Polygon Game Software
Total Page:16
File Type:pdf, Size:1020Kb
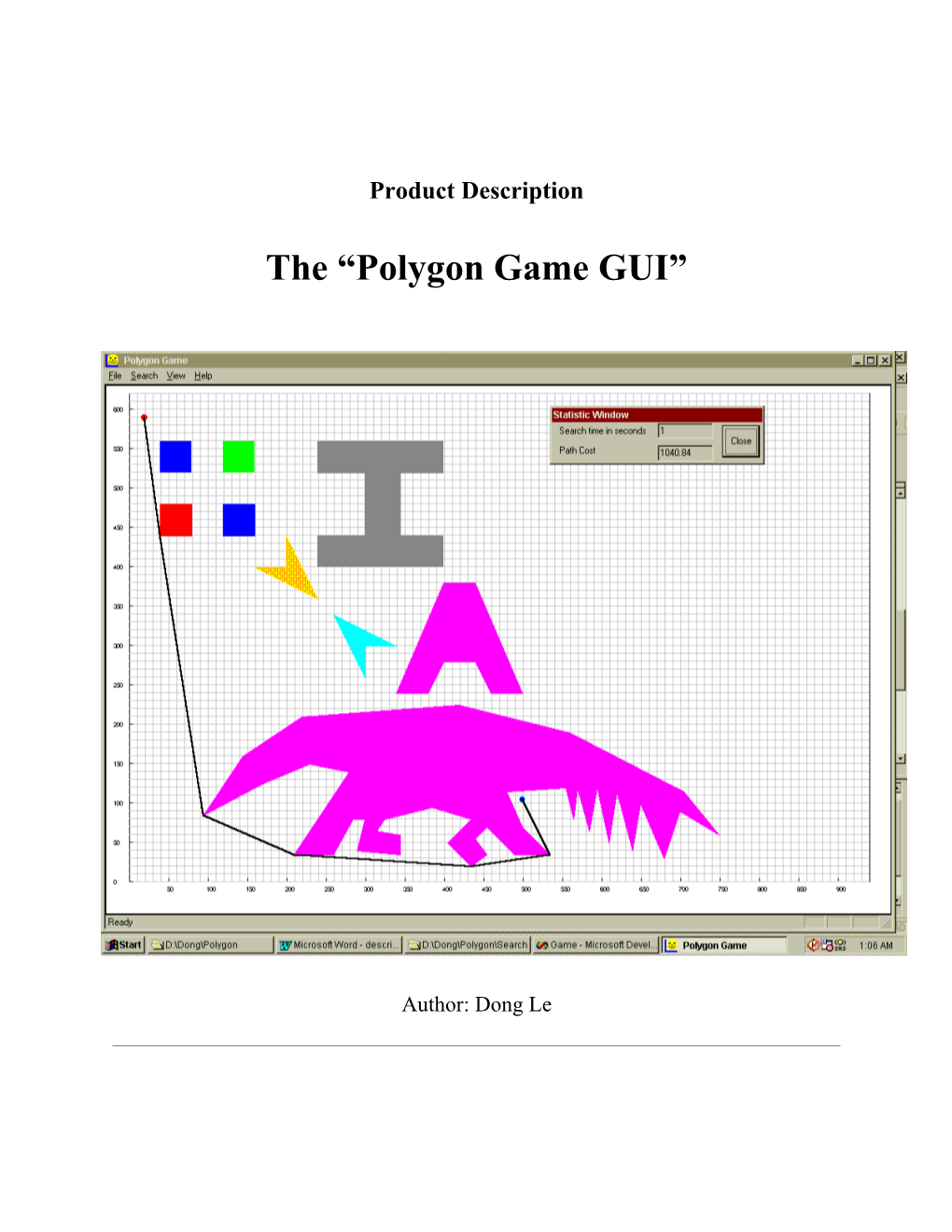
Product Description
The “Polygon Game GUI”
Author: Dong Le Table of Contents
Page
1. INTRODUCTION...... 2
2. PROBLEM DEFINITION...... 2
3. BEFORE USING THE SOFTWARE...... 2
3.1. STORE SOURCE CODE FILES IN THE CORRECT FOLDER...... 2 3.2. THE APPLICATION PROGRAM INTERFACE (API)...... 3 3.3. THE TWO FUNCTIONS: RETURNPATH() AND GETNUMBEROFPOINTS()...... 3 3.4. UNDERSTANDING THE DATA STRUCTURE...... 3 3.5. INPUT FILE FORMAT...... 3 4. THE SOFTWARE OVERVIEW...... 3
4.1. THE “POLYGON” WORKING FOLDER...... 3 4.2. THE APPLICATION PROGRAM INTERFACE – API.H...... 4 4.3. FUNCTIONS “RETURNPATH” AND “GETNUMBEROFPOINTS” IN “SEARCH.H”...... 5 4.4. DATA STRUCTURE...... 5 4.5. INPUT FORMAT...... 6 APPENDIX A...... 8
APPENDIX B...... 9
APPENDIX C...... 10
APPENDIX D...... 11
APPENDIX E...... 12
2 1. INTRODUCTION One of the project in “Introduction to Artificial intelligence, ICS171” is “Polygon Game,” a project demonstrating AI search techniques taught in the course. This Software “Polygon Game GUI” is written in Visual C++ and MFC for the purpose of providing application tools to developers of the project. Using features in MFC, the software provides a basic GUI to visualize graphic on the screen, making the work easier and more fun for its users.
2. PROBLEM DEFINITION The “Polygon Game” project in ICS171 is asking students to implement search techniques for finding paths from a start point to a goal point, through a maze consisting of non-overlapped polygons, without crossing any of the polygons. The co-ordinates of these start point, goal point, and points on the polygons are read from a text file. This “Polygon Game GUI” software will provide a graphical user interface for the project. The main window with its menu allows users to open file for input and run the search algorithm to find the path. Information on the start-point, the end-point, and all the polygons read in from a file are stored in the program’s domain data structure, and displayed to the screen. At any time, the developers can query these data for their needs. When a