SDL Image 3 November 2009
Total Page:16
File Type:pdf, Size:1020Kb
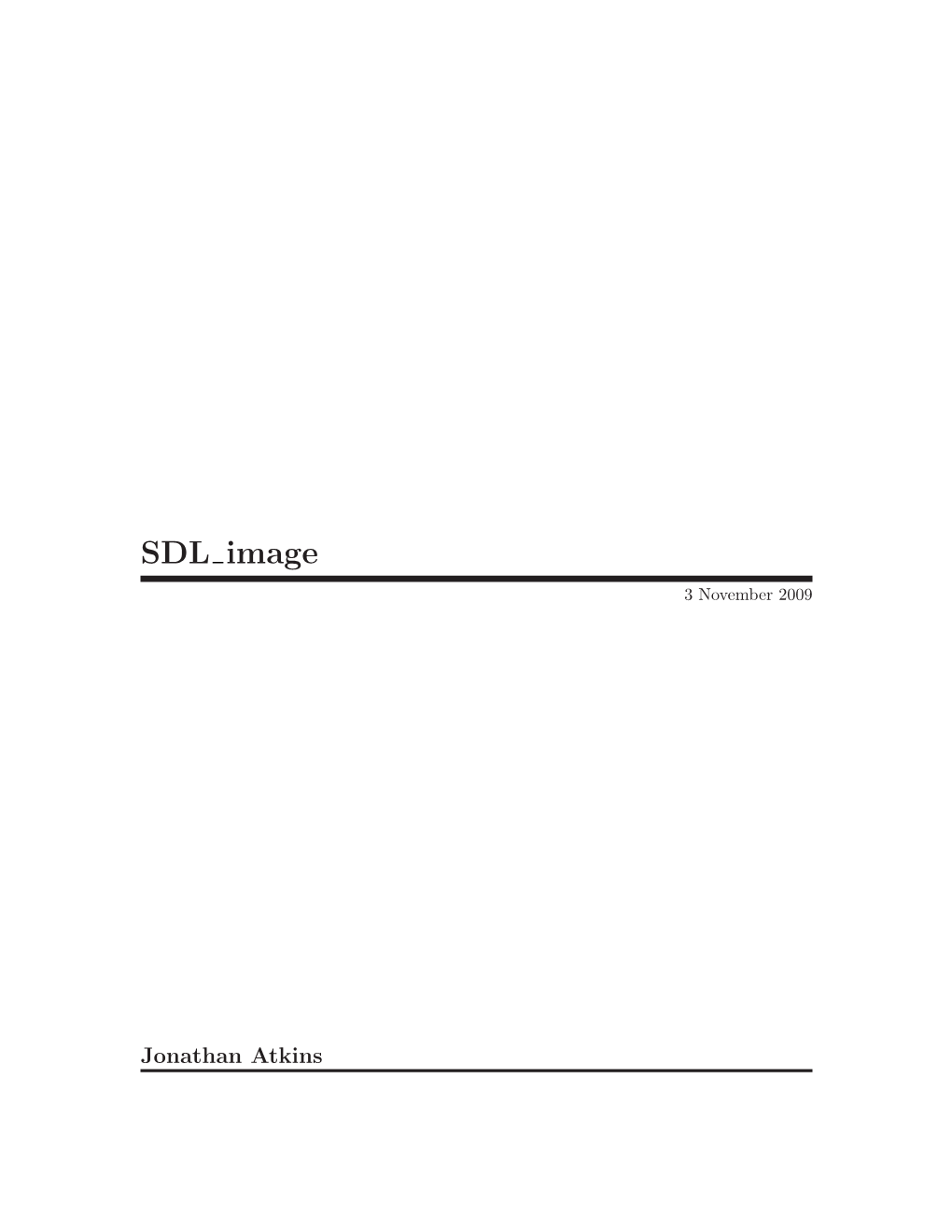
Load more
Recommended publications
-
Digital Backgrounds Using GIMP
Digital Backgrounds using GIMP GIMP is a free image editing program that rivals the functions of the basic versions of industry leaders such as Adobe Photoshop and Corel Paint Shop Pro. For information on which image editing program is best for your needs, please refer to our website, digital-photo- backgrounds.com in the FAQ section. This tutorial was created to teach you, step-by-step, how to remove a subject (person or item) from its original background and place it onto a Foto*Fun Digital Background. Upon completing this tutorial, you will have the skills to create realistic portraits plus photographic collages and other useful functions. If you have any difficulties with any particular step in the process, feel free to contact us anytime at digital-photo-backgrounds.com. How to read this tutorial It is recommended that you use your own image when following the instructions. However, one has been provided for you in the folder entitled "Tutorial Images". This tutorial uses screenshots to visually show you each step of the process. Each step will be numbered which refers to a number within the screenshot image to indicate the location of the function or tool being described in that particular step. Written instructions are first and refer to the following screenshot image. Keyboard shortcuts are shown after most of the described functions and are in parentheses as in the following example (ctrl+T); which would indicate that the "ctrl" key and the letter "T" key should be pressed at the same time. These are keyboard shortcuts and can be used in place of the described procedure; not in addition to the described procedure. -
Iriscompressor Software and to This Publication
User Guide Legal Notices ICOMP_Pro-dgi/pko-24012012-01 Copyrights Copyrights ©2011-2012 I.R.I.S. All Rights Reserved. I.R.I.S. owns the copyrights to the IRISCompressor software and to this publication. The information contained in this document is the property of I.R.I.S. Its content is subject to change without notice and does not represent a commitment on the part of I.R.I.S. The software described in this document is furnished under a license agreement which states the terms for use of this product. The software may be used or copied only in accordance with the terms of that agreement. No part of this publication may be reproduced, transmitted, stored in a retrieval system, or translated into another language without the prior written consent of I.R.I.S. Trademarks The I.R.I.S. logo and IRISCompressor are trademarks of I.R.I.S. OCR ("Optical Character Recognition"), IDR ("Intelligent Document Recognition") and iHQC ("intelligent High Quality Compression) technology by I.R.I.S. All other products mentioned in this publication are trademarks or registered trademarks of their respective owners. iHQCTM patent-protected. US Patent No. 8,068,684 B2. 1 INTRODUCTION IRISCompressor Pro is a handy compression tool that allows you to convert your image and PDF files into compressed PDF files in just a few mouse clicks. The PDF files IRISCompressor generates are fully text-searchable, thanks to I.R.I.S.' OCR technology (Optical Character Recognition). IMPORTANT NOTES IRISCompressor Pro can process multiple image and PDF files at a time. -
Seashore Guide
