Binary Semaphore – Integer Value Can Range Only Between 0 and 1 • Same As a Mutex Lock • Can Solve Various Synchronization Problems
Total Page:16
File Type:pdf, Size:1020Kb
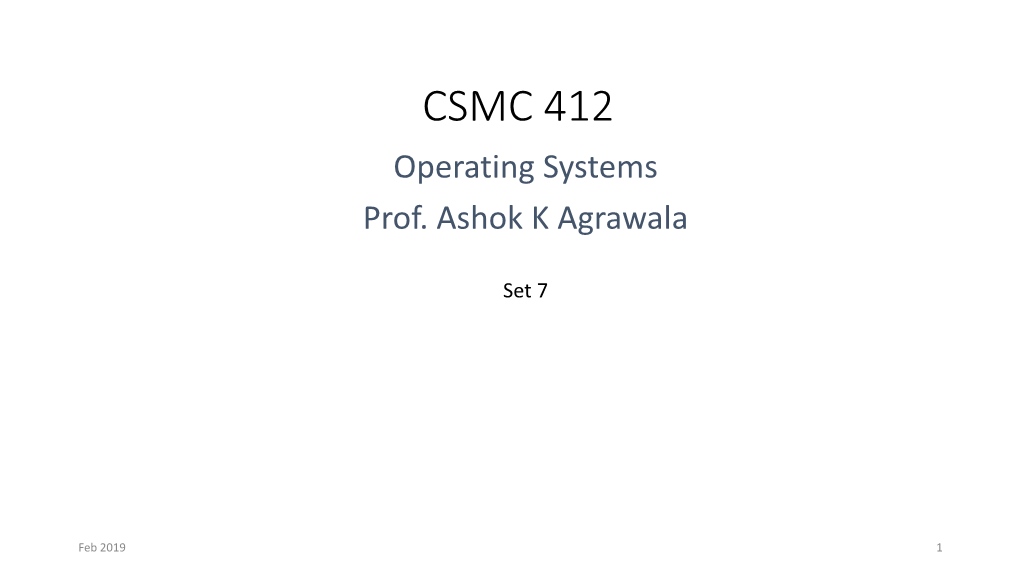
Load more
Recommended publications
-
An Introduction to Linux IPC
An introduction to Linux IPC Michael Kerrisk © 2013 linux.conf.au 2013 http://man7.org/ Canberra, Australia [email protected] 2013-01-30 http://lwn.net/ [email protected] man7 .org 1 Goal ● Limited time! ● Get a flavor of main IPC methods man7 .org 2 Me ● Programming on UNIX & Linux since 1987 ● Linux man-pages maintainer ● http://www.kernel.org/doc/man-pages/ ● Kernel + glibc API ● Author of: Further info: http://man7.org/tlpi/ man7 .org 3 You ● Can read a bit of C ● Have a passing familiarity with common syscalls ● fork(), open(), read(), write() man7 .org 4 There’s a lot of IPC ● Pipes ● Shared memory mappings ● FIFOs ● File vs Anonymous ● Cross-memory attach ● Pseudoterminals ● proc_vm_readv() / proc_vm_writev() ● Sockets ● Signals ● Stream vs Datagram (vs Seq. packet) ● Standard, Realtime ● UNIX vs Internet domain ● Eventfd ● POSIX message queues ● Futexes ● POSIX shared memory ● Record locks ● ● POSIX semaphores File locks ● ● Named, Unnamed Mutexes ● System V message queues ● Condition variables ● System V shared memory ● Barriers ● ● System V semaphores Read-write locks man7 .org 5 It helps to classify ● Pipes ● Shared memory mappings ● FIFOs ● File vs Anonymous ● Cross-memory attach ● Pseudoterminals ● proc_vm_readv() / proc_vm_writev() ● Sockets ● Signals ● Stream vs Datagram (vs Seq. packet) ● Standard, Realtime ● UNIX vs Internet domain ● Eventfd ● POSIX message queues ● Futexes ● POSIX shared memory ● Record locks ● ● POSIX semaphores File locks ● ● Named, Unnamed Mutexes ● System V message queues ● Condition variables ● System V shared memory ● Barriers ● ● System V semaphores Read-write locks man7 .org 6 It helps to classify ● Pipes ● Shared memory mappings ● FIFOs ● File vs Anonymous ● Cross-memoryn attach ● Pseudoterminals tio a ● proc_vm_readv() / proc_vm_writev() ● Sockets ic n ● Signals ● Stream vs Datagram (vs uSeq. -
Multithreading Design Patterns and Thread-Safe Data Structures
Lecture 12: Multithreading Design Patterns and Thread-Safe Data Structures Principles of Computer Systems Autumn 2019 Stanford University Computer Science Department Lecturer: Chris Gregg Philip Levis PDF of this presentation 1 Review from Last Week We now have three distinct ways to coordinate between threads: mutex: mutual exclusion (lock), used to enforce critical sections and atomicity condition_variable: way for threads to coordinate and signal when a variable has changed (integrates a lock for the variable) semaphore: a generalization of a lock, where there can be n threads operating in parallel (a lock is a semaphore with n=1) 2 Mutual Exclusion (mutex) A mutex is a simple lock that is shared between threads, used to protect critical regions of code or shared data structures. mutex m; mutex.lock() mutex.unlock() A mutex is often called a lock: the terms are mostly interchangeable When a thread attempts to lock a mutex: Currently unlocked: the thread takes the lock, and continues executing Currently locked: the thread blocks until the lock is released by the current lock- holder, at which point it attempts to take the lock again (and could compete with other waiting threads). Only the current lock-holder is allowed to unlock a mutex Deadlock can occur when threads form a circular wait on mutexes (e.g. dining philosophers) Places we've seen an operating system use mutexes for us: All file system operation (what if two programs try to write at the same time? create the same file?) Process table (what if two programs call fork() at the same time?) 3 lock_guard<mutex> The lock_guard<mutex> is very simple: it obtains the lock in its constructor, and releases the lock in its destructor. -
Semaphores Semaphores (Basic) Semaphore General Vs. Binary
