Ball Bounce Simulation Program
Total Page:16
File Type:pdf, Size:1020Kb
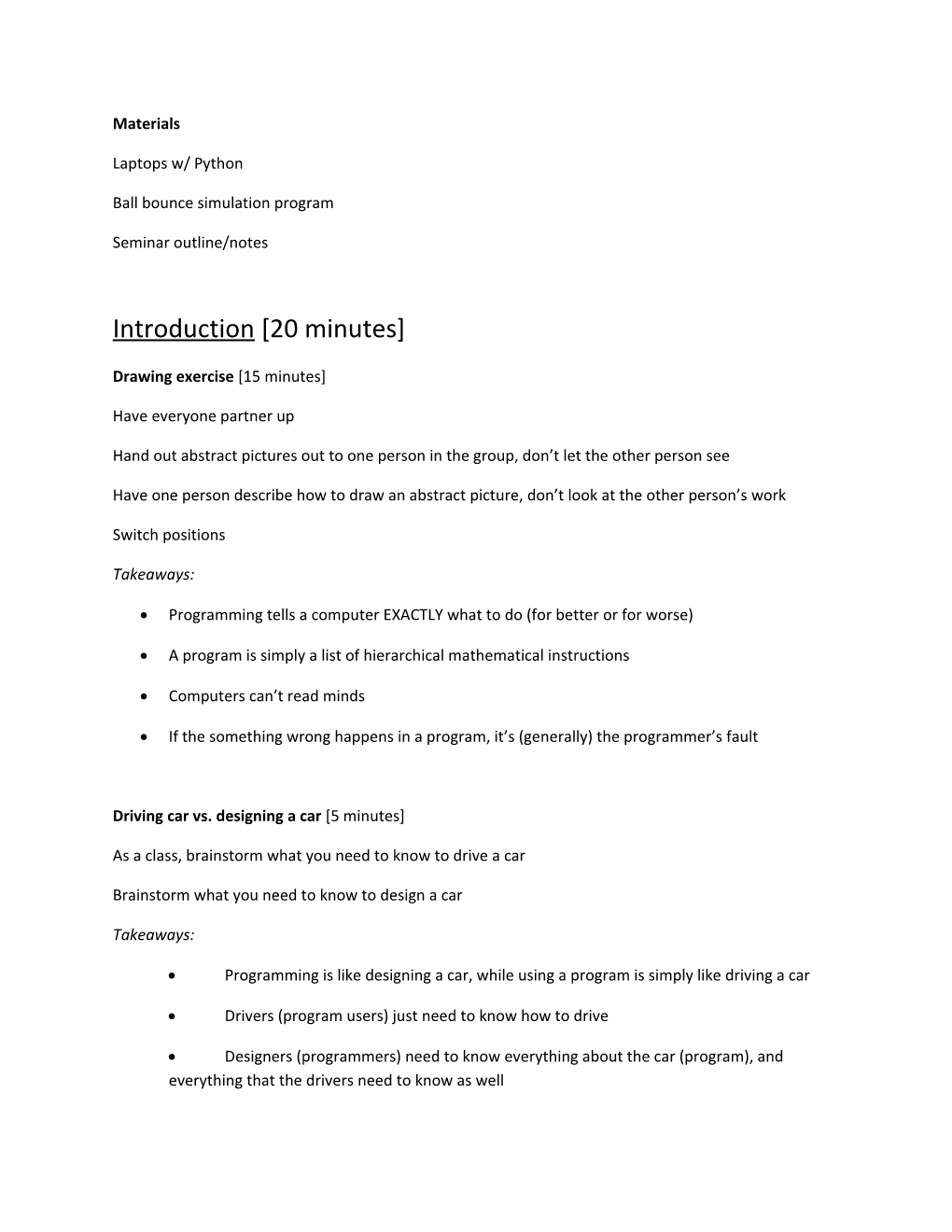
Materials
Laptops w/ Python
Ball bounce simulation program
Seminar outline/notes
Introduction [20 minutes]
Drawing exercise [15 minutes]
Have everyone partner up
Hand out abstract pictures out to one person in the group, don’t let the other person see
Have one person describe how to draw an abstract picture, don’t look at the other person’s work
Switch positions
Takeaways:
Programming tells a computer EXACTLY what to do (for better or for worse)
A program is simply a list of hierarchical mathematical instructions
Computers can’t read minds
If the something wrong happens in a program, it’s (generally) the programmer’s fault
Driving car vs. designing a car [5 minutes]
As a class, brainstorm what you need to know to drive a car
Brainstorm what you need to know to design a car
Takeaways:
Programming is like designing a car, while using a program is simply like driving a car
Drivers (program users) just need to know how to drive
Designers (programmers) need to know everything about the car (program), and everything that the drivers need to know as well Would you want to drive a car if the designer had no concept of a brake?