Seashore The Incomplete Guide Contents Contents..........................................................................................................................1 Introducing Seashore.......................................................................................................4 Product Summary........................................................................................................4 Technical Requirements ..............................................................................................4 Development Notice....................................................................................................4 Seashore’s Philosophy.................................................................................................4 Seashore and the GIMP...............................................................................................4 How do I contribute?...................................................................................................5 The Concepts ..................................................................................................................6 Bitmaps.......................................................................................................................6 Colours .......................................................................................................................7 Layers .........................................................................................................................7 Channels .................................................................................................................. -
Openimageio 1.7 Programmer Documentation (In Progress)
OpenImageIO 1.7 Programmer Documentation (in progress) Editor: Larry Gritz [email protected] Date: 31 Mar 2016 ii The OpenImageIO source code and documentation are: Copyright (c) 2008-2016 Larry Gritz, et al. All Rights Reserved. The code that implements OpenImageIO is licensed under the BSD 3-clause (also some- times known as “new BSD” or “modified BSD”) license: Redistribution and use in source and binary forms, with or without modification, are per- mitted provided that the following conditions are met: • Redistributions of source code must retain the above copyright notice, this list of condi- tions and the following disclaimer. • Redistributions in binary form must reproduce the above copyright notice, this list of con- ditions and the following disclaimer in the documentation and/or other materials provided with the distribution. • Neither the name of the software’s owners nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIB- UTORS ”AS IS” AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FIT- NESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUD- ING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABIL- ITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. -
Image Formats
Image Formats Ioannis Rekleitis Many different file formats • JPEG/JFIF • Exif • JPEG 2000 • BMP • GIF • WebP • PNG • HDR raster formats • TIFF • HEIF • PPM, PGM, PBM, • BAT and PNM • BPG CSCE 590: Introduction to Image Processing https://en.wikipedia.org/wiki/Image_file_formats 2 Many different file formats • JPEG/JFIF (Joint Photographic Experts Group) is a lossy compression method; JPEG- compressed images are usually stored in the JFIF (JPEG File Interchange Format) >ile format. The JPEG/JFIF >ilename extension is JPG or JPEG. Nearly every digital camera can save images in the JPEG/JFIF format, which supports eight-bit grayscale images and 24-bit color images (eight bits each for red, green, and blue). JPEG applies lossy compression to images, which can result in a signi>icant reduction of the >ile size. Applications can determine the degree of compression to apply, and the amount of compression affects the visual quality of the result. When not too great, the compression does not noticeably affect or detract from the image's quality, but JPEG iles suffer generational degradation when repeatedly edited and saved. (JPEG also provides lossless image storage, but the lossless version is not widely supported.) • JPEG 2000 is a compression standard enabling both lossless and lossy storage. The compression methods used are different from the ones in standard JFIF/JPEG; they improve quality and compression ratios, but also require more computational power to process. JPEG 2000 also adds features that are missing in JPEG. It is not nearly as common as JPEG, but it is used currently in professional movie editing and distribution (some digital cinemas, for example, use JPEG 2000 for individual movie frames). -