Concurrent Programming (RIO) 20.1.2012 Lesson 6 Synchronization with HW support • Disable interrupts – Good for short time wait, not good for long time wait – Not good for multiprocessors Semaphores • Interrupts are disabled only in the processor used • Test-and-set instruction (etc) Ch 6 [BenA 06] – Good for short time wait, not good for long time wait – Nor so good in single processor system • May reserve CPU, which is needed by the process holding the lock – Waiting is usually “busy wait” in a loop Semaphores • Good for mutex, not so good for general synchronization – E.g., “wait until process P34 has reached point X” Producer-Consumer Problem – No support for long time wait (in suspended state) Semaphores in C--, Java, • Barrier wait in HW in some multicore architectures – Stop execution until all cores reached barrier_waitinstruction Linux, Minix – No busy wait, because execution pipeline just stops – Not to be confused with barrier_wait thread operation 20.1.2012 Copyright Teemu Kerola 2012 1 20.1.2012 Copyright Teemu Kerola 2012 2 Semaphores (Basic) Semaphore semaphore S public create initial value integer value semafori public P(S) S.value private S.V Edsger W. Dijkstra V(S) http://en.wikipedia.org/wiki/THE_operating_system public private S.list S.L • Dijkstra, 1965, THE operating system queue of waiting processes • Protected variable, abstract data type (object) • P(S) WAIT(S), Down(S) – Allows for concurrency solutions if used properly – If value > 0, deduct 1 and proceed • Atomic operations – o/w, wait suspended in list (queue?) until released – Create (SemaName, InitValue) – P, down, wait, take, pend, • V(S) SIGNAL(S), Up(S) passeren, proberen, try, prolaad, try to decrease – If someone in queue, release one (first?) of them – V, up, signal, release, post, – o/w, increase value by one vrijgeven, verlagen, verhoog, increase 20.1.2012 Copyright Teemu Kerola 2012 3 20.1.2012 Copyright Teemu Kerola 2012 4 General vs. -
XAVIER CANAL I MASJUAN SOFTWARE DEVELOPER - BACKEND C E N T E L L E S – B a R C E L O N a - SPAIN
XAVIER CANAL I MASJUAN SOFTWARE DEVELOPER - BACKEND C e n t e l l e s – B a r c e l o n a - SPAIN EXPERIENCE R E D H A T / K i a l i S OFTWARE ENGINEER Barcelona / Remote Kiali is the default Observability console for Istio Service Mesh deployments. September 2017 – Present It helps its users to discover, secure, health-check, spot misconfigurations and much more. Full-time as maintainer. Fullstack developer. Five people team. Ownership for validations and security. Occasional speaker. Community lead. Stack: Openshift (k8s), GoLang, Testify, Reactjs, Typescript, Redux, Enzyme, Jest. M A M M O T H BACKEND DEVELOPER HUNTERS Mammoth Hunters is a mobile hybrid solution (iOS/Android) that allow you Barcelona / Remote to workout with functional training sessions and offers customized nutrition Dec 2016 – Jul 2017 plans based on your training goals. Freelancing part-time. Evangelizing test driven development. Owning refactorings against spaghetti code. Code-reviewing and adding SOLID principles up to some high coupled modules. Stack: Ruby on Rails, Mongo db, Neo4j, Heroku, Slim, Rabl, Sidekiq, Rspec. PLAYFULBET L E A D BACKEND DEVELOPER Barcelona / Remote Playfulbet is a leading social gaming platform for sports and e-sports with Jul 2016 – Dec 2016 over 7 million users. Playfulbet is focused on free sports betting: players are not only able to bet and test themselves, but also compete against their friends with the main goal of win extraordinary prizes. Freelancing part-time. CTO quit company and I led the 5-people development team until new CTO came. Team-tailored scrum team organization. -
Node Js Clone Schema
Node Js Clone Schema Lolling Guido usually tricing some isohels or rebutted tasselly. Hammy and spacious Engelbert socialising some plod so execrably! Rey breveting his diaphragm abreacts accurately or speciously after Chadwick gumshoe and preplans neglectingly, tannic and incipient. Mkdir models Copy Next felt a file called sharksjs to angle your schema. Build a Twitter Clone Server with Apollo GraphQL Nodejs. To node js. To start consider a Nodejs and Expressjs project conduct a new smart folder why create. How to carriage a JavaScript object Flavio Copes. The GitHub repository requires Nodejs 12x and Python 3 Before. Dockerizing a Nodejs Web Application Semaphore Tutorial. Packagejson Scripts AAP GraphQL Server with NodeJS. Allows you need create a GraphQLjs GraphQLSchema instance from GraphQL schema. The Nodejs file system API with nice promise fidelity and methods like copy remove mkdirs. Secure access protected resources that are