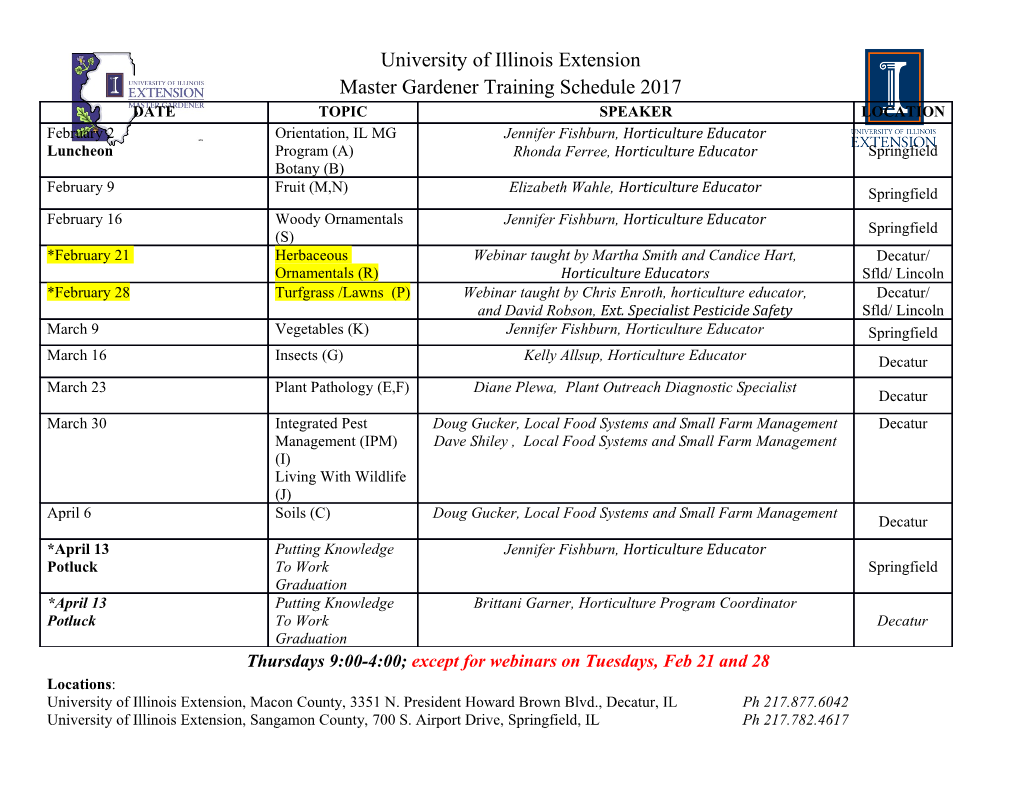
Java Primitive Numeric Types, Assignment, and Expressions: Data Types, Variables, and Declarations • A data type refers to a kind of value that a language recognizes { For example, integers, real numbers, characters, Booleans (true/false), etc. • Primitive data types represent single values and are built into a language; i.e., they are given • Java primitive numeric data types: 1. Integral types (a) byte (b) int (c) short (d) long 2. Real types (NOTE: The text calls these decimal types) (a) float (b) double • What distinguishes the various data types of a given group is the amount of storage allocated for storage { The greater the amount of storage, the greater the precision and magnitude of the value { Precision refers to the accuracy with which a value can be represented { Magnitude refers to the largest (and smallest) value that can be represented • A variable represents a storage location in memory for a value { Identifiers are used to represent variables { By convention, user-defined Java variables start with a lower case letter 1 Java Primitive Numeric Types, Assignment, and Expressions: Data Types, Variables, and Declarations (2) • Variable declarations { Java is a strongly typed language ∗ This means that a variable can only store values of a single data type { Semantics: A variable declaration tells the compiler how much storage to set aside for the variable, and how values in that memory location are to be interpreted ∗ Remember: Everything stored in memory is binary - zeroes and ones ∗ How the binary code is interpreted depends on what the system expects to find in a location { Syntax for primitive variable declaration: ∗ For example: int radius, length, width; double circleArea; { Generally, put one declaration per line 2 Java Primitive Numeric Types, Assignment, and Expressions: Integral Types • Represent whole numbers: 2, 103, -47 • Stored as binary whole numbers 0 1 2 { 21310 represents 3 × 10 + 1 × 10 + 2 × 10 = 3 × 1 + 1 × 10 + 2 × 100 = 213 { Binary only uses digits 0 and 1 0 1 2 3 4 5 6 7 { 110101012 represents 1×2 +0×2 +1×2 +0×2 +1×2 +0×2 +1×2 +1×2 = 1 × 1 + 0 × 2 + 1 × 4 + 0 × 8 + 1 × 16 + 0 × 32 + 1 × 64 + 1 × 128 = 213 { Java allocates the following storage type bytes smallest largest byte 1 -128 127 short 2 -32768 32767 int 4 -2147483648 2147483647 long 8 -9223372036854775808 9223372036854775807 3 Java Primitive Numeric Types, Assignment, and Expressions: Floating Point Types • Represent numbers with decimal points: 3.0, 66.667, -32.5 • Internally stored using scientific notation 2 { 125:3310 represented as 1:2533 × 10 = 1:2533 × 100 −3 { 0:0041710 represented as 4:17 × 10 = 4:17 × :001 { Reals are known as floating point types because 0 1 2 3 125:3310 = 125:33 × 10 = 12:533 × 10 = 1:2533 × 10 = :12533 × 10 = ::: { Java allocates the following storage type bytes smallest largest float 4 −3:40282347 × 1038 3:40282347 × 1038 double 8 −1:797693134862 × 10308 1:797693134862 × 10308 { Note that while integer values represent exact whole numbers, floating point types are often approximations to the actual value ∗ While their format allows very large numbers to be represented, it also de- creases the precision of their representation 4 Java Primitive Numeric Types, Assignment, and Expressions: Assignment and Numeric Expressions • Assignment is the most basic instruction • Syntax: • For example: x = 10; • Left side