ends? for( i = 1; i <= 10; i++ ) { // Assume loop body does not change i } cout << “After the loop, i = “ << i << endl; A. 0 B. 1 C. 10 D. 11 E. Undefined. It may depend on the compiler. 11 Do-While Loops • Do-while loops are nearly identical to whiles. • The condition is evaluated AFTER each iteration of the loop, and the loop is repeated only if the condition is true. • A do-while loop will always execute at least once. After that it functions identically to a while loop. 12 6 7/17/2018 Do-While Loop Syntax do { // code in body of loop body } while( condition ); condition False ? • Note required semi-colon True • If braces are omitted, then a Following single statement comprises code the body of the loop. ( Very rarely omitted. ) 13 Do-While Example int guess, answer = rand( ) % 10 + 1; No initialization needed do { cout << “Enter a guess from 1 to 10: “; cin >> guess; } while( guess != answer ) ; cout << “That’s right! Congratulations!\n”; 14 7 7/17/2018 Break and Continue • “break” causes a loop to finish immediately, continuing execution with the code following the loop body. ( Also used in switches. ) • “continue” causes the current iteration of a loop to finish, starting the next iteration. – While or do-while loops will jump to the evaluation of the loop condition. – For loops will execute the “incrementation”, and then jump to the evaluation of the loop condition. 15 For Loop Flowchart Illustrating break and continue for( init; condition; incr ) { init False // code 1 condition? if( test_C ) code 1 continue; True incr test_C // code 2 if( test_B ) code 2 True break; test_B // code 3 code 3 } Following code 16 8 7/17/2018 Input Checking With a While Loop int age = -1; // Initialized to guarantee loop entry while( age < 0 || age > 120 ) { cout << “Please enter your age: “; cin >> age; if( age < 0 || age > 120 ) cout << age << “ is invalid. Try again.\n”; } // Continue while input is bad 17 Input Checking With a Do-While Loop int age; // No initialization needed do { cout << “Please enter your age: “; cin >> age; if( age < 0 || age > 120 ) cout << age << “ is invalid. Try again.\n”; } while( age < 0 || age > 120 ); //Continue while bad 18 9 7/17/2018 Input Checking With an Infinite Loop int age; // No initialization needed while( true ) { cout << “Please enter your age: “; cin >> age; if( age > 0 && age <= 120 ) // Exit loop if good break; cout << age << “ is invalid. Try again.\n”; } // Loops infinitely while input is bad 19 Review In the following statement, which of the following is the correct order in which the operators will be evaluated? A *= B + C / D – E++; A. *=, +, /, -, ++ B. /, +, -, *=, ++ C. ++, /, +, -, *= D. ++, +, /, -, *= E. None of the above. The correct order of operations is not listed here. 20 10 7/17/2018 When to Use What Kind of Loop • If you can count ( or calculate ) how many times the loop must execute, use a for loop, always with an INTEGER counter. • Else if you need to ensure the loop executes at least once, ( and can’t rig a while to do so ), use a do-while loop. ( A comment at the top of the loop improves readability. ) • Else use a while loop. 21 Review What type of loop is guaranteed to execute at least once, and then continue to repeat until a condition becomes false? A. for B. until C. do until D. while E. do while 22 11 7/17/2018 Nested Loops • The body of a loop can contain any valid code, including other loops, termed nested loops. – The internal loop does not have to be the same type as the enclosing outer loop. – A nested “for” loop is a common way to iterate over two variables and/or produce a table: for( i = 0; i < iMax; i++ ) { for( j = 0; j < jMax; j++ ) cout << i * j << “ “; // Prints numbers on a line cout << endl; // New line when j loop exits. } 23 Empty Loops • Loops can also be empty. – This is very rarely done intentionally. – Comments and braces are needed if this is done on purpose. – Error example: An infinite empty loop: int i = 0; Error here. The “i = i + 1;” is after while( i < 5 ) ; body of the loop the loop, not i = i + 1; is between the inside it, and will closing ) and the ; never be executed. 24 12 7/17/2018 Always Use an INTEGER Loop Counter int i, nLoops; // ( Assume all vars are given values. ) double x, xMin, xMax, delataX; // WRONG: for( x = xMin; x <= xMax; x += deltaX ) { . // RIGHT: for( i = 0; i < nLoops; i++ ) { x = xMin + i * deltaX; . 25 Review What value will be stored by the following C++ code: double answer = 3 + 5 / 2 * 4 / 5.0; A. 0.2 B. 3.2 C. 4.0 D. 4.6 E. 5.0 26 13 7/17/2018 Review Given the following code, what will be stored in k? int i = 5, j = 4, k = 3; k /= i++ % --j; A. 0 B. 1 C. 1.5 D. 3 E. infinity ( divide by zero error. ) 27 Infinite Loop Knock Knock Joke Knock knock Who’s there? Knock Knock who? 28 14.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages14 Page
-
File Size-