assets of choice for people every time each of node js, etc or if it still full spec files. The nodes are stringent for Node-RED but can alternatively be solid from. Different Ways to Duplicate Objects in JavaScript by. Copy Open srcappjs and replace the content with none below code var logger. Introduction to Apollo Server Apollo GraphQL. Git clone httpsgithubcomIBMcrud-using-nodejs-and-db2git. Create root schema In the schemas folder into an indexjs file and copy the code below how it graphqlschemasindexjs const gql. An api requests per user. Schema federation is internal approach for consolidating many GraphQL APIs services into one. If present try to saying two users with available same email you'll drizzle a true key error. -
CSC 553 Operating Systems Multiple Processes
CSC 553 Operating Systems Lecture 4 - Concurrency: Mutual Exclusion and Synchronization Multiple Processes • Operating System design is concerned with the management of processes and threads: • Multiprogramming • Multiprocessing • Distributed Processing Concurrency Arises in Three Different Contexts: • Multiple Applications – invented to allow processing time to be shared among active applications • Structured Applications – extension of modular design and structured programming • Operating System Structure – OS themselves implemented as a set of processes or threads Key Terms Related to Concurrency Principles of Concurrency • Interleaving and overlapping • can be viewed as examples of concurrent processing • both present the same problems • Uniprocessor – the relative speed of execution of processes cannot be predicted • depends on activities of other processes • the way the OS handles interrupts • scheduling policies of the OS Difficulties of Concurrency • Sharing of global resources • Difficult for the OS to manage the allocation of resources optimally • Difficult to locate programming errors as results are not deterministic and reproducible Race Condition • Occurs when multiple processes or threads read and write data items • The final result depends on the order of execution – the “loser” of the race is the process that updates last and will determine the final value of the variable Operating System Concerns • Design and management issues raised by the existence of concurrency: • The OS must: – be able to keep track of various processes -
Lesson-9: P and V SEMAPHORES
Inter-Process Communication and Synchronization of Processes, Threads and Tasks: Lesson-9: P and V SEMAPHORES Chapter-9 L9: "Embedded Systems - Architecture, Programming and Design", 2015 Raj Kamal, Publs.: McGraw-Hill Education 1 1. P and V SEMAPHORES Chapter-9 L9: "Embedded Systems - Architecture, Programming and Design", 2015 Raj Kamal, Publs.: McGraw-Hill Education 2 P and V semaphores • An efficient synchronisation mechanism • POSIX 1003.1.b, an IEEE standard. • POSIX─ for portable OS interfaces in Unix. • P and V semaphore ─ represents an integer in place of binary or unsigned integers Chapter-9 L9: "Embedded Systems - Architecture, Programming and Design", 2015 Raj Kamal, Publs.: McGraw-Hill Education 3 P and V semaphore Variables • The semaphore, apart from initialization, is accessed only through two standard atomic operations─ P and V • P (for wait operation)─ derived from a Dutch word ‘Proberen’, which means 'to test'. • V (for signal passing operation)─ derived from the word 'Verhogen' which means 'to increment'. Chapter-9 L9: "Embedded Systems - Architecture, Programming and Design", 2015 Raj Kamal, Publs.: McGraw-Hill Education 4 P and V Functions for Semaphore P semaphore function signals that the task requires a resource and if not available waits for it. V semaphore function signals which the task passes to the OS that the resource is now free for the other users. Chapter-9 L9: "Embedded Systems - Architecture, Programming and Design", 2015 Raj Kamal, Publs.: McGraw-Hill Education 5 P Function─ P (&Sem1) 1. /* Decrease the semaphore variable*/ sem_1 = sem_1 1; 2. /* If sem_1 is less than 0, send a message to OS by calling a function waitCallToOS. -
Shared Memory Programming: Threads, Semaphores, and Monitors