Freeware Irfanview Windows 10 Latest Version Download Freeware Irfanview Windows 10 Latest Version Download
freeware irfanview windows 10 latest version download Freeware irfanview windows 10 latest version download. Advantages of IrfanView 64-bit over 32-bit version: It can load VERY large files/images (image RAM size over 1.3 GB, for special users) Faster for very large images (25+ Megapixels, loading or image operations) Runs 'only' on a 64-bit Windows (Vista, Win7, Win8, Win10) Advantages of IrfanView 32-bit over 64-bit version: Runs on a 32-bit and 64-bit Windows Loads all files/images for normal needs (max. RAM size is about 1.3 GB) Needs less disc space All PlugIns will work: not all PlugIns are ported (yet) to 64-bit (like OCR) and some 32-bit PlugIns must be still used in the 64-bit version, some with limitations (see the "Plugins32" folder) Some old 32-bit PlugIns (like RIOT and Adobe 8BF PlugIn) work only in compatilibilty mode in IrfanView-64 ( only 32-bit 8BF files/effects can be used ) Command line options for scanning (/scan etc.) work only in 32-bit (because no 64-bit TWAIN drivers ) Notes: You can install both versions on the same system, just use different folders . For example: install the 32-bit version in your "Program Files (x86)" folder and the 64-bit version in your "Program Files" folder (install 32-bit PlugIns to IrfanView-32 and 64-bit PlugIns to IrfanView-64, DO NOT mix the PlugIns and IrfanView bit versions) The program name and icon have some extra text in the 64-bit version for better distinguishing. Available 64-bit downloads. -
File Format Guidelines for Management and Long-Term Retention of Electronic Records
FILE FORMAT GUIDELINES FOR MANAGEMENT AND LONG-TERM RETENTION OF ELECTRONIC RECORDS 9/10/2012 State Archives of North Carolina File Format Guidelines for Management and Long-Term Retention of Electronic records Table of Contents 1. GUIDELINES AND RECOMMENDATIONS .................................................................................. 3 2. DESCRIPTION OF FORMATS RECOMMENDED FOR LONG-TERM RETENTION ......................... 7 2.1 Word Processing Documents ...................................................................................................................... 7 2.1.1 PDF/A-1a (.pdf) (ISO 19005-1 compliant PDF/A) ........................................................................ 7 2.1.2 OpenDocument Text (.odt) ................................................................................................................... 3 2.1.3 Special Note on Google Docs™ .......................................................................................................... 4 2.2 Plain Text Documents ................................................................................................................................... 5 2.2.1 Plain Text (.txt) US-ASCII or UTF-8 encoding ................................................................................... 6 2.2.2 Comma-separated file (.csv) US-ASCII or UTF-8 encoding ........................................................... 7 2.2.3 Tab-delimited file (.txt) US-ASCII or UTF-8 encoding .................................................................... 8 2.3 -
Digital Photo Editing