path is found, it is also display on the screen, along with a statistic window showing the search time and the path’s length. One difficult task among others in the project is to determine if there is a clear path between any pair of points in the maze. (A clear path between two points is a straight line connecting them without crossing any edge or touching any other point other than these two points themselves). However, in order to make the work easier, this software will provide a function to be used for this specific task. At any time when calling the function provided by the software, developers can check for a visible path between any two points in the maze.
3. BEFORE USING THE SOFTWARE The following are important things a developer should know when using this product.
3.1. Store source code files in the correct folder The software was developed using Visual C++ and MFC under a workspace called Game.dsw. The main directory for the project is called Polygon. Source codes to run the project are saved under the polygon directory. Under this folder, there are two important subdirectories called Includes and Search. The Includes directory contains included files on data structure and the file API.h, which described in sections 3.2, 3.4, and 3.5. The Search directory contains the file Search.h with the two functions returnPath() and getNumberOfPoints() described in section 3.3. Developers should save all their source code files in the folder Search, and rebuild the whole program before running the program.
3 3.2. The Application Program Interface (API) The software provides an API to its users in the file API.h. This package provides an interface which allows several function calls, including querying data on the start point, the goal point, and the polygons in the maze. To use the API, the file API.h needs to be included in the source code. To include this file, type #include”..\\Polygon\\Includes\\API.h.” It is through this package that developers can make call to the function isVisibility() to check for a clear path between any two points in the maze. For a detailed description of the API, please refer to section 4.2.