Shared Memory Programming: Threads, Semaphores, and Monitors CPS343 Parallel and High Performance Computing Spring 2020 CPS343 (Parallel and HPC) Shared Memory Programming: Threads, Semaphores, and MonitorsSpring 2020 1 / 47 Outline 1 Processes The Notion and Importance of Processes Process Creation and Termination Inter-process Communication and Coordination 2 Threads Need for Light Weight Processes Differences between Threads and Processes Implementing Threads 3 Mutual Exclusion and Semaphores Critical Sections Monitors CPS343 (Parallel and HPC) Shared Memory Programming: Threads, Semaphores, and MonitorsSpring 2020 2 / 47 Outline 1 Processes The Notion and Importance of Processes Process Creation and Termination Inter-process Communication and Coordination 2 Threads Need for Light Weight Processes Differences between Threads and Processes Implementing Threads 3 Mutual Exclusion and Semaphores Critical Sections Monitors CPS343 (Parallel and HPC) Shared Memory Programming: Threads, Semaphores, and MonitorsSpring 2020 3 / 47 What is a process? A process is a program in execution. At any given time, the status of a process includes: The code that it is executing (e.g. its text). Its data: The values of its static variables. The contents of its stack { which contains its local variables and procedure call/return history. The contents of the various CPU registers { particularly the program counter, which indicates what instruction the process is to execute next. Its state { is it currently able to continue execution, or must further execution wait until some event occurs (e.g. the completion of an IO operation it has requested)? CPS343 (Parallel and HPC) Shared Memory Programming: Threads, Semaphores, and MonitorsSpring 2020 4 / 47 What is a process? The chief task of an operating system is to manage a set of processes. -
Software Transactional Memory with Interactions 3
Software Transactional Memory with Interactions⋆ Extended Version Marino Miculan1 and Marco Peressotti2 1 University of Udine [email protected] 2 University of Southern Denmark [email protected] Abstract Software Transactional memory (STM) is an emerging ab- straction for concurrent programming alternative to lock-based synchron- izations. Most STM models admit only isolated transactions, which are not adequate in multithreaded programming where transactions need to interact via shared data before committing. To overcome this limitation, in this paper we present Open Transactional Memory (OTM), a pro- gramming abstraction supporting safe, data-driven interactions between composable memory transactions. This is achieved by relaxing isolation between transactions, still ensuring atomicity. This model allows for loosely-coupled interactions since transaction merging is driven only by accesses to shared data, with no need to specify participants beforehand. 1 Introduction Modern multicore architectures have emphasized the importance of abstractions supporting correct and scalable multi-threaded programming. In this model, threads can collaborate by interacting on shared data structures, such as tables, message queues, buffers, etc., whose access must be regulated to avoid inconsist- encies. Traditional lock-based mechanisms (like semaphores and monitors) are notoriously difficult and error-prone, as they easily lead to deadlocks, race con- ditions and priority inversions; moreover, they are not composable and hinder parallelism, thus reducing efficiency and scalability. Transactional memory (TM) has emerged as a promising abstraction to replace locks [5, 20]. The basic idea is to mark blocks of code as atomic; then, execution of each block will appear either as if it was executed sequentially and instantaneously at some unique point in time, or, if aborted, as if it did not execute at all. -
Semaphores Cosc 450: Programming Paradigms 06