Digital Photo Editing Digital Photo Editing There are two main catagories of photo editing software. 1. Photo Organizers - Programs that help you find your pictures. May also do some editing, and create web pages and collages. Examples: Picasa, XNView, ACDsee, Adobe Photoshop Elements 2. Photo Editors - Work on one picture file at a time. Usually more powerful editing features. Examples: Adobe Photoshop, Gimp, Paint.Net, Corel Paint Shop Photo Organizers Organizers tend to have a similar look and functionality to each other. Thumb nail views, a directory tree of your files and folders, and a slightly larger preview of the picture currently selected. A selection of the most used editing tools, and batch editing for making minor corrections to multiple pictures at once. The ability to create slide shows, contact sheets, and web pages are also features you can expect to see. XNView Picasa ACDsee Some of the editing features included in Photo Organizer software are: Red Eye Reduction, Rotate, Resize, Contrast, Color Saturation, Sharpen Focus and more. Many of these can be done in batch mode to as many pictures as you select. Picasa has added Picnik to it's tool set allowing you to upload your photo to the Picnik website for added editing features. Here is an example of Redeye removal in Picasa. Crop, Straighten, and Fill Light are often needed basic fixes. Saving and converting your picture file. In Xnview you can import about 400 file formats and export in about 50. For the complete list goto http://www.xnview. com/en/formats.html . Here is a list of some of the key file formats your likely to use and / or come across often. -
JPEG Image Compression2.Pdf
JPEG image compression FAQ, part 2/2 2/18/05 5:03 PM Part1 - Part2 - MultiPage JPEG image compression FAQ, part 2/2 There are reader questions on this topic! Help others by sharing your knowledge Newsgroups: comp.graphics.misc, comp.infosystems.www.authoring.images From: [email protected] (Tom Lane) Subject: JPEG image compression FAQ, part 2/2 Message-ID: <[email protected]> Summary: System-specific hints and program recommendations for JPEG images Keywords: JPEG, image compression, FAQ, JPG, JFIF Reply-To: [email protected] Date: Mon, 29 Mar 1999 02:24:34 GMT Sender: [email protected] Archive-name: jpeg-faq/part2 Posting-Frequency: every 14 days Last-modified: 28 March 1999 This article answers Frequently Asked Questions about JPEG image compression. This is part 2, covering system-specific hints and program recommendations for a variety of computer systems. Part 1 covers general questions and answers about JPEG. As always, suggestions for improvement of this FAQ are welcome. New since version of 14 March 1999: * Added entries for PIE (Windows digicam utility) and Cameraid (Macintosh digicam utility). * New version of VuePrint (7.3). This article includes the following sections: General info: [1] What is covered in this FAQ? [2] How do I retrieve these programs? Programs and hints for specific systems: [3] X Windows [4] Unix (without X) [5] MS-DOS [6] Microsoft Windows [7] OS/2 [8] Macintosh [9] Amiga [10] Atari ST [11] Acorn Archimedes [12] NeXT [13] Tcl/Tk [14] Other systems Source code for JPEG: [15] Freely available source code for JPEG Miscellaneous: [16] Which programs support progressive JPEG? [17] Where are FAQ lists archived? This article and its companion are posted every 2 weeks. -
R-Photo User's Manual
User's Manual © R-Tools Technology Inc 2020. All rights reserved. www.r-tt.com © R-tools Technology Inc 2020. All rights reserved. No part of this User's Manual may be copied, altered, or transferred to, any other media without written, explicit consent from R-tools Technology Inc.. All brand or product names appearing herein are trademarks or registered trademarks of their respective holders. R-tools Technology Inc. has developed this User's Manual to the best of its knowledge, but does not guarantee that the program will fulfill all the desires of the user. No warranty is made in regard to specifications or features. R-tools Technology Inc. retains the right to make alterations to the content of this Manual without the obligation to inform third parties. Contents I Table of Contents I Start 1 II Quick Start Guide in 3 Steps 1 1 Step 1. Di.s..k.. .S..e..l.e..c..t.i.o..n.. .............................................................................................................. 1 2 Step 2. Fi.l.e..s.. .M..a..r..k.i.n..g.. ................................................................................................................ 4 3 Step 3. Re..c..o..v..e..r.y.. ...................................................................................................................... 6 III Features 9 1 File Sorti.n..g.. .............................................................................................................................. 9 2 File Sea.r.c..h.. ............................................................................................................................ -