3.3. The Two Functions: returnPath() and getNumberOfPoints() When the search option from the menu is selected, the software will invoke two functions called returnPath and getNumberOfPoints. The prototypes of those two functions are defined in the file Search.h. Developers need to provide the code of these two functions for the program to work. Search.h is stored under the Polygon folder. For a detail on the requirements of returnPath and getNumberOfPoints, please refer to section 4.3.
3.4. Understanding the Data Structure It is important to understand the Data Structure used in the software. Developers need to understand the data structure of the class Vertex in order to write the function returnPath. In addition, developers might also want to understand the data structures of the class Edge and the class Polygon. For a detail on data structures, please refer to section 4.4.
3.5. Input File Format All input files to be read by the program must be in a correct format. A correct input file will include xy-coordinates for a start point, a goal point, and points for polygons if there is any of them. For a detail description of the input file format, please refer to section 4.5.
4. THE SOFTWARE OVERVIEW This section describes the Working Folder and the Work Space, the Application Program Interface, the two functions returnPath() and getNumberOfPoints(), the Data Structure, and the Input Format of the Polygon Project.
4.1. The Polygon Working Folder All source codes and related files to the program are stored in the folder Polygon. Included files to the main program are store under the folder Includes. Search.h is stored under the folder Search. Developers should save all their additional source codes in the folder Search to separate their files with all other existing files. The Polygon folder also contains a sub folder called TestFiles where developers can find several examples of input-files for testing the program. Figure 1 on next page shows a picture of the folder Polygon.
4 Figure 1. Polygon folder
4.2. The Application Program Interface – API.h The API provides seven functions as follow: (1) bool isVisible() (2) const Vertex * getStart() (3) const Vertex * getGoal() (4) int getNumberOfVertices() (5) const Vertex * getVertexArray() (6) int getNumberOfPolygons() (7) const Polygon * getPolygonArray() Each of the functions is described in following table: Function name Description Return Type Parameters IsVisible Check for a clear path between vertex A Bool 1.const Vertex A and vertex B. Return true if there is a 2.const Vertex B clear path, otherwise false. GetStart Return a pointer to the constant start Const Vertex None vertex in the maze. Users are not allowed to modify this vertex. However, users can make a copy of this vertex for his/her own use. GetGoal Return a pointer to the constant goal Const Vertex None vertex in the maze. Users are not allowed to modify this vertex. However, users can make a copy of this vertex for
5 his/her own use.
GetNumberOfVertices Return the numbers of all vertices in the Int None maze. GetVertexArray Return a pointer to the constant array Const Vertex* None holding all vertices in the maze. Users are not allowed to modify the content of this array. GetNumberOfPolygons Return the numbers of all polygons in Int None the maze. GetPolygonArray Return a pointer to the constant array Const polygon* None holding all polygons in the maze. Users are not allowed to modify the content of this array. The implementation of this API in C++ is enclosed in appendix A.