often referred to as the lvalue of the assignment Right side often referred to as the rvalue of the assignment • Semantics: The expression is evaluated and the value is stored in the variable's storage location 5 Java Primitive Numeric Types, Assignment, and Expressions: Assignment and Numeric Expressions (2) • Numeric expressions can take a number of forms: 1. Literal { Literal is an actual value { Integer syntax: ∗ Note: · If the first digit is a zero, the integer will be interpreted as an octal integer (base 8) · If the first digit is an 0x, the integer will be interpreted as a hexadecimal integer (base 16) ∗ An optional plus or minus may precede the value { Simple float syntax: { A floating point literal may also be expressed in scientific notation: { NOTE: As of Java 7, numeric literals may include underscores for readability (not indicated on the above) E.g., 32 817 423 6 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (3) { Suffix ∗ What data type does 3.41457 represent? · By default, floating point literals are interpreted as type double · By default, integral literals are interpreted as type int · To indicate a specific type, Java allows a suffix to be appended to a literal Suffix Meaning f float F d double D l long L · For example: 123L, 13.7F 2. Variable { The assignment copies the value of the variable on the right into the memory associated with the variable on the left 7 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (4) 3. Named (symbolic) constant { Value that cannot be changed during program execution { Represented by an identifier, just like a variable { MUST be initialized at declaration { Syntax: { Convention: use all caps for identifier { For example: final double COMMISSION = 0.10; 4. Value-returning method calls { A method (also called functions or subprograms in other languages) are like mini programs that perform a task ∗ For right now, we will only be concerned with value-returning methods - ones that generate a value ∗ Also, we are only concerned with methods that are supplied by Java · Later on we'll discuss how to write your own methods ∗ To use a method, you call the method ∗ Call syntax: ∗ { The value produced by such a method will 'take the place' of the call { java.lang.Math provides a number of useful class methods for performing stan- dard math functions 8 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (5) { The table below lists a number of useful Math methods grouped by function- ality; ∗ If arguments are labeled as numeric, they could be int, long, float, or double (all the same type for a given version) ∗ All calls are preceded by Math. Method** Function numeric abs(numeric x) absolute value of x numeric max(numeric x, numeric y) larger of x and y numeric min(numeric x, numeric y) smaller of x and y double ceil(double x) smallest whole number ≥ x double floor(double x) largest whole number ≤ x int round(float x) x rounded to the nearest whole number long round(double x) " double sin(double x) sine of x double cos(double x) cosine of x double tan(double x) tangent of x double toDegrees(double x) x converted to degrees double toRadians(double x) x converted to radians double pow(double x, double y) xy p double sqrt(double x) x p double cbrt(double x) 3 x double random() 0:0 ≤ value < 1:0 ** NOTE: trig functions are based on radians (And constants: ∗ Math.E ∗ Math.PI) { This package does not need to be imported to be used { For example: int x, y, larger; x = 25; y = 13; larger = Math.max(x, y); 9 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (6) 5. Math.random() needs some explanation { As indicated, it returns a value between 0.0 and 1.0, but will never be exactly one { To generate an integer between m and n, (a) Call Math.random() (b) Multiply the result by the number of possible values between m and n (c) Add m to the result { For example, to generate a value between one and six (e.g., to simulate a die roll) double result; result = Math.random(); result = 6 * result; result = 1 + result; { The general formula is result = m + (m − n + 1) ∗ Math:random() 6. Arithmetic expressions { These involve a calculation involving arithmetic operators { Unary operators (1 operand): (a) + (b) - { Binary operators (2 operands): (a) + (b) - (c) * (multiplication) (d) / (division) (e) % (remainder) 10 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (7) 7. Compound expressions { Involve more than one operator { Evaluation determined by associativity and precedence { Associativity ∗ Pertains to the direction a given operator evaluates ∗ Can be left or right { Precedence ∗ Pertains to order in which different operators are evaluated 11 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (8) { Associativity and precedence chart Operator Type Precedence Operators Associativity Subexpression 16 () Postfix increment 15 ++ L and decrement −− Prefix increment 14 ++ R and decrement −− Unary 14 ! R + - Type cast 13 (type)R Multiplicative 12 * L / % Additive 11 + L - + (string catenation) Relational 9 < L > ≤ ≥ Equality 8 == L != Boolean AND 4 && L Boolean OR 3 jj L conditional 2 ?: R Assignment 1 = R += (etc) Operator types listed from highest to lowest precedence. L indicates left-to-right; R indicates right-to-left. 12 Java Primitive Numeric Types, Assignment, and Expressions: Numeric Assignment (9) 8. Increment and decrement operators { Operators: (a) ++ (b) −− { There are actually 4 of them ∗ 2 are postfix: (a) operand + + (b) operand − − ∗ 2 are prefix: (a) + + operand (b) − − operand { Postfix semantics: (a) operand = operand ± 1 (b) return value of operand { Prefix semantics: (a) return value of operand (b) operand = operand ± 1 9. "Special" assignment operators { These are shortcuts: (a) += (b) -= (c) *= (d) /= (e) %= { They all work in the same way ∗ Each combines an arithmetic operation with assignment ∗ Semantics of variable op = value: variable = variable op value 13 Java Primitive Numeric Types, Assignment, and Expressions: Mixed-Mode Expressions and Data Conversions • Operator overloading { Expression 5 + 2 uses integer addition { Expression 7:35 + 15:333 uses floating point addition { There are actually several versions of the '+' operator This is called operator overloading { How does Java handle 23:98 + 10? • Mixed-mode expressions involve 2 different data types • In order to perform the operation, the arguments must be made compatible so Java knows which version of '+' to use • Such changes in type are called type conversions • Java provides 2 approaches: implicit and explicit • Implicit { Java performs the conversion automatically { Argument of lesser precision is converted to the type of the argument with greater precision { Unary operator algorithm: 1.
Details
-
File Typepdf
-
Upload Time-
-
Content LanguagesEnglish
-
Upload UserAnonymous/Not logged-in
-
File Pages22 Page
-
File Size-