Forcepoint DLP Supported File Formats and Size Limits
Forcepoint DLP Supported File Formats and Size Limits Supported File Formats and Size Limits | Forcepoint DLP | v8.8.1 This article provides a list of the file formats that can be analyzed by Forcepoint DLP, file formats from which content and meta data can be extracted, and the file size limits for network, endpoint, and discovery functions. See: ● Supported File Formats ● File Size Limits © 2021 Forcepoint LLC Supported File Formats Supported File Formats and Size Limits | Forcepoint DLP | v8.8.1 The following tables lists the file formats supported by Forcepoint DLP. File formats are in alphabetical order by format group. ● Archive For mats, page 3 ● Backup Formats, page 7 ● Business Intelligence (BI) and Analysis Formats, page 8 ● Computer-Aided Design Formats, page 9 ● Cryptography Formats, page 12 ● Database Formats, page 14 ● Desktop publishing formats, page 16 ● eBook/Audio book formats, page 17 ● Executable formats, page 18 ● Font formats, page 20 ● Graphics formats - general, page 21 ● Graphics formats - vector graphics, page 26 ● Library formats, page 29 ● Log formats, page 30 ● Mail formats, page 31 ● Multimedia formats, page 32 ● Object formats, page 37 ● Presentation formats, page 38 ● Project management formats, page 40 ● Spreadsheet formats, page 41 ● Text and markup formats, page 43 ● Word processing formats, page 45 ● Miscellaneous formats, page 53 Supported file formats are added and updated frequently. Key to support tables Symbol Description Y The format is supported N The format is not supported P Partial metadata -
Designing and Developing a Model for Converting Image Formats Using Java API for Comparative Study of Different Image Formats
International Journal of Scientific and Research Publications, Volume 4, Issue 7, July 2014 1 ISSN 2250-3153 Designing and developing a model for converting image formats using Java API for comparative study of different image formats Apurv Kantilal Pandya*, Dr. CK Kumbharana** * Research Scholar, Department of Computer Science, Saurashtra University, Rajkot. Gujarat, INDIA. Email: [email protected] ** Head, Department of Computer Science, Saurashtra University, Rajkot. Gujarat, INDIA. Email: [email protected] Abstract- Image is one of the most important techniques to Different requirement of compression in different area of image represent data very efficiently and effectively utilized since has produced various compression algorithms or image file ancient times. But to represent data in image format has number formats with time. These formats includes [2] ANI, ANIM, of problems. One of the major issues among all these problems is APNG, ART, BMP, BSAVE, CAL, CIN, CPC, CPT, DPX, size of image. The size of image varies from equipment to ECW, EXR, FITS, FLIC, FPX, GIF, HDRi, HEVC, ICER, equipment i.e. change in the camera and lens puts tremendous ICNS, ICO, ICS, ILBM, JBIG, JBIG2, JNG, JPEG, JPEG 2000, effect on the size of image. High speed growth in network and JPEG-LS, JPEG XR, MNG, MIFF, PAM, PCX, PGF, PICtor, communication technology has boosted the usage of image PNG, PSD, PSP, QTVR, RAS, BE, JPEG-HDR, Logluv TIFF, drastically and transfer of high quality image from one point to SGI, TGA, TIFF, WBMP, WebP, XBM, XCF, XPM, XWD. another point is the requirement of the time, hence image Above mentioned formats can be used to store different kind of compression has remained the consistent need of the domain.