4.3. Functions “returnPath” and “getNumberOfPoints” in Search.h The file Search.h under the Polygon folder has two important functions to be described as follow:
(1) returnPath: Syntax: const Vertex * returnPath(); Description: This function will return a pointer to the constant array holding vertices of the path form start to goal in the maze. For the testing purpose, developers will find this function is currently returning a dummy path consisting of five vertices. The program will use this information to draw the path on the screen. Developers need to modify this function in order to make it returning a correct path. (2) getNumberOfPoints: Syntax: int getNumberOfPoints(); Description: This function will return the numbers of total vertices in the path. For the testing purpose, developers will find this function is currently returning the value five. The program needs this information to draw the path on the screen. Developers need to modify this function in order to make it returning a correct number. The file Search.h is enclosed in appendix B. 4.4. Data Structure The main four data structures, used in the program to store information on the start point, the goal point and the polygons in the maze, are:
(3) class Vertex Object instantiated from this class will have these properties: int x x-coordinate of this vertex
6 int y y-coordinate of this vertex int PLG the number indicates what polygon this vertex belongs to. This number equals zero if this vertex is either the start point or the goal point. The declaration of this class in C++ is enclosed in appendix C. (4) class Edge Object instantiated from this class will have these properties: Vertex Start the start point of this Edge Vertex End the end point of this Edge The declaration of this class in C++ is enclosed in appendix D. (5) class Polygon Object instantiated from this class will have these properties: int TotalEdges the numbers of total edges / vertices in this polygon Vertex * vList list of all vertices in this polygon Edge * eList list of all edges in this polygon int color the color of this polygon being displayed on the screen, where 0=Red, 1=Blue, 2=Green, 3=Orange, 4=Magenta, 5= Cyan, and others=Gray. The declaration of this class in C++ is enclosed in appendix E. 4.5. Input Format A point in the plane presented by its x- and y-coordinators enclosed in parentheses. Example: (25, 40) presents the point with x-coordinate 25 and y-coordinate 40. A polygon in the plane presented by a series of all points in it following the counter-clockwise order. A polygon is terminated by a color code as shown below: c0 Red c1 Blue c2 Green c3 Orange c4 Magenta c5 Cyan Any number other than the above means the color is gray, e.g. c12 = color is gray. Example: (12,8) (24,8) (24,11) (12,11) c1 present a blue polygon with 4 points. All input files to the program will have the following format: Start with the start point in the format described above. The goal point may stay on the same line or on the next line. All Polygons must end with a color code as described above. Note: If there is at least one polygon in the maze, the file must contain a start point and a goal point.
7 Input file example: The following input file will make the screen display shown in figure 2: (36,4) (19,19) (5,7) (13,4) (7,16) c0 (12,8) (24,8) (24,11) (12,11) c1 (26,5) (35,13) (26,11) c4 (21,13) (32,13) (32,21) (21,21) c2 (9,15) (18,15) (18,21) (9,21) c3 (5,12) (5,25) (0,15) c4 (8,22) (15,30) (5,28) c5 (15,23) (23,23) (23,28) (15,28) c3 (37,17) (36,27) (23,30) c6
Goal
Start
Figure 2. The screen display after the program read the above input file More examples on input files can be found in the sub folder “TestFiles” inside the “Polygon” folder.
8 APPENDIX A The Application Program Interface - API.h
#if !defined(API_H) #define API_H
#include "Global.h"
///////////////////// THE APPLICATION PROGRAM INTERFACE ///////////////////// // Return true if there are a clear path between the two vertices v1 & v2 bool isVisible(const Vertex* v1, const Vertex* v2) { return theMaze->isVisible(v1,v2); }
// Return a pointer to the constant start vertex. Users are not allowed to modify this constant vertex. // However, users can make a copy of this vertex for their uses. const Vertex * getStart() { return theMaze->getStart(); }
// Return a pointer to the constant start vertex. Users are not allowed to modify this constant vertex. // However, users can make a copy of this vertex for their uses. const Vertex * getGoal() { return theMaze->getGoal(); }
// Return the total number of vertices in the maze int getNumberOfVertices() { return theMaze->getNumberOfVertices(); }
// Return a pointer to the constant dynamic array holding all vertices in the maze. User are not allowed to modify // the content of the array. const Vertex * getVertexArray() { return theMaze->getVertexArray(); }