Programming Concepts [75 minutes]
Introduce programming languages, Python [10 minutes]
A programming language is exactly how it sounds: a language of ‘talking’ with a computer
Many programming languages exist, some are better at some things than others
C – can basically do anything you want with the computer, slow to make a useful program
Php – REALLY GOOD at making websites. Not good at much else.
Mindstorms – REALLY GOOD at controlling Legos. Not good at much else. Wouldn’t make sense to program a website in Mindstorms, or to program Legos with Php.
Python – More general purpose language. Great at doing calculations, simulations, modeling. Can program things very quickly, but doesn’t run as fast as other languages.
Code vs program [10 minutes]
Open IDLE
File -> New Window
>>> is where the program output prints
The empty screen is where the code goes print “Hello, world!”
F5 to run
You are now a programmer!
Variables [10 minutes]
Just like in algebra: x=5
Any time you use ‘x’, you replace it with the number 5
Side note: variables are one word/number; python IS case sensitive! Exercise: x=5 y=10 z=x+y
Run program
What happened? What did you expect to happen?
You have to explicitly tell the computer what to do.
Try again:
Add “print z” to the bottom of the code. Run the program again.
Can also do other basic calculations (+,-,*,/,**)
We learned about variables. Variables store information in a program. Now, we are going to learn about changing how a program moves. What are some characteristics of a flow chart?
If statements [15 minutes]
An ‘if’ statement is also called a ‘conditional statement’
If a certain condition is met, something will happen
Indented regions determine what is part of an ‘if’ section
Consider the following code: x=4 y=9 if (x print “Hello, world!” What happened? Change ‘<’ to ‘>’; what happens? Add to the bottom of the program else: print “Goodbye, world!” Loops [15 minutes] Computers are really fast and really dumb We don’t want to program every single simple command that a computer should do Loops let us, well, loop a program a certain number of times automatically Type the following in: for count in range(20): print “Looped it!” Run the program What happened? Every time through a loop, the ‘count’ variable is equal to the number of times the loop has been run Change to: for count in range(20): print count Can you think of some way to add up all of the numbers 0-19? x=0 for count in range(20): x = x+count print x Can combine with if statements if (count < 5): print “count is less than 5” Functions (if enough time) [15 minutes] Functions are also called ‘subroutines’ Declare a function def functionName(): print “Hello!” print “Every time that I run this function…” print “ALL of these lines of code are run!” print “It would get really messy to do this manually.” What happens when this is run? (Nothing. Need to actually call the function.) Explain how you can repeatedly take a function to make a more complex function Inputs (if enough time) [10 minutes] As is, the programs we have worked with simply run the code that you give it, but don’t allow for any user interaction. print “Enter your text here” variableName = input() Simulations [25 minutes] Modify simulation [20 minutes] This is essentially a very basic particle simulation: physics aren’t perfect; discuss what that means Show basic structure of the simulation (What’s at top? Show how the variables are being used later in the program. What is the ‘def’ command for? Where is the main part of the program?) NOT SCARY! JUST LONG! Run the simulation Change a variable’s value on top Run again Did the program change like you expected it to? A well-written program with well-named variables is not difficult to understand How to teach a simulation [5 minutes] Simulations are so much more than an animation The computer is continually running mathematical and scientific formula calculations to run the simulation For instance, in the particle simulation, every step the computer uses the velocity to calculate the ball’s new position, changes the velocity due to gravity, and handles any collisions it might encounter In a population dynamics simulation (in biology, with wolves and rabbits), after each round the computer has to calculate (based off of some formula) what the population will be in the next round You don’t have to necessarily understand everything that went into the creation of a simulation that you are teaching from, but make sure that you explain to students that there are scientific and mathematical calculations which are being repeatedly run, in an attempt to accurately simulate the real world. No simulation is 100% accurate: we could theoretically simulate something down to the quantum level, but that’s ridiculously complex and slow. We only need a sim to be accurate enough for the purpose at hand.