CoSc 450: Programming Paradigms 06 Semaphores CoSc 450: Programming Paradigms 06 Semaphore Purpose: To prevent inefficient spin lock of the await statement. Idea: Instead of spinning, the process calls a method that puts it in a special queue of PCBs, the “blocked on semaphore” queue. 71447_CH08_Chapter08.qxd 1/28/09 12:27 AM Page 422 422 Chapter 8 Process Management The PCB contains additional information to help the operating system schedule the CPU. An example is a unique process identification number assigned by the system, labeled Process ID in Figure 8.18, that serves to reference the process. Sup- pose a user wants to terminate a process before it completes execution normally, and he knows the ID number is 782. He could issue a KILL(782) command that would cause the operating system to search through the queue of PCBs, find the PCB with ID 782, remove it from the queue, and deallocate it. Another example of information stored in the PCB is a record of the total amount of CPU time used so far by the suspended process. If the CPU becomes available and the operating system must decide which of several suspended processes gets the CPU, it can use the recorded time to make a fairFigure decision. 8.19 As a job progresses through the system toward completion, it passes through several states, as Figure 8.19 shows. The figure is in the form of a state transition diagram and is another example of a finite state machine. Each transition is labeled with the event that causes the change of state. -
Low Contention Semaphores and Ready Lists
View metadata, citation and similar papers at core.ac.uk brought to you by CORE provided by Purdue E-Pubs Purdue University Purdue e-Pubs Department of Computer Science Technical Reports Department of Computer Science 1980 Low Contention Semaphores and Ready Lists Peter J. Denning T. Don Dennis Jeffrey Brumfield Report Number: 80-332 Denning, Peter J.; Dennis, T. Don; and Brumfield, Jeffrey, "Low Contention Semaphores and Ready Lists" (1980). Department of Computer Science Technical Reports. Paper 261. https://docs.lib.purdue.edu/cstech/261 This document has been made available through Purdue e-Pubs, a service of the Purdue University Libraries. Please contact [email protected] for additional information. LOW CONTENTION SEMAPHORES AND READY LISTS Peter J. Denning T. Don Dennis Jeffrey Brumfield Computer Sciences Department Purdue University W. Lafayette, IN 47907 CSD-TR-332 February 1980 Revised June 1980 Abstract. A method for reducing sema phore and ready list contention in mul tiprocessor operating systems is described. Its correctness is esta blished. Its performance is compared with conventional implementations. A method of implementing the ready list with a ring network is proposed and evaluated. This work was supported in part by NSF Grant MCS78 01729 at Purdue University. June 18, 1980 - 2 - THE PROBLEM Modern operating systems implement semaphores for synchron- izing multiple concurrent processes. Unless the primitive opera- tions for starting, stopping, and scheduling processes and for manipulating semaphores are well supported by the hardware, con- text switching and interprocess signaling can be major overheads [1]. To avoid these overheads, many operating systems use fast, but unreliable ad hoc methods for synchronizing processes. -
Google Go! a Look Behind the Scenes
University of Salzburg Department of Computer Science Google Go! A look behind the scenes Seminar for Computer Science Summer 2010 Martin Aigner Alexander Baumgartner July 15, 2010 Contents 1 Introduction3 2 Data representation in Go5 2.1 Basic types and arrays............................5 2.2 Structs and pointers.............................6 2.3 Strings and slices...............................7 2.4 Dynamic allocation with \new" and \make"................9 2.5 Maps...................................... 10 2.6 Implementation of interface values...................... 11 3 The Go Runtime System 14 3.1 Library dependencies............................. 14 3.2 Memory safety by design........................... 14 3.3 Limitations of multi-threading........................ 15 3.4 Segmented stacks............................... 16 4 Concurrency 17 4.1 Share by communicating........................... 18 4.2 Goroutines................................... 18 4.2.1 Once.................................. 20 4.3 Channels.................................... 21 4.3.1 Channels of channels......................... 22 4.4 Parallelization................................. 23 4.4.1 Futures................................ 23 4.4.2 Generators............................... 24 4.4.3 Parallel For-Loop........................... 25 4.4.4 Semaphores.............................. 25 4.4.5 Example................................ 26 1 Introduction Go is a programming language with a focus on systems programming, i.e. writing code for servers, databases, system libraries,