// Return the total number of polygons in the maze int getNumberOfPolygons() { return theMaze->getNumberOfPolygons(); }
// Return a pointer to the constant dynamic array holding all polygons in the maze. User are not allowed to modify // the content of the array. const polygon * getPolygonArray() { return theMaze->getPolygonArray(); } ////////////////////////////////////////////////////////////////////////////// #endif; 9 APPENDIX B Search.h
#ifndef SEARCH_CPP #define SEARCH_CPP
#include "..\\Polygon\\Includes\\Polygon.cpp"
///////////////////////////////// Here is the example code /////////////////////////////////////// // You should delete the code below and call your search algorithm. /////// // One good way to do that is placing your code in other files and include them here so that you can make use of them
class Search {private: Vertex * pathArr; int numberOfPoints; public: Search() { pathArr = new Vertex[5]; for (int i = 0; i < 5; i++) pathArr[i] = Vertex(i*22, i*14 - i%2 * 6, 0); numberOfPoints = 5; }
~Search() { if (pathArr) delete []pathArr; }
const Vertex * returnPath() const { return pathArr; }
int getNumberOfPoints() { return numberOfPoints; } };
Search* s = new Search(); /////////////////////////////////////////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////////////////////////////////////////// ///Remark: You have to provide the code for the two functions /// returnPath() and getNumberOfPoints() //////////////////////////////////////////////////////////////////////////// // These two functions are required for the program to run. You must provide your code for each of them. const Vertex * returnPath() { //The line below makes use of the example code above. Replace it with your real code return s->returnPath(); }
int getNumberOfPoints() { //The line below makes use of the example code above. Replace it with your real code return s->getNumberOfPoints(); } //////////////////////////////////////////////////////////////////////////// #endif
10 APPENDIX C Vertex.h
#ifndef VERTEX_H #define VERTEX_H
#include
//operator overloading functions const Vertex & operator= (const Vertex &rhs); int operator== (const Vertex &rhs) const; int operator!= (const Vertex &rhs) const; private: int x; //x-coordinate int y; //y-coordinate int PLG; //the polygon# this vertex belongs to }; #endif 11 APPENDIX D Edge.h
#ifndef EDGE_H #define EDGE_H
#include "Vertex.h" #include "Const.h" /*------|| || Class Edge || || Member Data - Access through member funtions only: || || Vertex Start Start point of the edge || || Vertex End End point of the edge || || Methods Provide: || || Edge(); || || Edge(const Vertex&, const Vertex&) || || ~Edge() || || getStart() Access start point || || getEnd() Access end point || || getMidPoint() Get midpoint of the edge || || getReverse() Get the reverse edge || || getLength() Get the edge's length || || setStart(const Vertex &) Change start point || || setStart(int, int) Change start point || || setEnd(const Vertex &) Change end point || || setEnd(int, int) Change end point || || isIntersect(const Edge &) does the pass-in edge cross this edge? || || operator =, operator ==, operator != || ||------*/ class Edge {public: Edge() { }; Edge(const Vertex &a, const Vertex &b) : Start(a), End(b) { }; ~Edge() { Start.~Vertex(); End.~Vertex(); };
void enLarge(int enLargeFactor); const Vertex getStart() const; const Vertex getEnd() const; const Vertex getMidPoint() const; const Edge getReverse() const; double getLength() const; //Return the length of this object Edgev void setStart(const Vertex &v); void setStart(int x, int y, int p); void setEnd(const Vertex &v); void setEnd(int x, int y, int p); //isIntersect receive an edge as its parameter and check to see whether or not this edge will intersect the //current object Edge in one or the other ways. Return true if intersect, return false otherwise. bool isIntersect(const Edge & PQ, int skew) const; const Edge & operator= (const Edge &rhs); int operator== (const Edge &rhs) const; int operator!= (const Edge &rhs) const; private: Vertex Start; //start point of the Edge Vertex End; //end point of the Edge bool isParalell(const Edge & PQ) const; }; #endif; 12 APPENDIX E Polygon.cpp
#ifndef POLYGON_CPP #define POLYGON_CPP
#include "Edge.cpp"
//////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// class polygon { public: polygon() { TotalEdges = 0; vList = NULL; eList = NULL; color = 6;} polygon(Vertex * array, int n, int color); polygon(const polygon *); ~polygon(); void draw(CDC*) const;
const polygon & polygon::operator= (const polygon &rhs);
int TotalEdges; Vertex * vList; // List of all nodes in the polygon Edge * eList; // List of all edges in the polygon int color; };
13